Kotlin String Templates :Let’s include expressions in Strings
One of the standout features of Kotlin is its robust support for string templates, which enables developers to write concise and readable code while seamlessly interpolating values into strings. Let’s explore Kotlin string templates and how they can simplify your code.
What are Kotlin String Templates?
Kotlin string templates provide a convenient way to embed expressions and variables directly into strings, eliminating the need for string concatenation and making the code more readable. String templates use the syntax ${expression} to interpolate values dynamically.
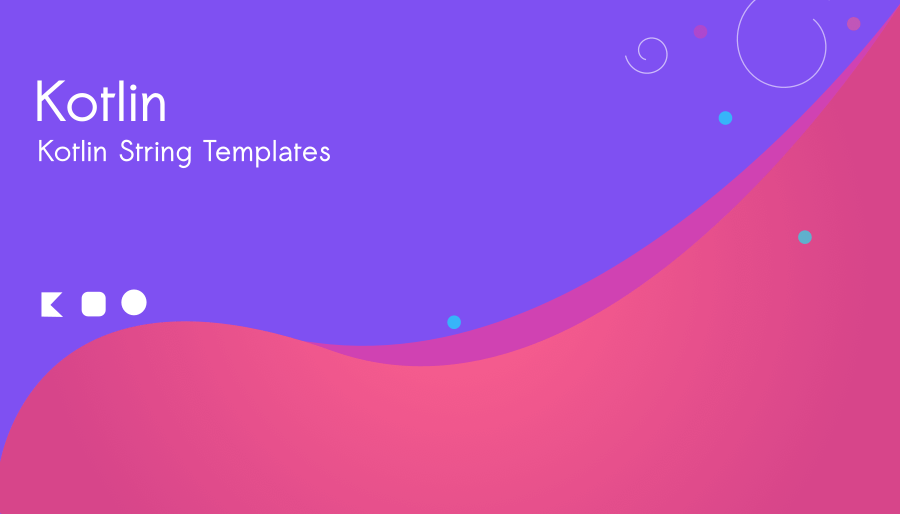
Let’s start with a basic example to illustrate how Kotlin string templates work
fun main() {
val name = "Nikky"
val age = 50
val message = "My name is $name and I am $age years old."
println(message)
}
Output
My name is Nikky and I am 50 years old.
We define two variables name and age. We then use string templates to interpolate these variables into the message string using the $ sign followed by the variable name.
Expression Evaluation in Kotlin
Kotlin string templates not only allow variable interpolation but also support arbitrary expressions within ${}. This enables us to perform calculations, invoke methods, and access properties while building the string.
fun main() {
val radius = 5
val circumference = "The circumference of a circle with radius $radius is ${2 * Math.PI * radius}."
println(circumference)
}
We compute the circumference of a circle using the formula 2 * π * radius and interpolate the result directly into the string.
Open Kotlin Play Ground and write the following program.
fun main()
{
val ask = "What's on my Calendar"
println("Hey Google: $ask")
println("Hm..please wait.. ${ask.uppercase()}")
val status = false
println("No response: ${if (status) "good" else "error"}")
}
Hey Google: What's on my Calendar
Hm..please wait.. WHAT'S ON MY CALENDAR
No response: error
The variable ask is assigned the string value “What’s on my Calendar”. This variable will be used later in string interpolations.
The first println statement uses string interpolation to concatenate the value of ask with a prefix message “Hey Google: “. The result is printed to the console as “Hey Google: What’s on my Calendar”.
The second println statement demonstrates string interpolation with a function call. Here, the ask variable is accessed and the uppercase() function is applied to it using the ${} syntax.
The third println statement uses string interpolation combined with a conditional expression. It checks the value of status using the if statement. If status is true, the interpolated value will be “good”, and if status is false, the interpolated value will be “error”. The result is printed to the console as “No response: error” since status is false.
The templates offer a powerful and concise way to build dynamic strings by interpolating values and expressions.