Kotlin Scope Functions : Guide on When to Use Them
Kotlin scope functions are powerful features that make your code more concise and readable by creating temporary scopes. In this tutorial, we’ll explore the five main scope functions—let, run, with, apply, and also—and provide clear guidance on when to use each one. Whether you’re a beginner or experienced Kotlin developer, understanding these functions will significantly improve your code quality.
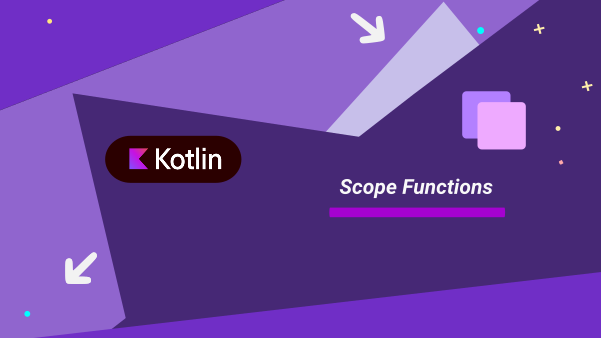
Understanding Kotlin Scope Functions
Kotlin scope functions allow you to execute a block of code within the context of an object. Each function serves a specific purpose, with differences in:
- How the object is referenced inside the scope (this vs. it)
- What value is returned (object itself vs. lambda result)
Let’s dive into each function and learn when to use them effectively.
The let Function: When to Use
The let function is ideal when you want to:
- Perform operations on non-null objects
- Use the result of a call chain
- Introduce a local variable with a limited scope
Example of let:
kotlin// Without let
val name: String? = "Kotlin"
if (name != null) {
println("Length of name is ${name.length}")
}
// With let
name?.let {
println("Length of name is ${it.length}")
}
- For null safety operations with ?.let
- When you need the lambda result rather than the object itself
- When you prefer using it instead of this for object reference
The run Function: When to Use
Use run when you want to:
- Execute a block of code with object member functions and properties
- Return a computed value from the lambda
- Group multiple operations on an object
val user = User("Nikin", "S", 25)
val formattedUser = user.run {
"$firstName $lastName, Age: $age"
}
- When you need to invoke multiple operations on an object
- When you want to use object members without its name qualifier
- When you need to return a result from the block
The with Function: When to Use
Choose with when you want to:
- Group operations on an object
- Use object members without repeating the object name
- Execute a block where the object is not nullable
val configuration = Configuration()
with(configuration) {
host = "localhost"
port = 8080
isSecure = true
}
- When working with non-null objects
- When you want to avoid repeating the object name in multiple operations
- When you’re not planning to chain further operations
The apply Function: When to Use
The apply function is perfect when you want to:
- Configure an object
- Set up object properties in a builder-style
- Return the object itself for chaining
Example of apply:
val textView = TextView(context).apply {
text = "Hello World"
textSize = 16f
setTextColor(Color.BLACK)
}
When to use apply:
- When configuring/initializing objects
- For builder-pattern implementations
- When you need to return the object itself after configuration
The also Function: When to Use
Use also when you want to:
- Perform additional operations on an object within a chain
- Add debugging or logging to a chain
- Verify object state within a chain
Example of also:
val numbers = mutableListOf(1, 2, 3)
numbers.also { println("The list elements: $it") }
.add(4)
- For side effects within a call chain (like logging)
- When you need to reference the object as it instead of this
- When you want to keep a clear separation between operations
Comparison and Selection Guide
To help you choose the right scope function, here’s a quick reference table:
Function | Object Reference | Return Value | Main Use Case |
---|---|---|---|
let | it | Lambda result | Null safety operations |
run | this | Lambda result | Operate and compute result |
with | this | Lambda result | Group multiple operations |
apply | this | Object itself | Object configuration |
also | it | Object itself | Additional actions |
Best Practices for Using Kotlin Scope Functions
- Choose for readability: Select the function that makes your code most readable
- Avoid nesting: Limit nesting of scope functions to maintain clarity
- Be consistent: Follow a consistent pattern in your codebase
- Consider return values: Choose functions based on whether you need the object or lambda result
- Mind the context: Use this (apply/run/with) when working with object members, it (let/also) when treating the object as a whole
Common Patterns and Use Cases
Builder Pattern with apply
data class User(var name: String = "", var age: Int = 0)
val user = User().apply {
name = "John"
age = 30
}
Safe Operations with let
val nullableValue: String? = "Hello"
nullableValue?.let {
// Only executes if nullableValue is not null
println("Value is: $it")
}
Chaining with also for Debugging
return loadData()
.process()
.also { Log.d("ProcessedData", "Size: ${it.size}") }
.display()
Conclusion
Kotlin scope functions are powerful tools that can make your code more concise, readable, and expressive. By understanding the unique characteristics and appropriate use cases for let, run, with, apply, and also, you can write cleaner and more maintainable Kotlin code.
Remember that the best choice depends on your specific situation, coding style preferences, and team conventions. Experiment with these functions in your projects to discover which patterns work best for your needs.
FAQ about Kotlin Scope Functions
Can I use scope functions with nullable objects?
Yes, particularly let with the safe call operator (?.let) for null safety operations.
What’s the performance impact of using scope functions?
Minimal to none. Kotlin scope functions are inline functions, meaning no additional overhead.
Should I always use scope functions?
No, use them when they improve readability. For simple operations, traditional approaches might be clearer.
Can scope functions be nested?
Yes, but limit nesting to maintain code readability. Excessive nesting can make code harder to understand.
What’s the difference between run and with?
run is an extension function called on an object, while with takes an object as a parameter. Use run when chaining and with for grouping operations.
Happy Coding!