Flutter AdMob Interstitial Android Studio Tutorial
Making money from mobile apps has become very important in app development, especially for small developers and new companies. There are millions of apps competing for users’ attention, so finding ways to earn money while keeping users happy is crucial. One good way to do this is by adding AdMob Interstitial ads to your Flutter app. In this flutter tutorial, we will explore how to integrate Flutter AdMob Interstitial ad in your app using Android Studio flamingo.
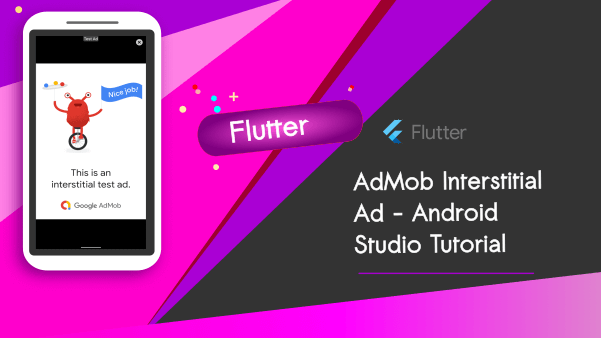
Why Choose Flutter AdMob Interstitial Ads?
Interstitial ads generally offer higher eCPMs (effective cost per thousand impressions) compared to other ad formats. Due to their full-screen nature, advertisers are willing to pay a premium for the increased visibility and engagement they receive.
Flutter, as a cross-platform framework, provides a seamless way to integrate AdMob Interstitial ads into both Android and iOS apps using a single codebase. This eliminates the need to develop separate implementations for each platform, saving time and effort.
Developers have control over when and where interstitial ads are displayed, ensuring they don’t interrupt critical user interactions. By strategically placing these ads during natural pauses, the user experience remains unaffected, leading to higher user retention.
Let’s create a new project:
Create a new Flutter Project in Android Studio.
Add the following Google Mobile Ads dependency in the pubspec.yaml file (created flutter project)
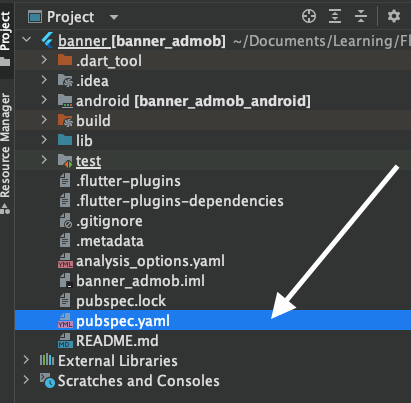
Add the following:
google_mobile_ads: ^1.2.0
To enable AdMob ads display in your Flutter application, you should integrate the dependency mentioned earlier. This incorporation will allow you to seamlessly showcase AdMob ads within your app.
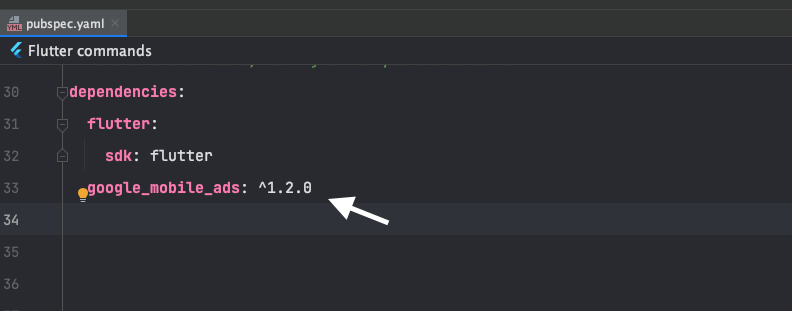
Finally hit on Pub get
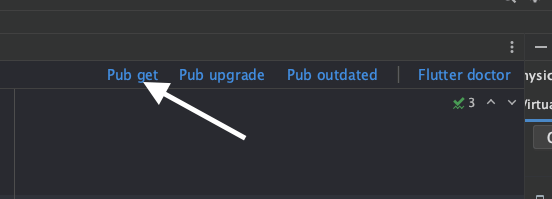
To access the “lib” folder of your project, navigate through the project directory. In case you’re using Android Studio, it usually generates a sample project automatically.
Once you locate the “lib” folder, open the “main.dart” file within it. This file contains the default code that Android Studio generates. Your next step is to remove all the default code to start with a clean slate for your Flutter application.
Rename the main.dart file to home.dart
Right click on ‘main.dart’ > Refactor > Rename : ‘home.dart’
Let’s create another file named ‘main_content.dart’
Currently, we have two files in place. However, we require an additional file to store our AdMob Interstitial Ad Unit ID. (AdMob Helper file)
Create an AdMob Helper file
admob_helper.dart :
import 'dart:io';
class AdMobHelper {
static String get interstitialAdUnit {
if (Platform.isAndroid) {
return "ca-app-pub-3940256099942544/1033173712";
} else if (Platform.isIOS) {
return "ca-app-pub-3940256099942544/4411468910";
} else {
throw UnsupportedError("Unsupported platform");
}
}
}
In your application, instead of utilizing the test unit IDs for AdMob, you have the option to use the production-ready AdMob Interstitial Unit ID from AdMob. However, if you intend to implement the Flutter AdMob banner, it is recommended to use the provided test IDs for testing purposes.
home.dart
Prior to loading ads, it is essential to initialize the Google Mobile Ads SDK.
Future<InitializationStatus> _initGoogleMobileAds()
{
return MobileAds.instance.initialize();
}
home.dart (complete code)
import 'package:flutter/material.dart';
import 'package:google_mobile_ads/google_mobile_ads.dart';
import 'package:banner_admob/main_content.dart';
void main() {
runApp(
MaterialApp(
title: 'Interstitial Flutter Demo',
initialRoute: '/',
routes: {
'/' : (context) => const MyApp(),
'/main_content' : (context) => const MainContent(),
},
)
);
}
class MyApp extends StatelessWidget{
const MyApp({super.key});
Future<InitializationStatus> _initGoogleMobileAds()
{
return MobileAds.instance.initialize();
}
@override
Widget build(BuildContext context) {
return Scaffold(appBar: AppBar(title: const Text('AdMob Demo'),),
body: Center(
child: Column(
children: [
const Text("Interstitial Ad Flutter"),
ElevatedButton(onPressed: (){
Navigator.pushNamed(context, '/main_content');
}, child: const Text("Access Content"))
],
),
),
);
}
}
main_content.dart
Add an interstitial ad:
InterstitialAd? _interstitialAd;
How to load the ad?
InterstitialAd.load(
adUnitId: AdMobHelper.interstitialAdUnit,
request: const AdRequest(),
//....
);
Show the ad:
if(_interstitialAd != null){
_interstitialAd?.show();
} else {}
main_content.dart (Complete code)
import 'package:flutter/material.dart';
import 'package:banner_admob/admob_helper.dart';
import 'package:google_mobile_ads/google_mobile_ads.dart';
void main()
{
runApp(
const MaterialApp(
title: 'AdMob Banner Ad Demo',
initialRoute: '/',
)
);
}
class MainContent extends StatefulWidget {
const MainContent({super.key});
@override
_MainContentState createState() => _MainContentState();
}
class _MainContentState extends State<MainContent> {
InterstitialAd? _interstitialAd;
@override
void initState() {
super.initState();
}
void _prepareInterstitialAd(){
InterstitialAd.load(
adUnitId: AdMobHelper.interstitialAdUnit,
request: const AdRequest(),
adLoadCallback: InterstitialAdLoadCallback(
onAdLoaded: (currentAd){
currentAd.fullScreenContentCallback = FullScreenContentCallback(
onAdDismissedFullScreenContent: (currentAd){
}
);
setState(() {
_interstitialAd = currentAd;
});
},
onAdFailedToLoad: (error) {
print("Failed to load : Flutter AdMob Interstitial Ad");
}),
);
}
@override
void dispose() {
_interstitialAd?.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(appBar: AppBar(title: const Text('AdMob Demo'),),
body: Center(
child: Column(
children: [
const Text("AdMob Interstitial Ad Demo App"),
ElevatedButton(onPressed: (){
if(_interstitialAd == null)
{
_prepareInterstitialAd();
}
}, child: const Text('On New Level')),
ElevatedButton(onPressed: (){
if(_interstitialAd != null){
_interstitialAd?.show();
} else {}
}, child: const Text("Main Content"))
],
),
),
);
}
}
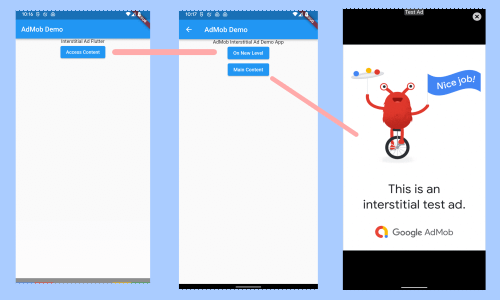
Integrating AdMob Interstitial ads into your Flutter app offers a lucrative pathway to monetize your hard work while ensuring a positive user experience.