A Comprehensive Guide to Dart Null Safety for Beginners
What is Null Safety ?
Dart offers a feature called “Null Safety” which is designed to eliminate the possibility of null references, and to make it easier for developers to write safe and reliable code. In this article, we will discuss what Null Safety is in Dart and provide examples to help you understand its functionality.
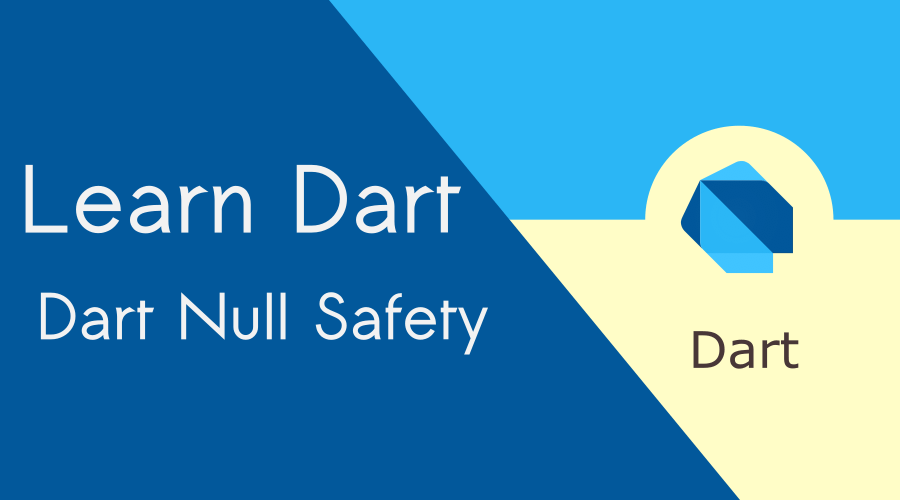
Null Safety in Dart
Null Safety is a feature in Dart that helps developers avoid null reference errors. In a null-safe program, the type system guarantees that variables are non-null, and any null reference errors are caught at compile-time.
This helps developers write code that is less prone to bugs and makes the program more stable.
Dart has introduced two new syntax elements to support null safety: the “?” and “!” operators. The “?” operator is used to indicate that a variable may be null, while the “!” operator is used to indicate that a variable is non-null.
The “?” and “!” operators are used with variable names, function calls, and other expressions.
Open the Dart Pad or Android Studio for writing the below code.
The below Dart code declares a variable x of type String without initialising it to any value, and then prints its value using the print function.
void main() {
String x;
print(x); // Error
}
However, this code will produce a runtime error because the variable ‘x’ has not been initialised and therefor has a ‘null’ value.
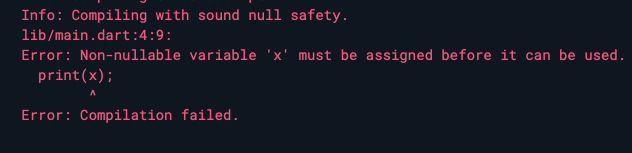
In Dart’s null safety feature, variables can’t have a null value unless it is explicitly defined with the ? operator.
To fix the error, you can either initialize the variable x to a non-null value, or use the null-aware operator ? when declaring the variable, like this:
void main() {
String? x; // Declare a nullable string variable
print(x); // Output: null
}
In Dart, you can declare variables with or without the “late” keyword. If you declare a variable with the “late” keyword, you must initialize it before you can use it. If you declare a variable without the “late” keyword, it can be null.
void main() {
// Declare a variable without the "late" keyword
String? name;
// Declare a variable with the "late" keyword
late String lastName;
print(name); // possible
//print(lastName); // not possible
lastName = "SK";
print(lastName); // possible
}
In this example, the “name” variable can be null because it is declared with the “?” operator. The “lastName” variable is declared with the “late” keyword, which means it must be initialized before it can be used.
Null Safety is a powerful feature in Dart that helps developers avoid null reference errors. By using the “?” and “!” operators, developers can declare variables and function parameters as nullable or non-nullable, and handle null values with conditional expressions.