Data Class in Kotlin : Copying, hashCode and toString
Let’s explore the data class in Kotlin : In this Kotlin tutorial, We’ll unfold Kotlin data classes and understand how they can simplify your codebase.
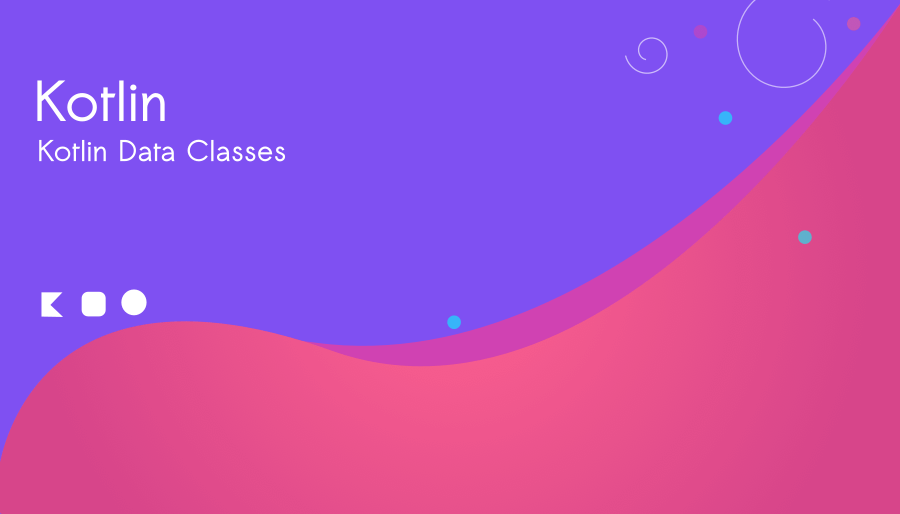
What are Kotlin Data Classes?
In Kotlin, data classes are a special type of class that is designed to hold data. They automatically generate useful methods such as toString(), equals(), hashCode(), and copy() based on the properties defined in the class. This eliminates the need for boilerplate code that developers often write to implement these methods.
How to Define a Data Class in Kotlin?
To define a data class in Kotlin, you simply use the data keyword before the class declaration.
data class Employee(val name:String, val id: Int)
In the above example, we have defined a data class called ‘Employee’ with two properties ‘name’ of type String an ‘id’ of type Int. One of the advantages of Kotlin is that it provides a feature called data classes, which automatically generates several methods for you, including toString(), equals(), hashCode(), and copy(). This saves you from writing repetitive boilerplate code.
toString()
Returns a string representation of the object, including all the property values.
val emp = Employee("Rocky", 898)
println(emp)
Output
Employee(name=Rocky, id=898)
Program
data class Employee(val name:String, val id: Int)
fun main()
{
val emp = Employee("Rocky", 898)
println(emp)
}
Let’s explicitly call the toString() method. Here’s the updated code snippet:
val emp = Employee("Rocky", 898)
println(emp.toString())
We explicitly call the toString() method on the emp object using the dot notation (emp.toString()). This will produce the same output as before, displaying the string representation of the empire object, including its property values.
Comparing Objects in Kotlin
Data classes are also useful when it comes to comparing objects. Instead of manually implementing the equal() and hashCode() methods, Kotlin generates them for you based on the properties defined in the class. This makes it easy to compare objects for equality without writing additional code.
val employee1 = Employee("Raju", 6768)
val employee2 = Employee("Raju", 6768)
val check = if (employee1 == employee2) "same person" else "unique details"
println(check)
Output
same person
Full source code:
Open Kotlin Playground and Write the following program
fun main()
{
val emp = Employee("Rocky", 898)
println(emp.toString()) // toString() is not necessary
// checking equality
val employee1 = Employee("Raju", 6768)
val employee2 = Employee("Raju", 6768)
val check = if (employee1 == employee2) "same person" else "unique details"
println(check)
}
Overview of the above program:
- We create an instance of the Employee class called employee1 with the name “Raju” and an ID of 6768.
- Similarly, we create another instance of the Employee class called employee2 with the same name “Raju” and the same ID of 6768.
- We then compare employee1 and employee2 using the == operator to check if they represent the same person.
- If employee1 and employee2 have the same property values (name and ID), the condition evaluates to true, and the string “same person” is assigned to the check variable.
- If employee1 and employee2 have different property values, the condition evaluates to false, and the string “unique details” is assigned to the check variable.
- Finally, we print the value of the check variable, which indicates whether the employee1 and employee2 objects represent the same person or have different details.
Read more about Conditional Expression in Kotlin.
Kotlin copy method()
Data classes also provide a convenient copy() method that allows you to create copies of objects with some properties modified:
val emps = Employee("Rahul",737)
val newEmp = emps.copy(id = 7844)
println(newEmp)
Output
Employee(name=Rahul, id=7844)
The copy() method copies all the properties of the original object while allowing you to override specific properties as needed.
Kotlin hashCode()
val e1 = Employee("Amidal",67)
val e2 = Employee("Amidal", 67)
println(e1.hashCode())
println(e2.hashCode())
Output
778820017
778820017
By calling the hashCode() method on each object, we can see that both e1 and e2 produce the same hash code value (778820017). The hashCode() method generated by Kotlin is based on the object’s property values. Since the property values are the same for both objects, they result in the same hash code.
Why do we need HashCodes?
The hashCode() method is useful when you need to store objects in hash-based data structures such as HashSet or HashMap. It ensures that objects with equal property values produce the same hash code, allowing efficient retrieval and comparison operations in these data structures.
Kotlin data classes are a powerful feature that simplifies the creation and manipulation of immutable data models. By eliminating the need for boilerplate code, they help developers write clean code.