Inheritance in Dart : Single, required and super keywords
Inheritance is a fundamental feature of all modern programming languages. In Dart, inheritance allows a class to inherit properties and behavior from another class. This tutorial will guide you through the various concepts related to inheritance in the Dart language.
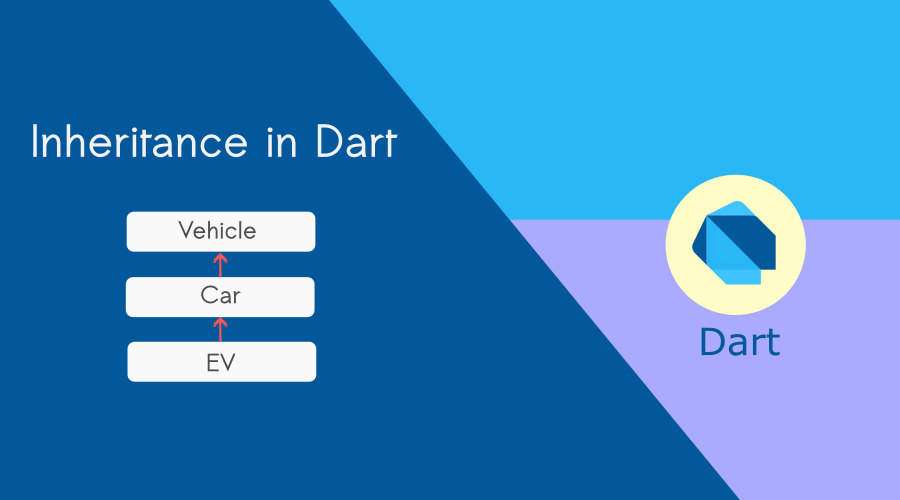
Prerequisites for this tutorial (optional)
Understanding Inheritance in Dart
Inheritance in Dart enables the creation of a new class by reusing and extending the features of an existing class. This is super helpful for achieving code reusability and a hierarchical structure in your project’s codebase.
How can a class be made inheritable in Dart?
The syntax of inheritance is straightforward because Dart uses the ‘extends‘ keyword, as do many other programming languages. To create a subclass in Dart, use the ‘extends‘ keyword followed by the superclass name. An example is given below.
Example:
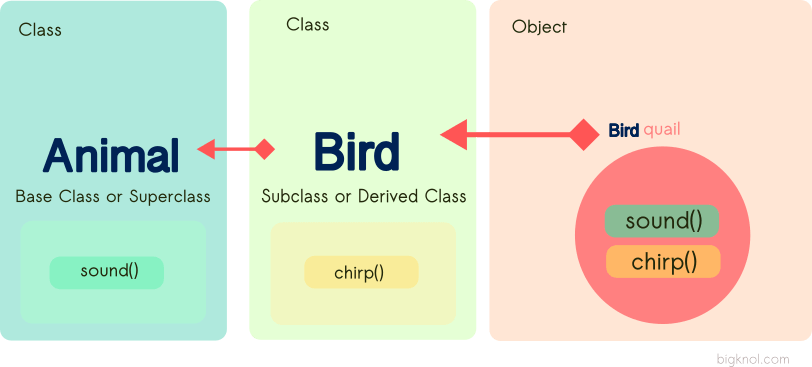
class Animal {
void sound() {
print("Animals make sound!");
}
}
class Bird extends Animal{
void chirp(){
print("wuvoo.. wuvoo");
}
}
void main() {
Bird quail = Bird();
quail.sound();
quail.chirp();
}
Animals make sound!
wuvoo.. wuvoo
If you observe the above program, the Animal class has a method sound() that prints the message ‘Animals make sound.’ There is another class called Bird that extends (inherits from) the superclass Animal. The object ‘quail‘ is used to invoke the superclass method sound() and the subclass (derived class) method chirp().
Single Inheritance in Dart
To implement single inheritance in Dart, use the ‘extends’ keyword followed by the name of the superclass.
Example :
Open DartPad and write your fist inheritance program:
class School {
String? name;
School(String? name){
this.name = name;
}
void info(){
print("School Name: $name");
}
}
class Teacher extends School{
String? id;
Teacher(super.name, {required id}){
this.id = id;
}
void info_teacher() {
print('Teacher ID: $id');
}
}
void main(){
Teacher new_teacher = Teacher('S.N School', id: 'RX1001VB');
new_teacher.info();
new_teacher.info_teacher();
}
School Name: S.N School
Teacher ID: RX1001VB
The code presents a Teacher class that extends (inherits from) the School class. The Teacher class introduces a String variable ‘id‘ and a constructor that takes the ‘name’ parameter from the superclass (School) along with a required ‘id’ parameter. The constructor initializes the ‘id’ field with the provided value.
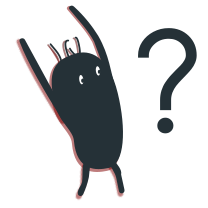
Read more about : Null Safety in Dart
Q1
Why do we use curly braces {} with parameters?
Here, I can see {required id}.
In Dart, using curly braces {} in parameters indicates that the parameters are named parameters.
Named parameters are useful when there are a lot of parameters to pass to a function or when creating an instance of a class. They provide a clear idea of what values we are passing.
Teacher new_teacher = Teacher('S.N School', id: 'RX1001VB');
Passing an id like ‘RX1001VB’ here will not be useful, as any other programmer may not quickly understand the purpose of this value. If you set the parameter ‘id’ as named, it will show ‘id:’ before passing the argument.
Q2
What is ‘required’ ?
The ‘required‘ keyword indicates that the named parameter must be provided. When used in the context of constructor parameters, ‘required‘ indicates that the corresponding parameter must be provided.
Q3
Why do we use ‘super’ keyword?
The ‘super’ keyword is used to refer to the superclass, enabling a subclass to access members (fields or methods) of its immediate superclass.
It is also helpful for calling the superclass constructor, as shown below:
class Car {
String? type;
Car(String type)
{
this.type = type;
print('Initializing car type..');
}
}
class Electric extends Car {
int? range;
Electric(String? type, int range): super(type!){
print('Constructor is called for showing range: $range');
}
}
void main() {
Car newCar = Electric('EV', 450);
}
Initializing car type..
Constructor is called for showing range: 450
Three advantages of using inheritance include:
Code Reusability
One of the primary benefits of inheritance is the capability to reuse code. By inheriting from another class, a class automatically gains access to the properties and methods of the superclass.
Polymorphism
Polymorphism, a fundamental concept in object-oriented programming,It enables the treatment of objects from different classes as objects of a shared superclass.
Code Organization and Abstraction
Inheritance fosters a hierarchical organization of classes, empowering developers to establish a clear and structured class hierarchy.
Inheritance supports the abstraction of common characteristics and behaviors in a superclass, capturing shared functionality at a higher level.
Happy Coding!