Learn Dart Functions: A Beginner’s Guide
One of the most important features of Dart is its support for functions. Functions are a fundamental building block of any programming language, and in Dart, they provide a powerful way to organize code and perform tasks.
In this tutorial, we will cover the basics of Dart functions.
In Dart, a function is a set of statements that perform a specific task. Functions allow us to encapsulate a piece of code and reuse it whenever we need it.
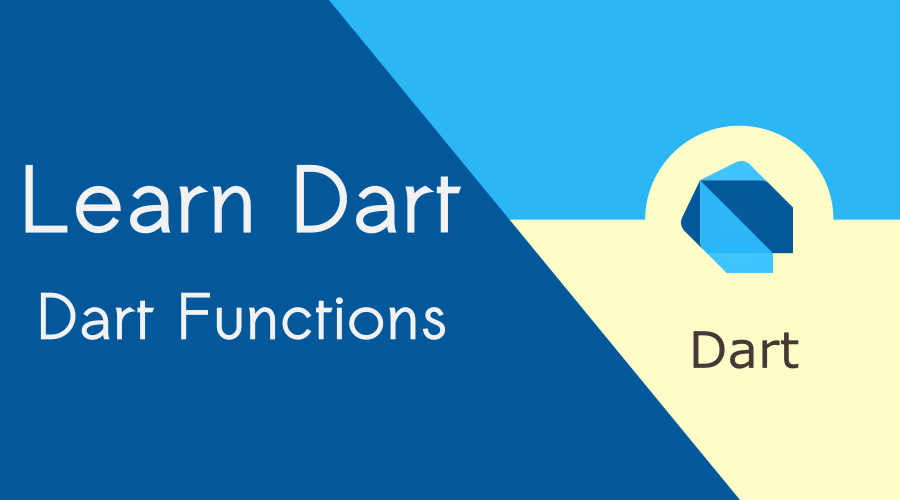
In other words, we can define a function once and call it multiple times with different arguments. Functions can take zero or more parameters, and they can return zero or more values.
Syntax
return_type function_name(parameter1, parameter2, ...) {
// Function body
return value;
}
The return_type is the type of value that the function returns. If the function doesn’t return a value, then the return type should be void.
The function_name is the name of the function, which can be any valid Dart identifier.
The parameters are the input values that the function takes. If the function doesn’t take any parameters, then the parameter list should be empty.
Let’s define a simple function that takes two integers as parameters and returns their sum:
int sum(int a, int b) {
return a + b;
}
Open the Dart Pad and Write the following code
void main()
{
print(sum(5,6));
}
int sum(int a, int b) {
return a + b;
}
The output of the above program will be 11
In this example, the sum function takes two integers as input and returns their sum. The return type of the function is int, which means that the function returns an integer value.
Here, we pass two integers 5 and 6 as arguments to the sum function, and it returns their sum 11.
Dart Function Default Parameters
Dart allows us to specify default values for function parameters. Default parameters are used when the corresponding argument is not provided during function invocation.
Let’s take a look at an example:
void main()
{
errorMessage("Server issue");
}
void errorMessage(String message, {String error = 'Unknown Error'}) {
print('$message : $error');
}
This is a Dart code that defines a function errorMessage that takes two parameters: message and an optional parameter error with a default value of ‘Unknown Error’. The function simply prints out the concatenated string of message and error.
In the main function, the errorMessage function is called with the argument ‘Server issue’ for the message parameter. The output of this program would be the string ‘Server issue : Unknown Error‘.
Positional Parameters in Dart
Dart also allows us to define positional parameters, which are similar to default parameters, but they are mandatory. Positional parameters are denoted by square brackets ([]) in the function signature.
Let’s take a look at an example:
void main()
{
printInfo("Book","Pen", "ID card");
}
void printInfo(String item1, String item2, [String? requiredItem3]) {
print('$item1, $item2, $requiredItem3');
}
Book, Pen, ID
This is a Dart code that defines a function printInfo that takes three parameters: item1, item2, and an optional parameter requiredItem3 that is marked with a ? indicating it can be null (Dart null safety). The function simply prints out the concatenated string of item1, item2, and requiredItem3.
In the main function, the printInfo function is called with the arguments ‘Book’, ‘Pen’, and ‘ID card’. The output of this program would be the string ‘Book, Pen, ID card’.
Note that the requiredItem3 parameter is optional, which means it may or may not be provided in the function call. If it is not provided, it will default to null. The ? after String indicates that the variable can be null.
In this tutorial, we have covered the basics of Dart functions. We have learned how to define functions, and how to use default and positional parameters.