Kotlin Null Safety : How to Eliminate NPEs ?
Null pointer exceptions (NPEs) have long plagued developers, causing unexpected crashes and bugs in software applications. Kotlin, a modern programming language developed by JetBrains, introduced a groundbreaking feature called “null safety.”
Null Pointer Exceptions (NPEs)
Null references have been a notorious source of bugs in programming languages like Java. When a variable is declared but not assigned a value, or when a method returns null, accessing that variable or invoking methods on it can lead to NPEs.
These runtime errors occur when a program tries to access or manipulate an object that doesn’t exist, resulting in unexpected crashes and erratic behaviour.
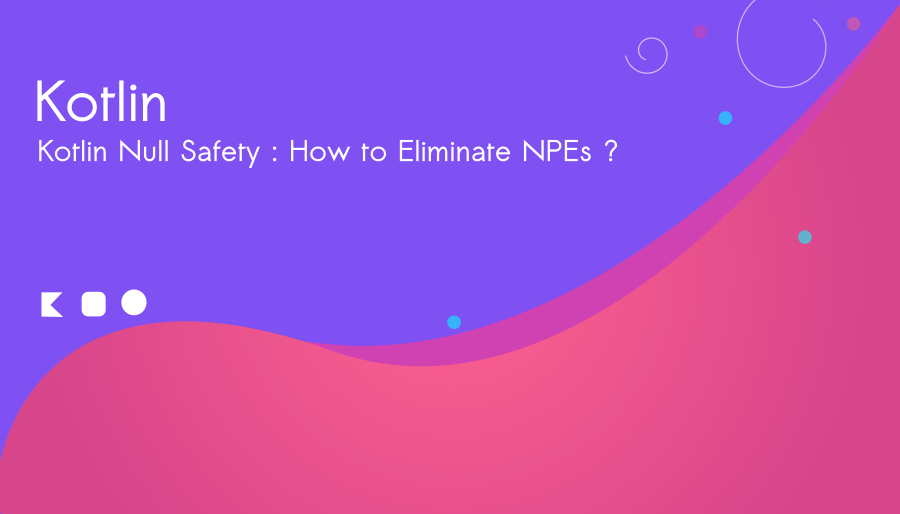
Introducing Kotlin Null Safety
The null safety addresses the problem of NPEs by building the concept of nullability directly into the type system. It introduces two types: nullable and non-nullable types.
In Kotlin, a type is nullable if it can hold a null value, and non-nullable if it cannot. The compiler enforces strict nullability rules, requiring developers to handle null cases explicitly.
Declaring Nullable Types
To declare a nullable type in Kotlin, you simply append a question mark (?) to the type declaration. For example, String? declares a nullable string type.
By explicitly indicating nullability, Kotlin forces developers to handle the possibility of null values, promoting safer programming practices.
Consider the following example
fun main() {
var name:String = "Nikky"
name = "Aswin"
println(name) // prints Aswin
}
The above program will print the name ‘Aswin’ on playground or IntelliJ IDEA console.
Let’s make some changes to the above program.
fun main() {
var name:String = "Nikky"
name = null
println(name)
}
name = null
You will get an error message as below:
Null can not be a value of a non-null type String
Why ? Why ?
In Kotlin, variables that are not explicitly declared as nullable cannot hold null values. When you declare the variable ‘name’ as var name: String = “Nikky”, you are explicitly stating that name can only hold non-null String values.
However, in the next line name = null, you are assigning a null value to the name variable, which contradicts its non-null type declaration. This violates Kotlin’s null safety rules, and the compiler will flag it as an error.
To fix this issue
fun main() {
var name: String? = "Nikky"
name = null
println(name)
}
By adding a question mark (?) after the type declaration, var name: String? makes name a nullable String. Now, assigning null to name is valid.
Kotlin Non-Null Assertion Operator
fun main() {
var name: String? = "Nikky"
if (name != null) {
println(name!!)
}
}
Output
Nikky
We need to ensure that the variable is not null. We use an if statement to check if name is not null before applying the non-null assertion operator (!!). If name is null, the code inside the if block will not execute, and therefore, the non-null assertion operator won’t be triggered. This prevents the NullPointerException.