Extension Methods in Dart : Add Functionalities Quickly
Extension methods in Dart allow you to add functionality to existing classes without modifying their actual source code.
Syntax : Extension Methods in Dart
Define an extension using the ‘extension’ keyword, followed by a name and the type it extends.
extension MyExtension on String {
//Add an extension method here.
}
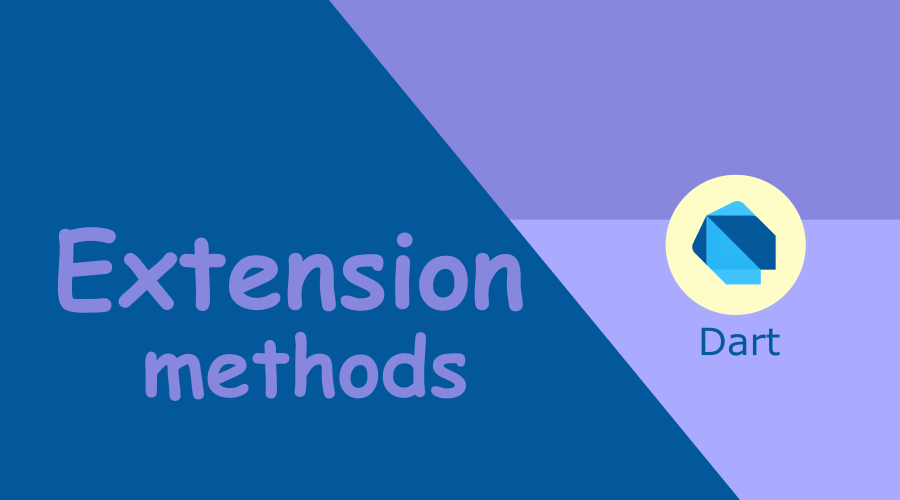
Step 1: Adding a method
Use the ‘this‘ keyword inside the extension to refer to the instance of the type being extended.
extension MyExtension on String {
int wordCount() {
return this.split(' ').length;
}
}
The wordCount() method, implemented within an extension function, calculates and returns the number of words in a given string
Step 2: Applying Extensions
To use an extension method, import the file containing the extension and invoke the method on an instance of the extended type.
void main() {
String text = "Hello I like Lua";
int count = text.wordCount();
print('Word count: $count');
}
extension MyExtension on String {
int wordCount() {
return this.split(' ').length;
}
}
Output:
Word count: 4
Step 3: Handling Null Values
Additionally, it’s super important to ensure null safety when using extensions in dart.
Open DartPad and write the following code:
void main() {
String text = "Hello I like Lua";
int count = text.wordCount();
print('Word count: $count');
}
extension MyExtension on String? {
int wordCount() {
// check if the String is null and return 0 if true
if(this == null) {
return 0;
}
return this?.split(' ')?.length ?? 0;
}
}
Advantages of extension methods in Dart:
Better Code Organization
Extension methods allow developers to organize code more logically. Instead of scattering related functions across multiple files or classes, you can group them together within a single extension, promoting a cleaner codebase.
Readability
By extending existing classes with methods that logically belong to them, code becomes more readable and intuitive. This readability leads to better comprehension and easier maintenance, especially when collaborating with other developers.
Reusability
Extension methods encourage code reuse by allowing developers to define functionality once and apply it to multiple classes as needed. This reduces redundancy.
Clear Intent
Extension methods explicitly declare their intent by extending specific classes. This clarity helps other developers understand the purpose of the extension and encourages consistent usage throughout the codebase.
To sum up, extension methods in Dart provide numerous benefits such as better code organization, heightened readability, non-invasive implementation, increased reusability, simplified retrofitting, and explicit intent declaration. Integrating extension methods into your Dart projects can result in streamlined development workflows and more maintainable codebases.
Happy Coding with Dart!