Inheritance in Kotlin: Define and Overriding Superclass
In this Kotlin tutorial, we’ll delve into the world of inheritance, exploring its benefits and learning how to harness its potential in crafting clean and effective code.
Inheritance lets a new class, called a subclass or derived class, inherit properties and behaviors from an existing class known as a superclass or base class.
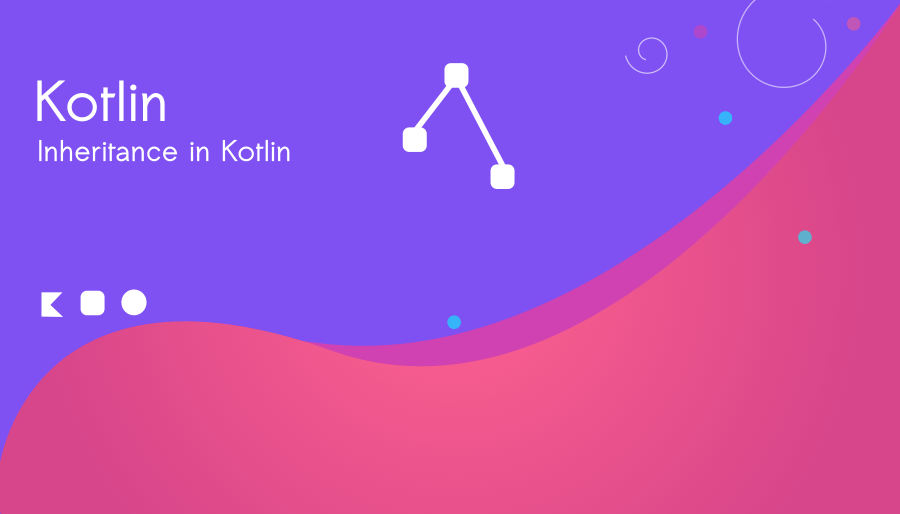
Inheritance in Koltin : Defining a Subclass
In Kotlin, the process of creating a subclass involves using the colon “:” followed by the name of the superclass in the class declaration.
Kotlin declares classes as final by default, which prevents their inheritance by other classes.
However, if you desire to allow class inheritance, you can achieve this by adding the “open” modifier to the class declaration.
This “open” modifier explicitly permits other classes to inherit from it, making it extendable and providing the ability to create subclass relationships.
open class Vegetable {
//Properties and methods of the Vegetable class
}
class Fruits: Vegetable() {
//Properties and methods specific to the Fruits class
}
Here, we have a Vegetable class as the superclass, and the Fruits class extends it. When we use the “open” keyword before declaring the Vegetable class, it explicitly grants permission for other classes to inherit from it.
When you want a class to inherit from a superclass, you can do so by specifying the superclass name after the class name, like this: class ClassName : SuperclassName()
. The empty parentheses ()
signify that the superclass’s default constructor is being invoked. This way, the subclass can access and build upon the functionalities provided by the superclass.
Open Kotlin play ground and write the following code:
package inheritance
open class Vegetable {
open fun sayHelloVeg() : String
{
return "Hello Vegetables"
}
}
class Fruits: Vegetable() {
fun sayHelloAll()
{
println("Hello Fruits " + sayHelloVeg())
}
}
fun main()
{
val obj = Fruits()
println(obj.sayHelloVeg());
obj.sayHelloAll()
}
The open keyword allows other classes to inherit from this class. It contains a single function sayHelloVeg(), which returns the string “Hello Vegetables”.
Inside the Fruits class, there’s a function sayHelloAll(), which prints the string “Hello Fruits” concatenated with the result of the sayHelloVeg() function inherited from the Vegetable class.
In the main()
function:
An instance of the Fruits class is created and assigned to the variable obj
.
Output:
Hello Vegetables
Hello Fruits Hello Vegetables
Inheritance in Kotlin: Overriding Superclass
Inheritance isn’t solely about inheriting properties; it also allows us to override methods and properties from the superclass in the subclass. To override a function in the subclass, use the override keyword.
package inheritance
fun main()
{
val ins = PaperDraw()
ins.draw()
val superIns = Drawing()
superIns.draw()
}
open class Drawing()
{
open fun draw()
{
println("A circle is drawn")
}
}
class PaperDraw : Drawing()
{
override fun draw() {
println("A circle is drawn on a paper")
}
}
Output
A circle is drawn on a paper
A circle is drawn
package inheritance
open class Animal(val name: String, val age: Int) {
open fun makeSound() {
println("Animal makes a sound.")
}
}
class Dog(name: String, age: Int, private val breed: String) : Animal(name, age) {
override fun makeSound() {
println("Dog barks.")
}
fun displayInfo() {
println("Name: $name, Age: $age, Breed: $breed")
}
}
fun main() {
val dog = Dog("Buddy", 3, "Labrador")
dog.makeSound() // Output: Dog barks.
dog.displayInfo() // Output: Name: Buddy, Age: 3, Breed: Labrador
}
Output:
Dog barks.
Name: Buddy, Age: 3, Breed: Labrador
Passing Constructor Arguments to Superclass
package inheritance
open class Animals(val name: String, val sound: String) {
fun makeSound() {
println("$name makes a sound: $sound")
}
}
class Cat(name: String) : Animals(name = name, sound = "meow")
fun main() {
val animal: Animals = Cat("Whiskers")
animal.makeSound()
}
Whiskers makes a sound: meow
In Kotlin, inheritance is a strong tool that helps reuse code, keep code organized, and make it easier to maintain. When developers design class hierarchies well, they can fully use Kotlin’s inheritance and build applications that are flexible and can grow as needed.