Generic Class in Dart: Let’s Use Different Types
All major programming languages support generics; it’s one of the finest features for handling types. A generic class in Dart also maintains type safety.
What are Generic Classes in Dart?
In simple terms, Dart generic classes and methods enable you to define a class or method with placeholders for the types of its fields, parameters, or return values. Moreover, this awesome flexibility not only enhances readability but also promotes code reusability.
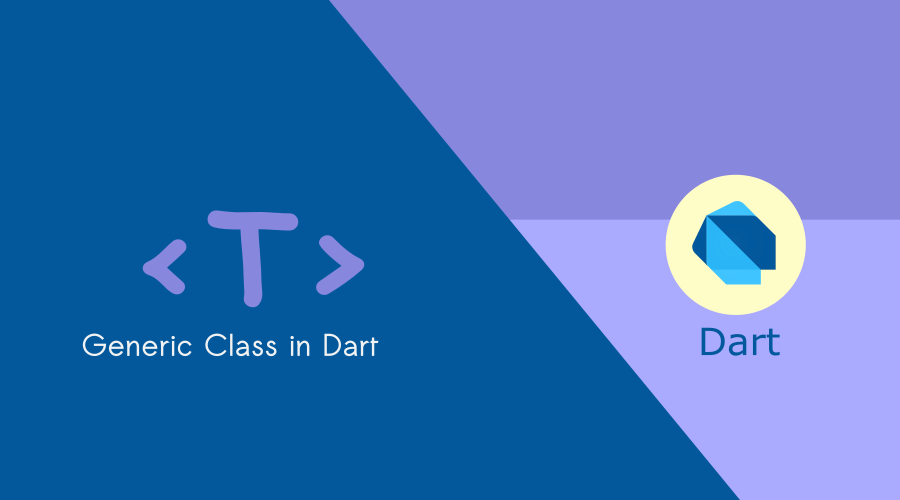
Let’s look at a simple example of a generic class in Dart :
Write the following program in DartPad:
class Tag<T>
{
late T value;
Tag(this.value);
T getTagValue() => value;
}
void main() {
Tag<int> integerTag = Tag<int>(60);
Tag<String> stringTag = Tag<String>('#learn #dart');
print(integerTag.getTagValue());
print(stringTag.getTagValue());
}
Output
60
#learn #dart
If you observe the above program, you might be wondering where ‘T‘ comes into the program. A generic class is one that can work with different types, and the type is specified when creating an instance of the class.
In the above example, the generic class ‘Tag’ serves as a container capable of holding a value of any type, represented by the placeholder ‘T‘. When you create an instance of ‘Tag’, you specify the type within angle brackets (<T>). Additionally, the class features a method named ‘getTagValue,’ responsible for returning the stored value of type T.
Example:
abstract class Tags {
void perform();
}
class Foo<T> implements Tags {
final List<Tags> list;
// Correct constructor implementation
Foo(this.list);
@override
void perform() => print('Start performing...');
}
class TagManager implements Tags {
@override
void perform() => print('Performing from TagManager');
}
void main() {
// Creating an instance of Foo<Tags> with a list containing TagManager
var tag = Foo<Tags>([
TagManager(),
]);
// Accessing the list and calling perform() on each element
for (var item in tag.list) {
item.perform();
}
}
Performing from TagManager
Advantages of Generic Classes
Efficiency
Generic code frequently results in more efficient and optimized code execution. This occurs because the compiler can generate specialized implementations for each type used, enhancing performance.
Safety
Dart’s type system guarantees the enforcement of generic types at compile-time, thereby delivering robust type safety.
Dart’s standard libraries, along with numerous third-party libraries, extensively utilize generics. This results in a rich ecosystem of generic classes and functions that you can efficiently utilize in your applications.
Refactoring
When there is a necessity to modify the type of data a class or method operates on, generics facilitate the process, making it easier to refactor the code without adversely impacting its overall structure.
In summary, using generic classes in Dart offers a powerful mechanism for creating flexible, reusable, and type-safe code.
Happy Darting!