Cracking the Kotlin Code: A Beginner’s Kotlin Tutorial
What is Kotlin?
Kotlin is a modern programming language that runs on the Java Virtual Machine (JVM) and can also be compiled to JavaScript or native code. It was developed by JetBrains, the creators of popular development tools like IntelliJ IDEA.
The Kotlin programming language can be compiled using the Kotlin compiler(kotlinc). The Kotlin compiler is a command-line tool that takes Kotlin source code as input and produces bytecode that can be executed on the JVM, JavaScript code or native code, depending on the target platform.
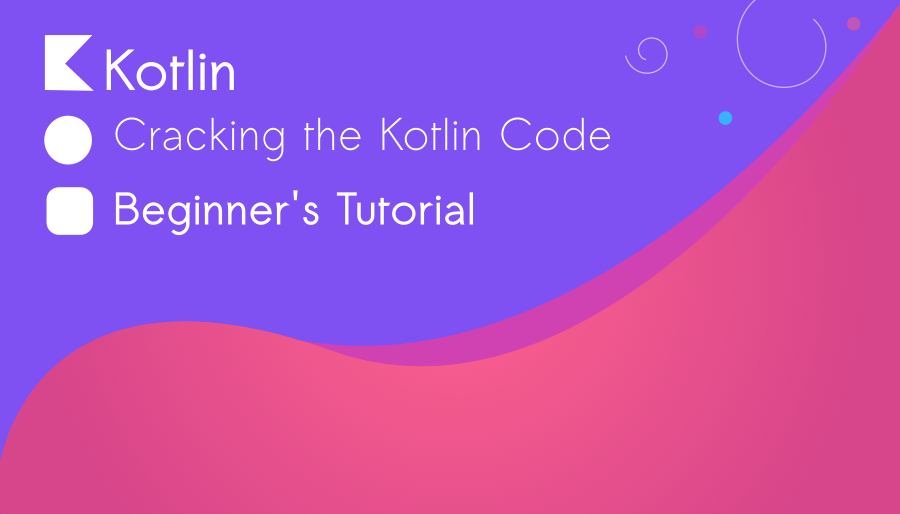
When you install the Kotlin programming language, the Kotlin compiler is included as part of the Kotlin distribution. We can use the compiler directly from the command line or integrate it into the your development environment or build system.
Kotlin is also supported by various integrated development environments (IDEs) such as IntelliJ IDEA, Android Studio, and Eclipse.
These IDEs provide built-in support for Kotlin, including features like code completion, syntax highlighting, and error checking, making it easier to develop Kotlin applications.
Why Kotlin ?
Kotlin is used for various purposes and different domains. Here are some of the reasons why Kotlin is commonly used:
- Android App Development :
Kotlin has gained significant popularity in the Android development community. In 2017, Google announced Kotlin as an officially supported language for Android Development, alongside Java.
Many developers prefer Kotlin for Android Development due to its concise syntax, enhanced productivity, null safety features, and seamless interoperability with existing Java code and libraries.
2. Server-Side Development:
Kotlin is not limited to Android app development. It can also be used for server-side development. Kotlin provides frameworks and libraries such as Ktor, and Spring Boot, which make it easier to build web applications, APIs, and backend services.
3. Desktop Applications:
Kotlin can be used to develop desktop applications for platforms like Windows, macOS, and Linux.
The Kotlin programming language, along with frameworks like TornadoFX and JavaFX, provides the necessary tools and libraries to create efficient desktop applications with a modern and user-friendly interface.
4. Cross-Platform Development:
Kotlin can be used for cross platform development, allowing developers to write code once and deploy it on multiple platforms. With Kotlin Multiplatform Mobile (KMM), developers can share business logic and code across Android and iOS platforms.
5. Gradual Adoption:
Kotlin’s interoperability with Java allows for gradual adoption in existing Java projects. Developers can introduce Kotlin code into their Java projects and leverage Kotlin’s features and benefits without the need for a complete rewrite.
Let’s write our first Kotlin program
fun main()
{
println("Hello world!")
}
An entry point of a Kotlin application is the main function. fun main { … } This line declares a function named main. In Kotlin the main
function is the entry point of the program, similar to languages like Java and C++. It serves as the starting point for program execution.
println(“Hello world!”)
The line prints the string `Hello world!` to the console (output area). println() is a built-in function used for printing text to the standard output(console). When you run this program, it will simply print the message “Hello world!” tothe console.
How to execute Kotlin program?
Way 1 : Using Command Line Tools | run kotlin command line
Step 1: Create a Kotlin source file, typically with a .kt extension. For example, hi.kt.
Step 2: Write the above code in this file (hi.kt)
Step 3: Compile the Kotlin code using the Kotlin compiler(kotlinc) by running the command:
kotlinc hello.kt -include-runtime -d hi.jar
This will generate a JAR file named `hi.jar`, which includes the compiled byte code of the Kotlin program along with Kotlin runtime library.
Finally run the program using the command:
java -jar hi.jar
This will execute the compiled program, and you will see the output ‘Hello world!’ Printed to the console.
Way 2 : Using IntelliJ IDEA (Easy method)
Step 1: Download the latest version of IntelliJ IDEA software and install on your computer.
Click on New Project, Give a name for your project. Choose Kotlin from Language options. The build system will be IntelliJ. (Make sure that you have downloaded and installed Java on your computer)
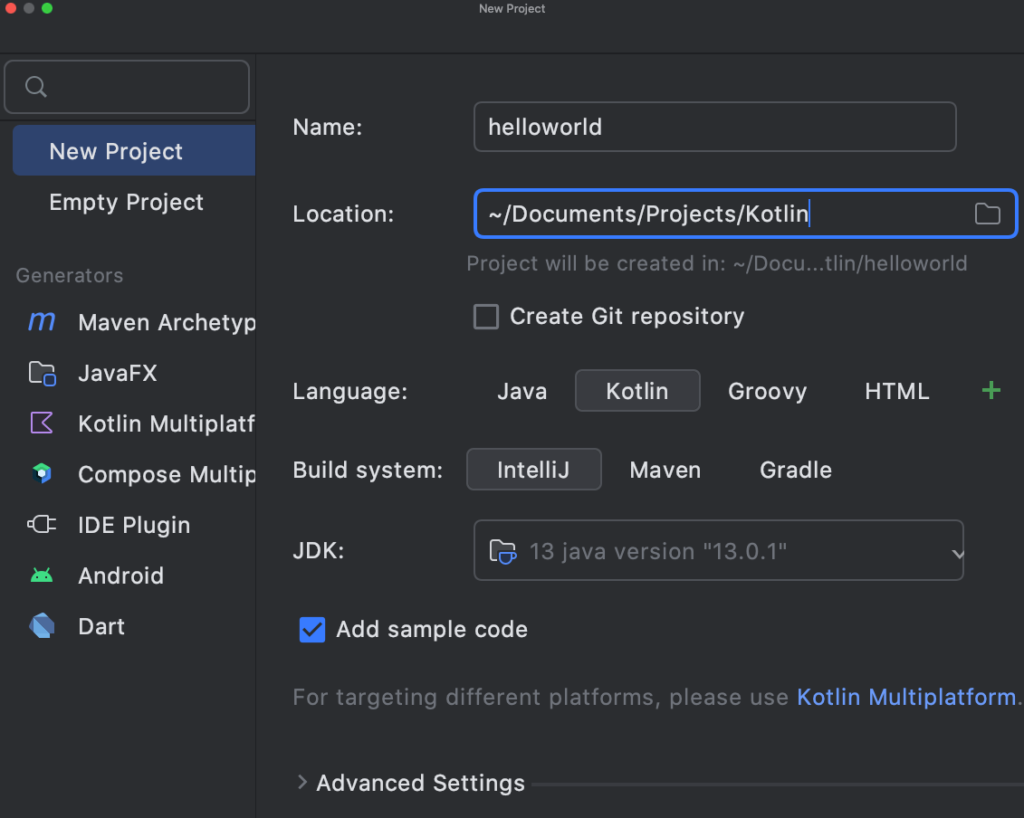
Write your code and run it.
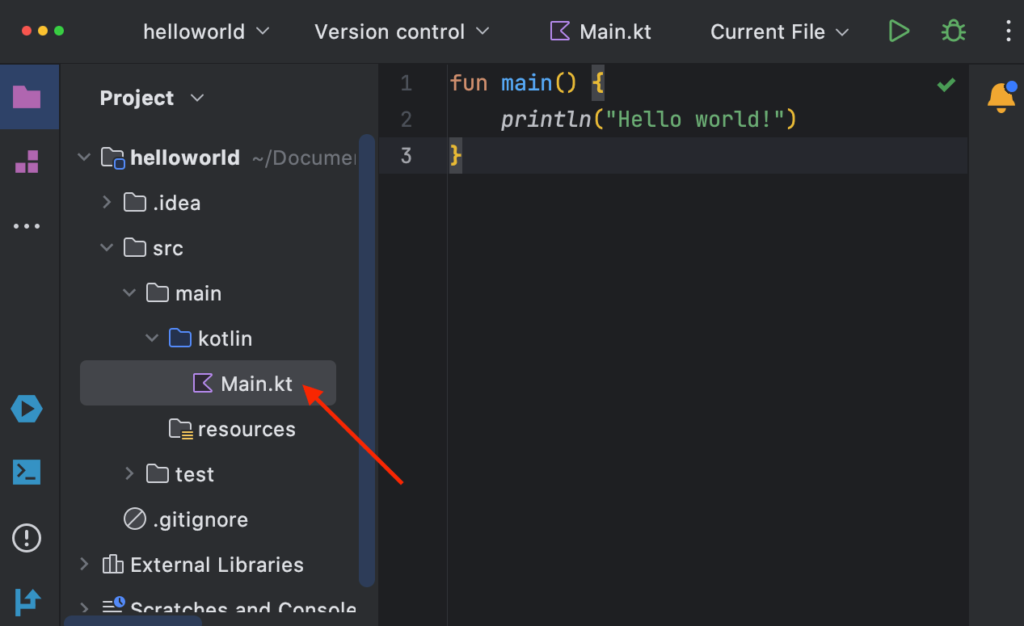
Your main source file will be Main.kt You can easily locate this file from your Project.
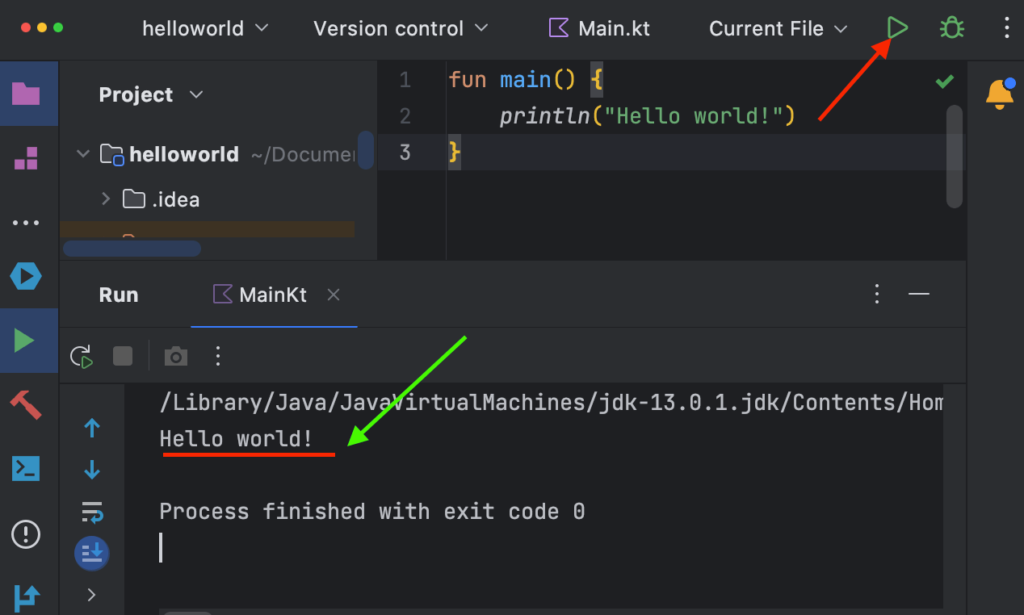
Click the play button (run button) to execute your Hello world Kotlin program.
Way 3 : Using Kotlin playground (Online)
This an incredible online tool for executing Kotlin programs. Just open the https://play.kotlinlang.org/ and type your code.
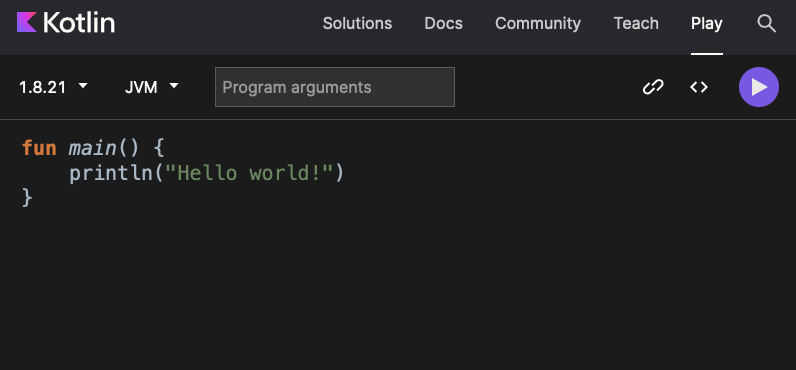
In this beginner’s Kotlin tutorial, we have learned about the usage of Kotlin and how it works, as well as how to run Kotlin programs using various methods