JSON File Reading in Kotlin: Using Gson and resources folder
This tutorial explores the process of reading a JSON file from a resources folder in IntelliJ IDEA. Let’s explore JSON file reading in Kotlin with an example.
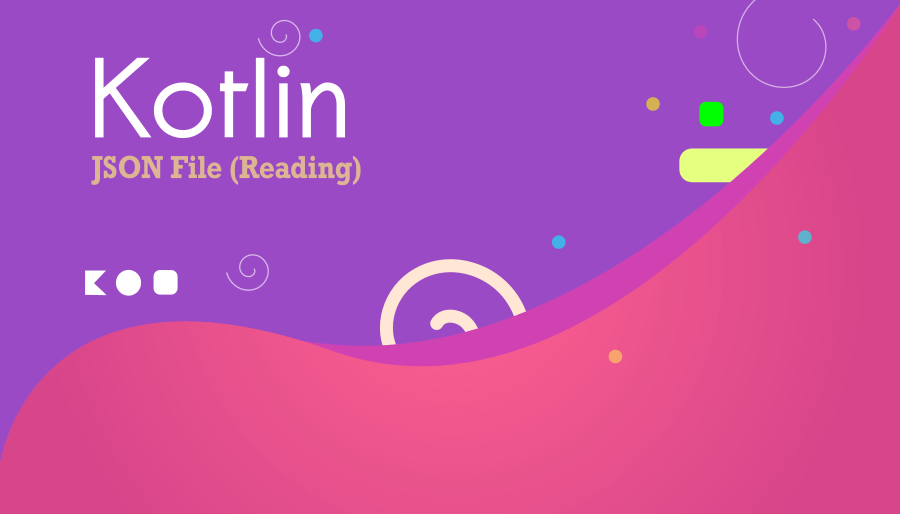
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data interchange format that programmers use to transmit structured data between systems. It employs a human-readable text format to represent data objects consisting of attribute-value pairs.
JSON File
A JSON file is a text file that stores data in the JavaScript Object Notation (JSON) format. It contains structured data represented in attribute-value pairs, organized in a human-readable format. JSON files are commonly used for data exchange between applications, web services, and databases due to their simplicity and ease of parsing across different programming languages.
To extract values from a JSON file using a data class in Kotlin, you’ll need to follow these steps:
Step 1: Create a JSON file
Open the IntelliJ IDEA IDE and Create a new Kotlin project.
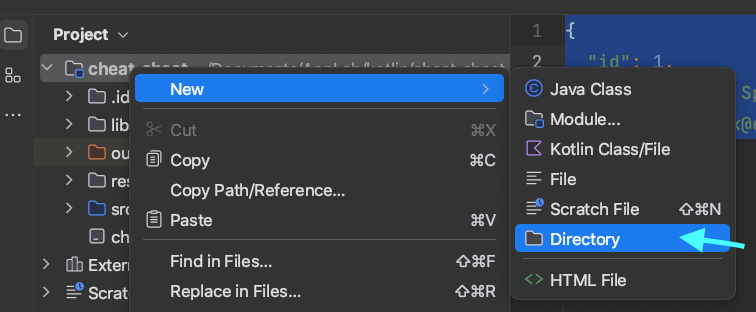
Let’s create a new directory for holding the JSON file. Select your Project > New > Directory. Give directory names as ‘resources‘
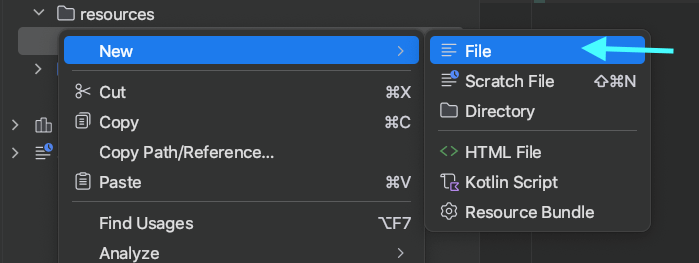
Let’s create a file (New > File) data.json
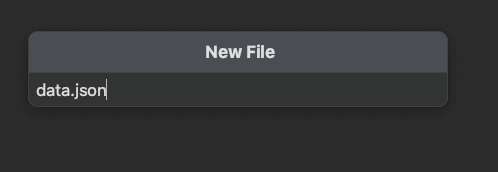
data.json
{
"id": 1,
"name": "Jack Sparrow",
"email": "jack@example.com"
}
Step 2: Define a Data Class for reading JSON (Kotlin)
Create a data class that represents the structure of the JSON data you want to extract. For instance, if your JSON contains information about users, create a corresponding data class. You can convert JSON to a Kotlin data class using either an online or offline method.
Create a data class
User.kt
data class User(
val id: Int,
val name: String,
val email: String
)
Step 3: Add GSON library
Gson, a Java library, converts Java Objects into JSON representations and vice versa, effortlessly handling JSON-to-Java object conversions.
Read the JSON file and parse its content into an object using a library called Gson. Let’s add a new library / dependency to IntelliJ IDEA.
com.google.code.gson:gson:2.10.1
Step 4: Read the JSON file and Print the data
Main.kt
import com.google.gson.Gson
import java.io.File
fun main() {
val jsonFile = File("resources/data.json")
val jsonString = jsonFile.readText()
val gson = Gson()
val user:User = gson.fromJson(jsonString, User::class.java)
println("User ID : ${user.id}")
println("Name : ${user.name}")
println("Email: ${user.email}")
}
Once the JSON content is parsed into the User object, you can access its properties directly to retrieve the values stored in the JSON file.
Performing JSON file reading in Kotlin is a seamless process, facilitating efficient data handling and manipulation.
This example assumes that the JSON file contains data for a single user. If your JSON file contains an array of users or more complex structures, adjustments to the parsing logic will be needed to handle those cases.
Output
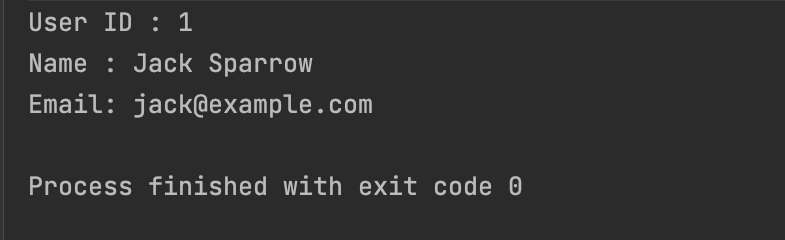
Choose a JSON parsing library that best fits your project requirements. Gson, Moshi, and Kotlinx Serialization are commonly used and well-documented libraries for JSON parsing in Kotlin.