AdMob App Open Ads : Android Studio Implementation
What are AdMob App Open Ads?
AdMob App Open Ads are a unique ad format designed to display full-screen advertisements during app loading times or app transitions, such as when launching the app or switching between app screens.
Unlike traditional interstitial ads that interrupt the user’s flow within the app, App Open Ads are displayed in a non-intrusive manner, enhancing the user experience and maintaining engagement.
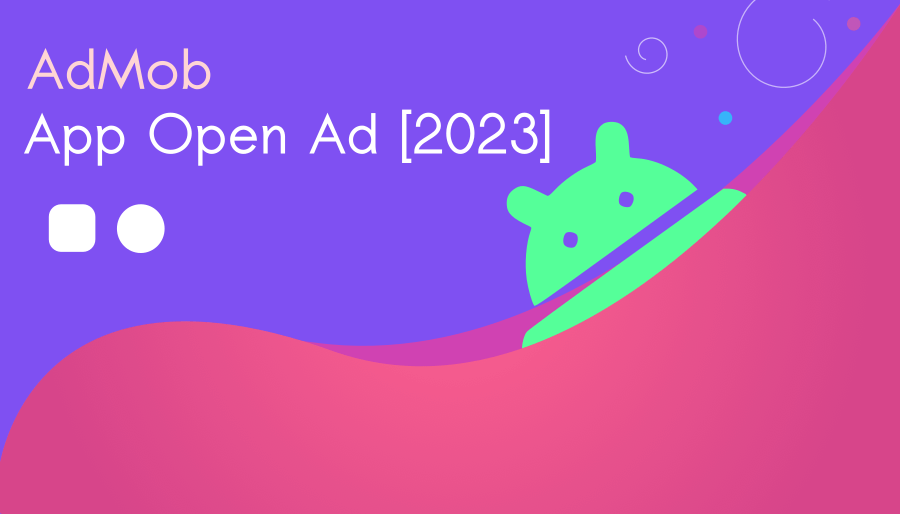
Key Benefits
Non-Intrusive User Experience
By displaying ads during natural app transitions, App Open Ads avoid interrupting the user’s flow, resulting in a seamless and non-disruptive experience. Users can continue using the app without feeling frustrated or annoyed by sudden ad interruptions.
Higher Revenue Potential
App Open Ads provide an excellent opportunity for developers and publishers to boost their revenue. By monetizing app loading times and transitions, which would otherwise remain unutilized, developers can significantly increase their ad inventory and maximize revenue potential.
AdMob App Open Ads Implementation Guide [AdMob Setup]
Step 1: Open AdMob and create an unit for App Open Ad.
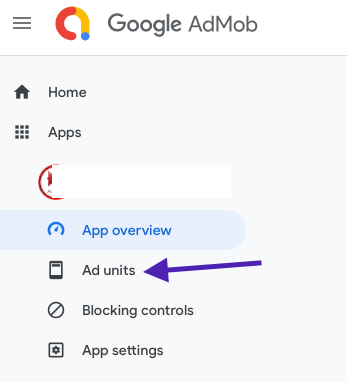
Please select the app in which you want to display the ads. Select Ad units from the menu.
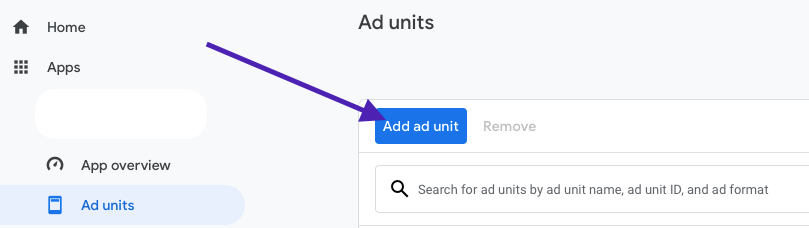
Click on “Add ad unit” button.
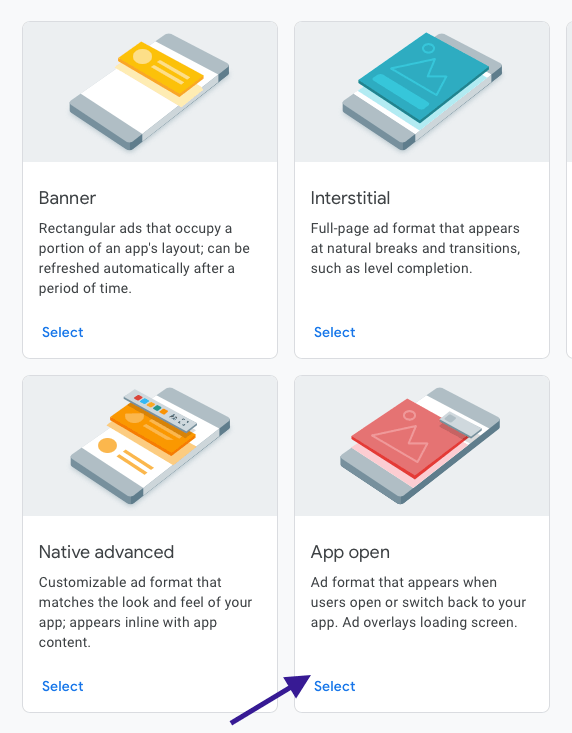
Next, you can choose ‘App open’ from the list of options.
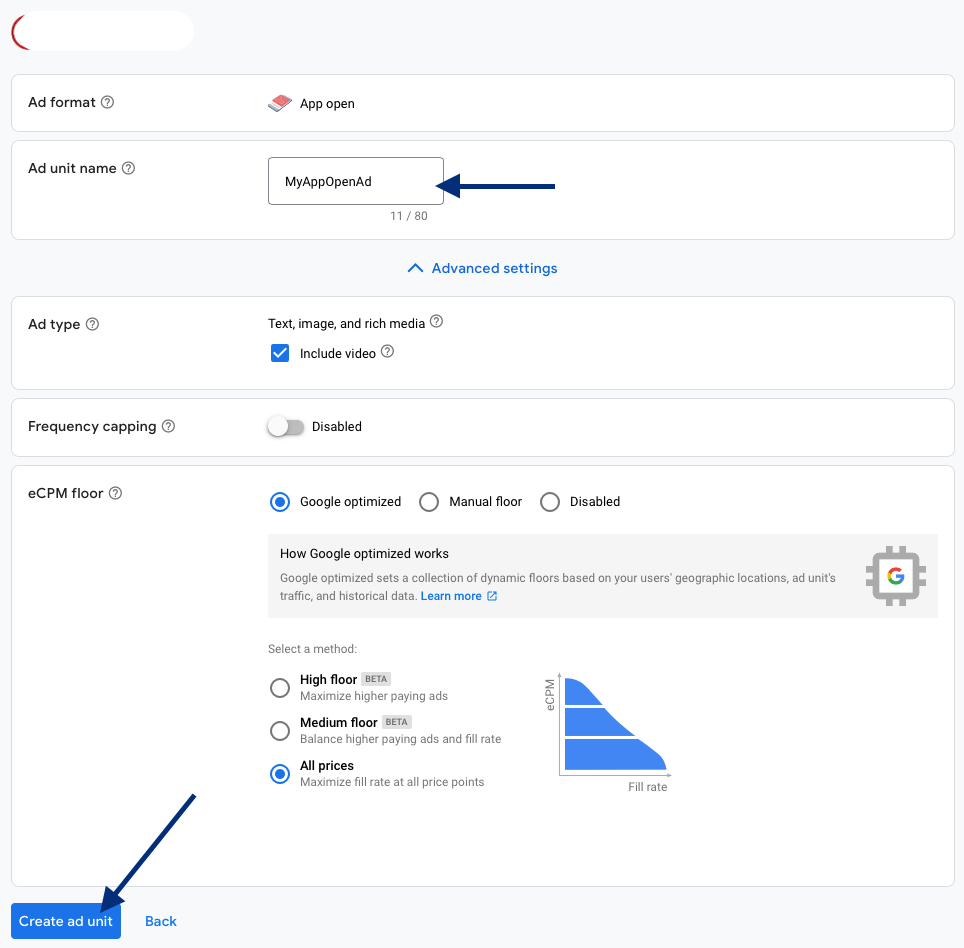
Let’s give our ad unit a name. It’s better to keep the name short.(Here MyAppOpenAd) Finally click on ‘Create ad unit’.
You can then view the App ID and App Open Ad Unit ID. Copy these IDs for future use. In this tutorial, We use test unit id for implementing app open ads. (Test ID: ca-app-pub-3940256099942544/3419835294)
App Open Ads Implementation [Android Studio Setup]
Create a new Android Project or use existing one.
Add the following dependencies to [build.gradle (:app)]
def lifecycle_version = "2.2.0"
implementation "androidx.lifecycle:lifecycle-extensions:$lifecycle_version"
implementation "androidx.lifecycle:lifecycle-runtime:$lifecycle_version"
annotationProcessor "androidx.lifecycle:lifecycle-compiler:$lifecycle_version"
Sync your project.
Let’s place the App ID within the <application></application> tags of the AndroidManifest.xml file.
<meta-data
android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="ca-app-pub-XXX"/>
Replace ‘ca-app-pub-XXX’ with your own App ID.
In Android Studio, let’s create a new class called MyApplication.java
MyApplication.java [Full Code]
package com.your.packge.name;
import android.app.Activity;
import android.app.Application;
import android.content.Context;
import android.os.Bundle;
import android.util.Log;
import androidx.annotation.NonNull;
import androidx.lifecycle.Lifecycle;
import androidx.lifecycle.LifecycleObserver;
import androidx.lifecycle.OnLifecycleEvent;
import androidx.lifecycle.ProcessLifecycleOwner;
import com.google.android.gms.ads.AdError;
import com.google.android.gms.ads.AdRequest;
import com.google.android.gms.ads.FullScreenContentCallback;
import com.google.android.gms.ads.LoadAdError;
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.appopen.AppOpenAd;
import com.google.android.gms.ads.initialization.InitializationStatus;
import com.google.android.gms.ads.initialization.OnInitializationCompleteListener;
import java.util.Date;
public class MyApplication extends Application implements Application.ActivityLifecycleCallbacks, LifecycleObserver {
private AppOpenAdManager appOpenAdManager;
private Activity currentActivity;
public long loadTime = 0;
@Override
public void onCreate() {
super.onCreate();
MobileAds.initialize(
this,
new OnInitializationCompleteListener() {
@Override
public void onInitializationComplete(InitializationStatus initializationStatus) {}
});
ProcessLifecycleOwner.get().getLifecycle().addObserver(this);
appOpenAdManager = new AppOpenAdManager(this);
}
/** LifecycleObserver method that shows the app open ad when the app moves to foreground. */
@OnLifecycleEvent(Lifecycle.Event.ON_START)
protected void onMoveToForeground() {
// Show the ad (if available) when the app moves to foreground.
appOpenAdManager.showAdIfAvailable(currentActivity);
}
/** Show the ad if one isn't already showing. */
/** Inner class that loads and shows app open ads. */
/** Interface definition for a callback to be invoked when an app open ad is complete. */
public interface OnShowAdCompleteListener {
void onShowAdComplete();
}
private class AppOpenAdManager {
private static final String LOG_TAG = "AppOpenAdManager";
private static final String AD_UNIT_ID = "ca-app-pub-3940256099942544/3419835294";
private AppOpenAd appOpenAd = null;
private boolean isLoadingAd = false;
private boolean isShowingAd = false;
/** Constructor. */
public AppOpenAdManager(MyApplication myApplication) {}
/** Request an ad. */
private void loadAd(Context context) {
// We will implement this below.
// Do not load ad if there is an unused ad or one is already loading.
if (isLoadingAd || isAdAvailable()) {
return;
}
isLoadingAd = true;
AdRequest request = new AdRequest.Builder().build();
AppOpenAd.load(
context, AD_UNIT_ID, request,
AppOpenAd.APP_OPEN_AD_ORIENTATION_PORTRAIT,
new AppOpenAd.AppOpenAdLoadCallback() {
@Override
public void onAdLoaded(AppOpenAd ad) {
// Called when an app open ad has loaded.
Log.d(LOG_TAG, "Ad was loaded.");
appOpenAd = ad;
isLoadingAd = false;
loadTime = (new Date()).getTime();
}
@Override
public void onAdFailedToLoad(LoadAdError loadAdError) {
// Called when an app open ad has failed to load.
Log.d(LOG_TAG, loadAdError.getMessage());
isLoadingAd = false;
}
});
}
/** Shows the ad if one isn't already showing. */
public void showAdIfAvailable(
@NonNull final Activity activity
/*@NonNull OnShowAdCompleteListener onShowAdCompleteListener */){
// If the app open ad is already showing, do not show the ad again.
if (isShowingAd) {
Log.d(LOG_TAG, "The app open ad is already showing.");
return;
}
// If the app open ad is not available yet, invoke the callback then load the ad.
if (!isAdAvailable()) {
Log.d(LOG_TAG, "The app open ad is not ready yet.");
//** onShowAdCompleteListener.onShowAdComplete();
loadAd(MyApplication.this);
return;
}
appOpenAd.setFullScreenContentCallback(
new FullScreenContentCallback() {
@Override
public void onAdDismissedFullScreenContent() {
// Called when fullscreen content is dismissed.
// Set the reference to null so isAdAvailable() returns false.
Log.d(LOG_TAG, "Ad dismissed fullscreen content.");
appOpenAd = null;
isShowingAd = false;
//** onShowAdCompleteListener.onShowAdComplete();
loadAd(activity);
}
@Override
public void onAdFailedToShowFullScreenContent(AdError adError) {
// Called when fullscreen content failed to show.
// Set the reference to null so isAdAvailable() returns false.
Log.d(LOG_TAG, adError.getMessage());
appOpenAd = null;
isShowingAd = false;
//** onShowAdCompleteListener.onShowAdComplete();
loadAd(activity);
}
@Override
public void onAdShowedFullScreenContent() {
// Called when fullscreen content is shown.
Log.d(LOG_TAG, "Ad showed fullscreen content.");
}
});
isShowingAd = true;
appOpenAd.show(activity);
}
/** Check if ad exists and can be shown. */
private boolean isAdAvailable() {
return appOpenAd != null && wasLoadTimeLessThanNHoursAgo(4);
}
}
private boolean wasLoadTimeLessThanNHoursAgo(long numHours) {
long dateDifference = (new Date()).getTime() - this.loadTime;
long numMilliSecondsPerHour = 3600000;
return (dateDifference < (numMilliSecondsPerHour * numHours));
}
/** ActivityLifecycleCallback methods. */
@Override
public void onActivityCreated(Activity activity, Bundle savedInstanceState) {}
@Override
public void onActivityStarted(Activity activity) {
// Updating the currentActivity only when an ad is not showing.
if (!appOpenAdManager.isShowingAd) {
currentActivity = activity;
}
}
@Override
public void onActivityResumed(Activity activity) {}
@Override
public void onActivityStopped(Activity activity) {}
@Override
public void onActivityPaused(Activity activity) {}
@Override
public void onActivitySaveInstanceState(Activity activity, Bundle bundle) {}
@Override
public void onActivityDestroyed(Activity activity) {}
}
Let’s modify the AndroidManifest.xml file as below. Add MyApplication as android:name.
<application
android:name=".MyApplication"
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name">
<!-- .... -->
</application>
Run your app. The ad will show when you switch back to your app screen.