Getting Started with Dart for Loop: An Introduction
Dart provides a few different types of loops, but in this tutorial, we will focus on the for loop.
The for loop is a commonly used loop in Dart that allows you to iterate over a sequence of values, such as a list or an array.
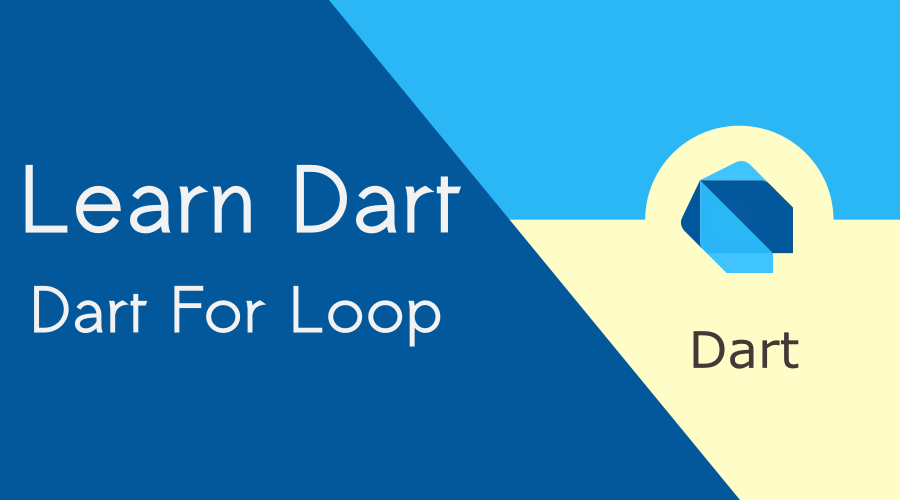
Syntax
for (initialisation; condition; increment/decrement) {
// code block to be executed
}
The initialization statement is executed once before the loop starts. It typically initializes a variable that controls the loop’s iteration, like an index variable.
The condition statement is evaluated before each iteration, and if it evaluates to true, the code block inside the loop is executed. Otherwise, the loop is terminated.
The increment/decrement statement is executed after each iteration, and it typically increments or decrements the index variable.
void main() {
for (int i = 0; i < 5; i++) {
print('hello ${i + 1}');
}
}
Output
hello 1
hello 2
hello 3
hello 4
hello 5
This Dart code snippet is an example of a for loop that prints out “hello” followed by a number, where the number is one more than the index of the current iteration. Let’s break down the code line by line.
This line initializes a new integer variable i to 0 and sets the loop condition to run as long as i is less than 5. The i++ statement increments i by 1 after each iteration.
print(‘hello ${i + 1}’);
This line prints out the string “hello” followed by the value of i + 1. Since i starts at 0, the first printed line will be “hello 1”. This will be followed by hello 2 … hello 5 as the loop runs.
Let’s use StringBuffer in Dart Program
void main() {
var x = StringBuffer("I like BigKnol");
for(var i=0; i<5; i++)
{
x.write("!");
}
print(x);
}
Output
I like BigKnol!!!!!
StringBuffer is a built-in class that represents a mutable sequence of characters. It provides a way to efficiently build up a string by appending or inserting characters, without the overhead of creating a new string for every concatenation operation.
Example : For Loop in Dart for List
This Dart code snippet is an example of using a for loop to iterate over a list of strings and printing each element to the console.
Open Dart Pad and Write the following code.
void main() {
List<String> os = ["Android","iOS","WearOS","Ubuntu"];
for(var i=0; i<os.length; i++)
{
print(os[i]);
}
}
Output
Android
iOS
WearOS
Ubuntu
List<String> os = ["Android","iOS","WearOS","Ubuntu"];
This line creates a new list of strings called os
and initializes it with four elements: “Android”, “iOS”, “WearOS”, and “Ubuntu”.
This is a for loop that iterates over the os list. The loop variable i is initialized to 0, and the loop condition is set to run as long as i is less than the length of the os list.
Example : Nested for Loop in Dart language
void main() {
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 2; j++) {
print('$i, $j');
}
}
}
Output
1, 1
1, 2
2, 1
2, 2
3, 1
3, 2
This output shows that the nested for loop has iterated over every possible combination of the values 1 through 3 and 1 through 2.
It’s important to note that nested for loops can be resource-intensive and can slow down your program if used excessively. So, it’s important to use them only when necessary and optimize your code for efficiency.