Learn Dart in 30 Minutes Beginner’s Guide
In this beginner’s guide to learning Dart programming language, you can learn the basics of Dart in just 30 minutes. This article is ideal for anyone who wants to start learning Dart, whether you are a beginner or an experienced programmer. By following this guide, you will be introduced to the syntax and features of Dart and gain an understanding of how to use it for web and mobile app development.
What is Dart ?
Dart is an object-oriented programming language that was developed by Google in 2011. It is a general-purpose language that is used for developing web, mobile, and desktop applications. Dart is similar to JavaScript in many ways, but it is designed to be faster, more efficient, and easier to learn. In this blog post, we will introduce you to the basics of Dart programming.
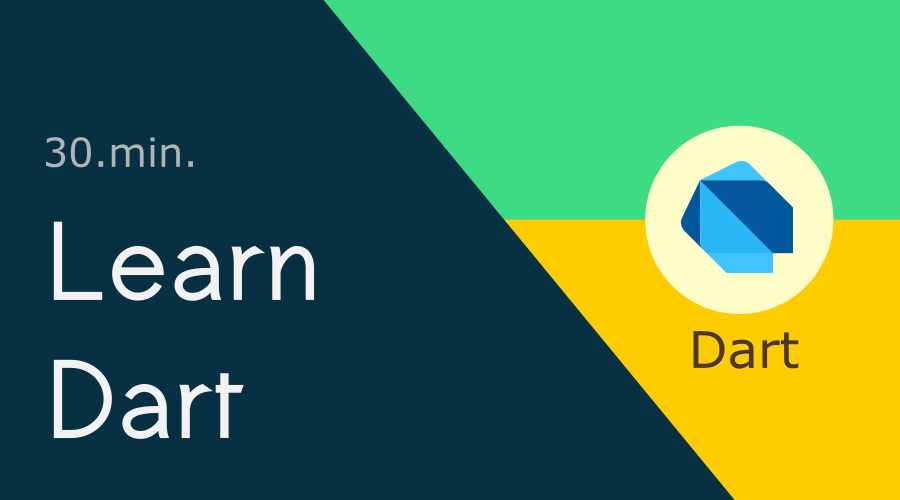
Variables and Data Types
Like most programming languages, Dart allows you to declare variables. You can use the var keyword to declare a variable without specifying its data type, or you can specify the data type using one of the following keywords: int, double, bool, or String.
Here is an example of how to declare a variable:
void main() {
var name = 'John';
String city = 'New York';
int age = 30;
double height = 1.85;
bool isMarried = false;
}
Operators
Dart supports many operators that you can use to perform arithmetic, logical, and comparison operations. Here are some examples of the most commonly used operators:
- Arithmetic operators: +, -, *, /, %
- Logical operators: && (AND), || (OR), ! (NOT)
- Comparison operators: == (equal to), != (not equal to), > (greater than), < (less than), >= (greater than or equal to), <= (less than or equal to)
Functions
Functions are a fundamental part of Dart programming. A function is a set of instructions that perform a specific task. Here is an example of how to define a function in Dart:
void main() {
sayHello("Nikin");
}
void sayHello(String name) {
print('Hello, $name!');
}
Output
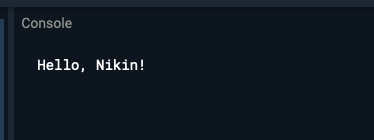
This function takes a string parameter called name and uses the print() function to display a message that includes the name. The void keyword indicates that the function does not return a value.
Control Structures
Dart supports several control structures that you can use to control the flow of your program. These include if-else statements, for loops, while loops, and switch statements. Here is an example of how to use an if-else statement in Dart:
void main() {
var age = 19;
if (age >= 18) {
print('You are an adult.');
} else {
print('You are not an adult yet.');
}
}
Output
You are an adult
This code uses an if-else statement to check whether the variable age is greater than or equal to 18. If it is, the program displays a message indicating that the person is an adult. Otherwise, it displays a message indicating that the person is not yet an adult.
Classes and Objects
Dart is an object-oriented programming language, which means that it supports the use of classes and objects. A class is a blueprint for creating objects, while an object is an instance of a class. Here is an example of how to define a class in Dart:
void main() {
var john = Person();
john.name = 'John';
john.age = 30;
john.sayHello();
}
class Person {
String? name;
int? age;
void sayHello() {
print('Hello, my name is $name and I am $age years old.');
}
}
Output
Hello, my name is John and I am 30 years old.
This code defines a class called Person that has two properties: name and age. It also defines a method called sayHello() that displays a message that includes the person’s name and age.
This code creates an object called john of the Person class, sets its name and age properties, and then calls the sayHello() method.
Conclusion
Dart is a powerful and versatile programming language that is ideal for developing web, mobile, and desktop applications.