Top 10 Essential Flutter Widgets for Newbie Developers
As a beginner in Flutter development, it is overwhelming to navigate the vast library of available widgets. However, there are a few essential flutter widgets that make life easier for newbie developers. These include basic layout elements like Container, AppBar and interactive components like RaisedButton.
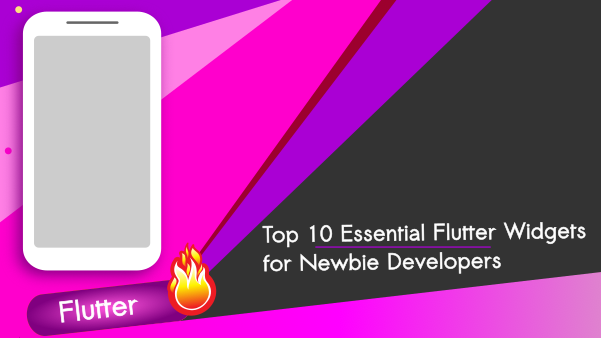
Simple Examples of Essential Flutter Widgets
1. Container Widget
A box that can contain other widgets. It has many options for customizing its appearance, such as background color and padding.
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Container(
width: 200,
height: 200,
color: Colors.blue,
child: Text(
'Hello World!',
style: TextStyle(fontSize: 24, color: Colors.white),
),
),
),
);
}
}
This example creates a blue Container that is 200 pixels wide and 200 pixels tall, and contains a white Text widget that says “Hello World!” The Container is centered on the screen using the Center widget.
2. RaisedButton (Button)
A clickable button with customisable text and appearance.
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyButton(),
),
);
}
class MyButton extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: RaisedButton(
onPressed: () {
print('Hello Jack');
},
child: Text('Click Me!'),
),
),
);
}
}
This will create a simple button that, when pressed, will print “Hello Jack” to the console.
You can customise the appearance and behaviour of the button by setting various properties on the RaisedButton widget.
3. ListView
A scrollable list of widgets. It can display a large number of items efficiently, with the ability to reuse widgets that are no longer visible.
Here is a simple example of how to create a ListView in Flutter:
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyListView(),
),
);
}
class MyListView extends StatelessWidget {
final List<String> items;
MyListView({Key key, this.items}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text('${items[index]}'),
);
},
),
);
}
}
This will create a scrolling list of items, where each item is represented by a ListTile widget.
4. Text Widget
A widget for displaying text. It has options for styling the text, such as color, font size, and font weight.
How to use the Text widget in Flutter?
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyText(),
),
);
}
class MyText extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text(
'Hello World',
style: TextStyle(
fontSize: 28,
color: Colors.red,
),
),
),
);
}
}
This will display the text “Hello World” in red and with a font size of 28.
We can customise the appearance and behaviour of the Text widget by setting various properties, such as the style, textAlign, and textDirection.
5. Scaffold
A widget that provides a consistent visual structure for an app, including an app bar, a drawer, and a bottom navigation bar.
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyScaffold(),
),
);
}
class MyScaffold extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('My App'),
),
body: Center(
child: Text('Hello World'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
print('Button pressed!');
},
child: Icon(Icons.add),
),
);
}
}
We can customise the appearance and behaviour of the Scaffold and its children by setting various properties, such as the appBar, body, floatingActionButton, and bottomNavigationBar.
6. Expanded
A widget that expands to fill available space. It is often used in combination with Row and Column to create a flexible layout.
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyExpanded(),
),
);
}
class MyExpanded extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
children: [
Container(
height: 100,
color: Colors.red,
),
Expanded(
child: Container(
color: Colors.green,
),
),
Container(
height: 100,
color: Colors.blue,
),
],
),
);
}
}
This will create a layout with three containers stacked vertically, with the middle container taking up the remaining free space.
7. Form
A container for form fields, such as TextFormField and DropdownButtonFormField. It provides built-in validation and saves form data when the app goes to the background.
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyForm(),
),
);
}
class MyForm extends StatefulWidget {
@override
_MyFormState createState() => _MyFormState();
}
class _MyFormState extends State<MyForm> {
final _formKey = GlobalKey<FormState>();
String _name;
int _age;
@override
Widget build(BuildContext context) {
return Scaffold(
body: Form(
key: _formKey,
child: Column(
children: [
TextFormField(
decoration: InputDecoration(labelText: 'Name'),
validator: (value) {
if (value.isEmpty) {
return 'Please enter your name';
}
return null;
},
onSaved: (value) => _name = value,
),
TextFormField(
decoration: InputDecoration(labelText: 'Age'),
validator: (value) {
if (value.isEmpty) {
return 'Please enter your age';
}
return null;
},
onSaved: (value) => _age = int.parse(value),
),
RaisedButton(
onPressed: () {
if (_formKey.currentState.validate()) {
_formKey.currentState.save();
print('Name: $_name, Age: $_age');
}
},
child: Text('Submit'),
),
],
),
),
);
}
}
8. Image Widget
Image: A widget for displaying an image. It can display images from assets, network, or file system.
Here is an example of how to display an image in Flutter
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyImage(),
),
);
}
class MyImage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Image.asset('assets/images/simple_pic.png'),
),
);
}
}
This will display an image located in the assets/images directory of your project.
You can also display an image from the network by using the Image.network constructor
Image.network('https://cdn.pixabay.com/photo/2022/12/21/07/53/tooth-7669374_960_720.png')
9. Card Widget
A material design card that can contain other widgets, such as text, images, and buttons.
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyCard(),
),
);
}
class MyCard extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Card(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
const ListTile(
leading: Icon(Icons.album),
title: Text('Epic Malayalam Stories '),
subtitle: Text('Podcast By Nikin'),
),
ButtonBar(
children: [
FlatButton(
child: const Text('PLAY'),
onPressed: () {},
),
FlatButton(
child: const Text('MORE'),
onPressed: () {},
),
],
),
],
),
),
),
);
}
}
10. AppBar
A bar that is displayed at the top of the screen, usually containing a title, actions, and leading widget (such as a hamburger menu).
a simple example of an AppBar in Flutter
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
home: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('My App'),
),
body: Center(
child: Text('Hello World'),
),
);
}
}
You can customize the appearance of the AppBar by passing various arguments to the AppBar constructor.