Flutter Performance Optimization: Build Blazing Fast Apps in 2025
This handy guide explores better Flutter performance optimization techniques.
Ever launched your Flutter app and felt that subtle lag that makes you wince? I’ve been there. After spending countless hours building the perfect app, watching it stutter can feel like a punch to the gut. But here’s the thing – performance optimization isn’t just about making your app faster; it’s about creating experiences that keep users coming back.
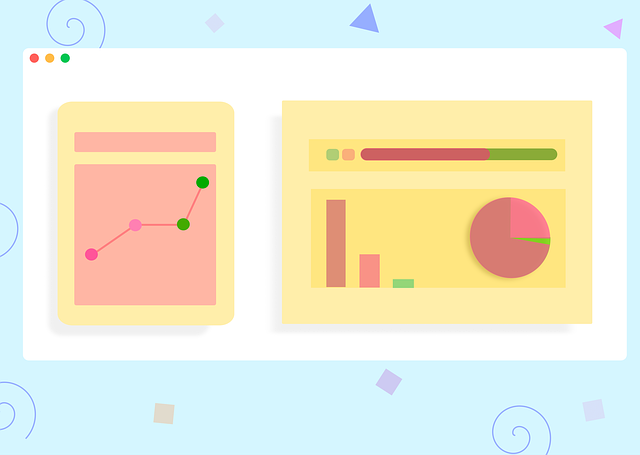
Understanding Flutter Performance: The Basics
Remember when we used to think adding more RAM would solve all our performance problems? Those were simpler times. Flutter performance optimization is more like conducting an orchestra – every instrument needs to play its part perfectly. Let me break this down in a way that actually makes sense.
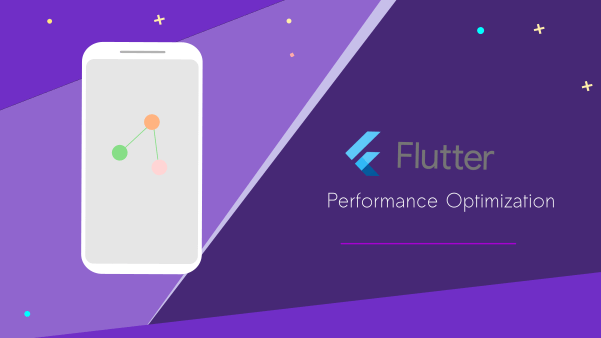
What Really Impacts Flutter Performance?
You know that feeling when you’re trying to run through water? That’s what an unoptimized Flutter app feels like to your users. Here are the key players in the performance game:
- Widget rebuilds (the silent performance killer)
- Memory management (your app’s breathing room)
- Asset loading (the heavy lifting)
- State management (the puppet master)
Common Performance Issues and Their Impact
Issue | Impact Level | User Experience Effect |
Excessive rebuilds | High | Stuttering UI |
Memory leaks | Critical | App crashes |
Large assets | Medium | Slow loading times |
Poor state management | High | UI freezes |
The Art of Widget Optimization
Let’s get real for a second – I once had an app that rebuilt its entire widget tree every time a user blinked (okay, slight exaggeration, but you get the point). Here’s how to avoid my rookie mistakes:
1. Embrace const Constructors
// Instead of this
Container(
padding: EdgeInsets.all(8.0),
child: Text('Hello')
)
// Do this
const Container(
padding: EdgeInsets.all(8.0),
child: Text('Hello')
)
2. Strategic Widget Building
Think of your widget tree like a family tree – not everyone needs to know about every little change. I’ll show you how to keep those chatty widgets in check:
class OptimizedList extends StatelessWidget {
const OptimizedList({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: 1000,
itemBuilder: (context, index) {
return const ListItem(); // Notice the const!
},
);
}
}
Memory Management: Flutter Performance Optimization
Let me tell you a story about a memory leak that had me debugging until 3 AM (fueled by coffee and determination). Here’s what I learned:
Image Optimization Techniques
- Implement proper caching
- Use appropriate image formats
- Lazy load when possible
Building for Speed: Practical Tips
These are the techniques that have saved my apps (and my sanity):
Profile Mode Testing
flutter run --profile
Why profile mode? Because running in debug mode is like trying to judge a car’s speed with the parking brake on.
The BuildContext Diet
Remember: every unnecessary BuildContext lookup is like making your app run an extra lap. Here’s how to keep it lean:
// Instead of multiple context lookups
Theme.of(context).textTheme.headline1;
MediaQuery.of(context).size.width;
// Store what you need
final textTheme = Theme.of(context).textTheme;
final screenWidth = MediaQuery.of(context).size.width;
The Road to Smoother Animations
Animations are like jokes – timing is everything. Here’s how to keep them smooth:
- Use AnimatedBuilder wisely
- Implement custom tickers
- Optimize render objects
Measuring Success: Performance Metrics
Let’s talk numbers (don’t worry, the fun kind):
- Frame rate (aim for that sweet 60 FPS)
- Memory footprint
- Launch time
- Response time
Conclusion: Your Next Steps
Performance optimization isn’t a one-time thing – it’s a journey. Start with the basics we’ve covered, measure your improvements, and keep iterating. Your users might not notice when everything runs perfectly, but they’ll definitely notice when it doesn’t.
Flutter performance optimization is a meticulous process that requires significant attention both before and after launching your apps on the respective app stores. Keep a close eye on every activity that consumes a significant amount of resources.
Ready to supercharge your Flutter app? Start implementing these optimization techniques today, and don’t forget to bookmark this guide for future reference.