Null Aware Operator in Dart
In Dart, null safety is enforced, which means that values are non-nullable by default unless explicitly marked as nullable. This ensures that you explicitly indicate when a value can be null. Let’s explore the null aware operator in the Dart language.
Dart, the programming language used for developing Flutter applications, introduces a powerful feature called the Null Aware Operator. This operator simplifies null handling by providing a concise and safe way to work with nullable values.
The Null Aware Access Operator, denoted by ?., allows developers to access properties and methods on an object only if it is not null.
It provides a convenient way to avoid null reference errors by short-circuiting the expression and returning null if the object is null.
For instance, object?.property will safely access property if object is not null; otherwise, it will return null. This operator significantly reduces the need for excessive null checks and improves code readability.
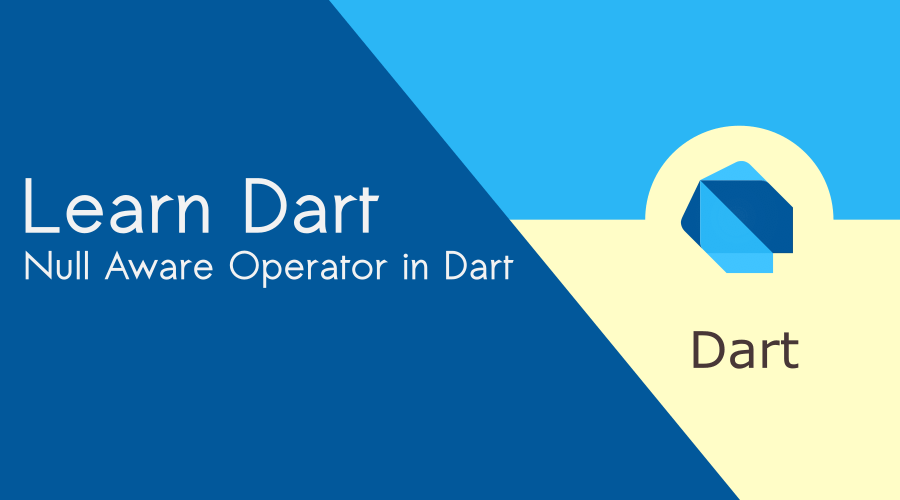
How to use Null Aware Operator in Dart ?
Consider the following program | Try this on Dartpad
void main() {
int? a; // = null
a ??= 43;
print(a); // <-- Prints 43.
a ??= 15;
print(a); // <-- Still prints 43
}
In the given code, we have an integer variable a that is declared as nullable (int?) and initially assigned the value null.
We then use the null-aware assignment operator (??=) to assign a new value to a only if it is currently null.
int? a; // = null
Here, we declare the variable a as nullable using the int? type. Since it is not assigned a value explicitly, it is initialized as null.
a ??= 43;
In this line, we use the null-aware assignment operator (??=) to assign 43 to a only if a is currently null.
Since ‘a’ is null at this point, the assignment takes place, and a is assigned the value 43.
print(a); // <– Prints 43.
Here, we print the value of a, which is now 43. Since the null-aware assignment operator only assigns a new value if the variable is currently null, and it was indeed null at the time of the assignment, it prints 43.
a ??= 15;
In this line, we use the null-aware assignment operator again to assign 15 to a only if a is currently null.
However, since ‘a’ already has a value (43) from the previous assignment, the assignment does not occur, and the value of a remains unchanged.