Crafting an Effective Android App Architecture: An Easy Guide
Creating a well-structured architecture is crucial for developing maintainable, scalable, and efficient Android applications. An effective architecture ensures separation of concerns modularity, and easy testability. In this tutorial, we will dive into the essential components of Android app architecture, exploring various patterns and best practices to help you build high-quality apps.
MVC (Model-View-Controller) : Android App Architecture
The Model-View-Controller architecture is one of the foundational patterns in Android development. It divides your app into three main components:
Model: Represent the data and business logic. It handles data manipulation, validation and storage.
View: Displays the user interface. It captures user input and renders data from the model. View should be kept as dump as possible, avoiding complex logic.
Controller: Acts as intermediary between the model and view. It receives user input form the view, interacts with the model, and updates the view accordingly.
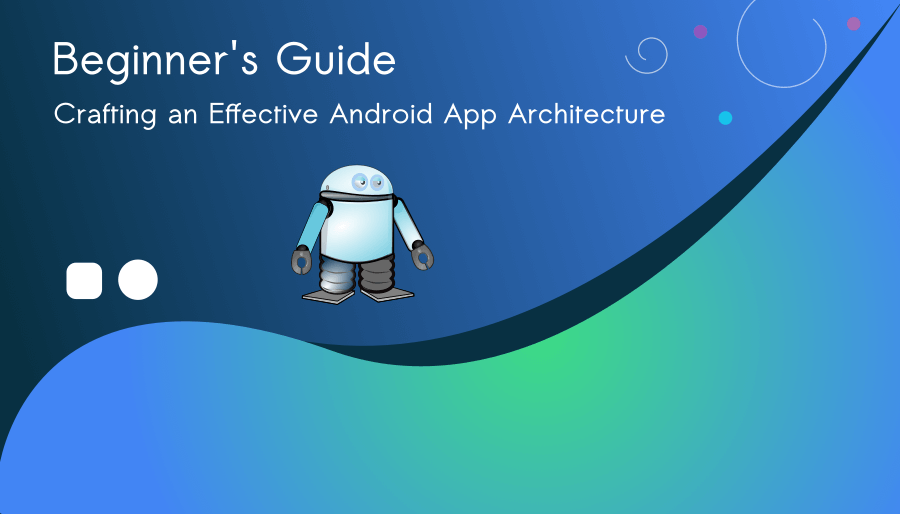
MVP (Model-View-Presenter)
MVP is an evolution of MVC that focuses on better separation of concerns. It addresses the issue of tightly coupled views and controllers by introducing presenters:
Model: Same as in MVC, handling data and business logic.
View: Passive interface that displays data and forwards user input to the presenter.
Presenter: Handles user input, interacts with the model, and updates the view. It eliminates direct interaction between the view and the model.
MVVM (Model-View-ViewModel)
MVVM is a modern architecture pattern that promotes a cleaner separation between the user interface and the business logic.
Model: Unchanged, manages data and business logic.
View: Renders data and captures user input, but it doesn’t contain complex logic. It binds to the ViewModel.
ViewModel: Exposes data and commands that the view needs. It abstracts the UI state and logic from the view, making it highly testable.
Clean Architecture
Clean Architecture focuses on decoupling the app’s components, making it easier to maintain and test:
Entities: Represent core business models.
Use Cases : Contain application-specific business rules.
Repository: Provides an abstraction for data sources, separating the app from data storage.
Frameworks & Drivers: Houses the UI and external components like databases, frameworks, and libraries.
Architecture Components
Android provides a set of architecture components that simplify the development process:
Room: A SQLite abstraction library that makes database handling seamless.
ViewModel: Manages UI-related data, surviving configuration changes.
LiveData: Delivers data changes to the UI in a lifecycle-aware manner.
Navigation: Handles navigation between different screens in a modular way.
Dependency Injection
Dependency Injection (DI) is crucial for building modular and testable apps. It reduces tight coupling between classes and promotes reusability:
Testing in Architecture
Effective testing ensures the reliability of your architecture. Employ these strategies:
Unit Testing: Test individual components in isolation, verifying functionality.
Integration Testing: Test how different components interact.
UI Testing: Automate tests to mimic user interactions and validate the UI behavior.
Verdict
The choice of architecture depends on your project’s complexity, team expertise, and future scalability needs. Consider the trade-offs before making a decision.
A solid app architecture is the foundation of a successful Android application. By adopting a structured approach and following established patterns, you can create apps that are maintainable, testable, and scalable. Whether you opt for MVC, MVP, MVVM, or Clean Architecture, always prioritize separation of concerns, modularity, and code reusability for a smoother development process and a high-quality end product.