Kotlin Flow Beginner Tutorial: Let’s Handle Data Streams
Welcome to this Kotlin Flow Beginner tutorial. Kotlin Flow provides a simple yet powerful way to handle data streams. It’s a crucial part of Kotlin’s Coroutine library, making asynchronous tasks easier to manage. You can use it for network requests, database operations and more without blocking the main thread.
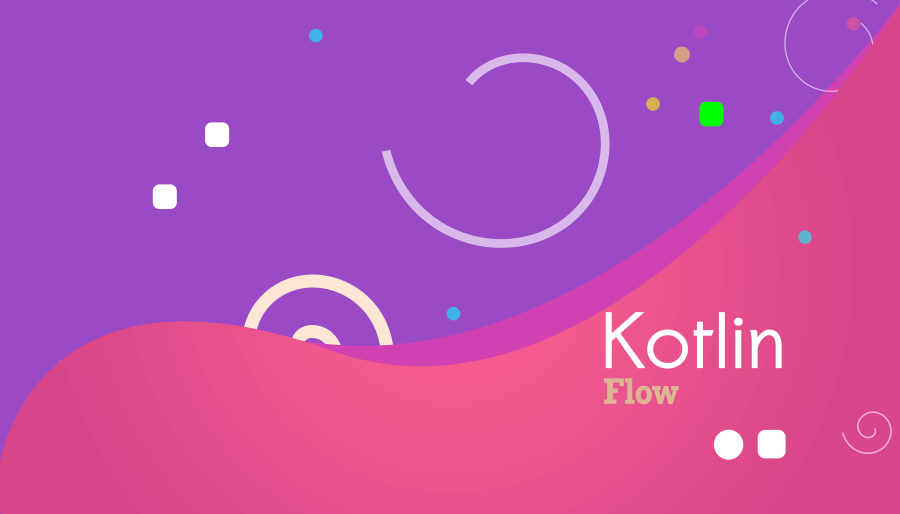
What is Kotlin Flow?
Kotlin Flow allows you to manage reactive streams of data using Coroutines. Unlike LiveData, Kotlin Flow gives you more control over data emission and transformation. This control makes it suitable for scenarios requiring efficient data stream management.
Here’s a simple Kotlin Flow example:
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.flow
import kotlinx.coroutines.runBlocking
fun simpleFun() : Flow<Int> = flow {
for(i in 1..3)
emit(i);
}
fun main() = runBlocking {
simpleFun().collect {
value -> println(value)
}
}
Output
1
2
3
This simpleFun function emits values from 1 to 3. The collect function gathers these values and prints them. Mastering this basic concept is crucial for every beginner.
Why Use Kotlin Flow?
Kotlin Flow offers several advantages:
Efficient Asynchronous Handling
Flow allows easy management of asynchronous streams of data. Whether fetching data from a network or reading from a database, Flow handles these tasks effectively.
Cold Streams
Kotlin Flow operates with cold streams, meaning data flows only when you collect it. This feature optimizes resource usage.
Automatic Cancellation
Flow respects Coroutine’s structured concurrency, canceling ongoing tasks when no longer needed. This feature prevents memory leaks and other issues in long-running operations.
Creating Your First Flow
Creating a Flow that emits a sequence of numbers is a great start. Here’s how you can do it:
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.flow
fun numberFlow(): Flow<Int> = flow {
for (i in 1..5) {
emit(i) // Emits numbers from 1 to 5
}
}
This numberFlow function emits numbers from 1 to 5. The emit function sends each number downstream to the collector. To see this in action, you need to collect the Flow:
import kotlinx.coroutines.runBlocking
fun main() = runBlocking {
numberFlow().collect { number -> println("Received: $number") }
}
Running this code prints each number as it’s emitted. This simple example illustrates the core idea behind Kotlin Flow.
Transforming Data with Flow Operators
Kotlin Flow provides several operators for transforming data streams. Understanding these operators helps you manipulate emitted data effectively.
map Operator
The map operator transforms data emitted by the Flow. For instance, you might square each number before collecting it:
numberFlow()
.map { it * it }
.collect { squaredNumber -> println("Squared: $squaredNumber") }
This code squares each number before printing it. The map operator is versatile and can handle more complex transformations as needed.
filter Operator
The filter operator allows emission of only specific items that meet a condition. For example, you can emit only even numbers:
numberFlow()
.filter { it % 2 == 0 }
.collect { evenNumber -> println("Even: $evenNumber") }
Only even numbers are collected and printed. This operator makes handling specific cases in your data stream simple.
Handling Errors in Flow
Errors are a part of programming, but Kotlin Flow makes handling them straightforward. You can use the catch operator to manage exceptions effectively:
numberFlow()
.map { check(it <= 3) { "Number too large" } }
.catch { e -> println("Caught an error: $e") }
.collect { println("Received: $it") }
The check function throws an exception if the number exceeds 3. The catch operator intercepts this exception, allowing graceful error handling.
Combining Multiple Flows
Kotlin Flow allows you to combine multiple flows using operators like zip, combine, and merge. Here’s how you can zip two flows:
val flow1 = flowOf(1, 2, 3)
val flow2 = flowOf("A", "B", "C")
flow1.zip(flow2) { num, letter -> "$num$letter" }
.collect { println("Zipped: $it") }
This example combines numbers and letters into pairs, demonstrating how you can merge different data streams efficiently.
Final Thoughts
Kotlin Flow offers a robust way to manage asynchronous data streams in Kotlin applications. For every Kotlin Flow beginner, mastering the basics is essential. Start by creating simple flows, then explore operators for transforming and managing your data. Understanding these core concepts unlocks the full potential of Kotlin Flow in your projects.