Dependency Injection in Android : Simple Explanation
As an Android developer, you might have come across the term “Dependency Injection” when exploring software design patterns or best practices.
Dependency Injection (DI) is a powerful concept that promotes modularity, reusability, and testability in your Android applications.
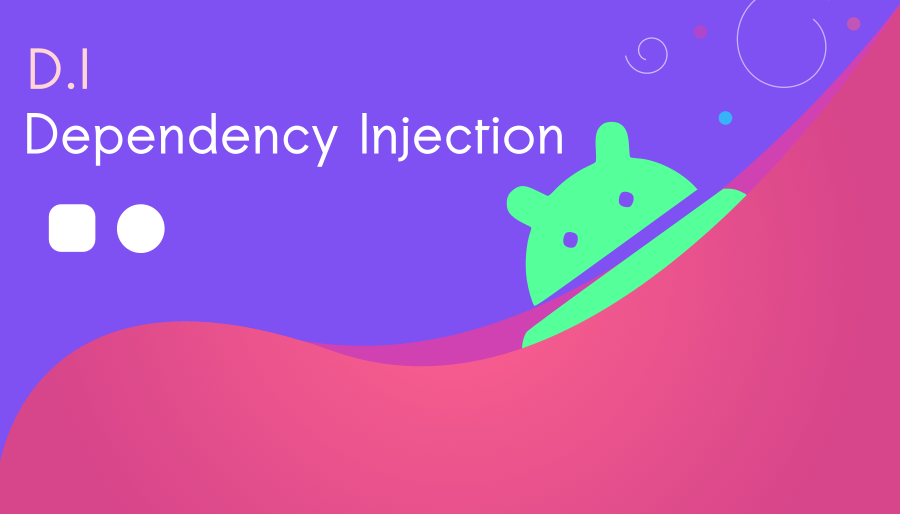
What is Dependency Injection in Android?
Dependency Injection is a design pattern that allows the separation of object creation and dependency resolution from the class that uses those dependencies.
In simpler terms, it is a way to provide the required dependencies to a class from an external source, rather than the class creating them itself. This external source is typically called the “dependency injector” or “container.”
Why is Dependency Injection important in Android development?
Dependency Injection offers several advantages in Android development
Modularity and Reusability
By decoupling dependencies from the classes that use them, you can create modules that are more modular, independent, and reusable.
Each class can focus on its specific responsibility without worrying about how dependencies are obtained.
Testability
Dependency Injection greatly simplifies unit testing. With DI, you can easily mock or substitute dependencies during testing, allowing for isolated and more effective unit tests.
This helps identify and fix issues early in the development process.
Flexibility and Maintainability
Dependency Injection promotes loose coupling between components, making it easier to replace or modify dependencies without affecting the overall structure of your application.
This flexibility enhances maintainability and makes codebase more adaptable to future changes.
Implementing Dependency Injection
There are several popular Dependency Injection frameworks available for Android, such as Dagger, Koin, and Hilt.
Define dependencies: Start by identifying the dependencies your class requires and create separate classes or interfaces for them.
Create a Module – Create a Dagger module that provides the necessary dependencies. Annotate the module with @Module and provide methods annotated with @Provides to define how the dependencies are created.
Inject dependencies – Annotate the class where you want to inject dependencies with @Inject. Dagger will automatically look for the required dependencies in the provided modules and inject them into the class.
Set up the Dagger component – Create a Dagger component interface and annotate it with @Component. Define the modules to be used in the component using the modules attribute.
Build the Dagger component – Build the Dagger component using the generated Dagger class. This component acts as the entry point for dependency injection in your application.
Use the injected dependencies : Once the Dagger component is built, you can access the injected dependencies by calling the appropriate methods on the component.
Dependency Injection is a powerful design pattern that can greatly enhance the modularity, testability, and maintainability of your Android applications.
By separating the creation and resolution of dependencies, you can build more flexible and reusable code.
Android provides several Dependency Injection frameworks like Dagger, Koin, and Hilt to simplify the implementation process.