While and Do While in Dart Programming Language
In Dart, loops provide powerful constructs for executing a block of code repeatedly. Two commonly used loop types are the while loop and the do-while loop. Let’s explore the while and do while in Dart language.
Understanding these looping mechanisms will empower you to write more efficient and flexible code.
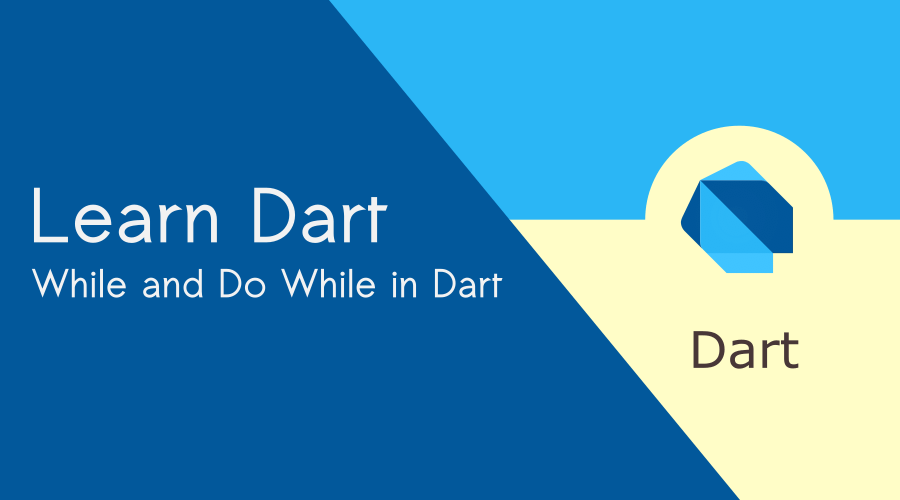
While Loop in Dart
The while loop is a fundamental looping construct that repeatedly executes a block of code as long as a specified condition is true. The syntax of the while loop in Dart is as follows:
while (condition) {
// code to be executed
}
A while loop evaluates the condition before the loop.
he condition is a boolean expression that determines whether the loop should continue executing. The loop checks the condition before each iteration.
If the condition evaluates to true, the code block inside the loop is executed. Once the condition evaluates to false, the loop terminates, and the program continues with the next statement.
The while loop is useful when the number of iterations is not known beforehand and depends on a specific condition.
It allows you to repeat a set of instructions until the condition becomes false. However, be cautious to ensure that the condition eventually becomes false; otherwise, you may end up in an infinite loop.
a while loop to print numbers from 1 to 5
Open Dart pad and write the following program.
void main() {
int i = 1;
while (i <= 5) {
print(i);
i++;
}
}
Output
1
2
3
4
5
Do-While Loop in Dart
The do-while loop is similar to the while loop, but it guarantees that the code block inside the loop is executed at least once, even if the condition is initially false.
The syntax of the do-while loop in Dart is as follows:
do {
// code to be executed
} while (condition);
A do-while loop evaluates the condition after the loop.
In this loop, the code block is executed first, and then the condition is checked. If the condition evaluates to true, the loop continues executing, and the process repeats.
If the condition evaluates to false, the loop terminates, and the program proceeds to the next statement.
The do-while loop is suitable when you need to execute a block of code at least once, regardless of the initial condition.
It is commonly used in scenarios where you want to prompt the user for input and ensure that the code block executes before checking the condition.
a do-while loop to calculate the factorial of a number
void main() {
int number = 5;
int factorial = 1;
int i = 1;
do {
factorial *= i;
i++;
} while (i <= number);
print("Factorial of $number is $factorial");
}
Output
Factorial of 5 is 120
The while and do-while loops are powerful constructs in Dart that enable you to iterate over code blocks based on specific conditions.
While the while loop validates the condition before executing the code block, the do-while loop executes the code block first and then checks the condition.
Understanding these loops will enhance your ability to design flexible and efficient code structures in Dart.