8 Reasons Why Kotlin is Better than Java
There are compelling reasons why Kotlin is emerging as the preferred choice for many developers. In this article, we’ll explore these reasons and shed light on why Kotlin is better than Java for modern software development.
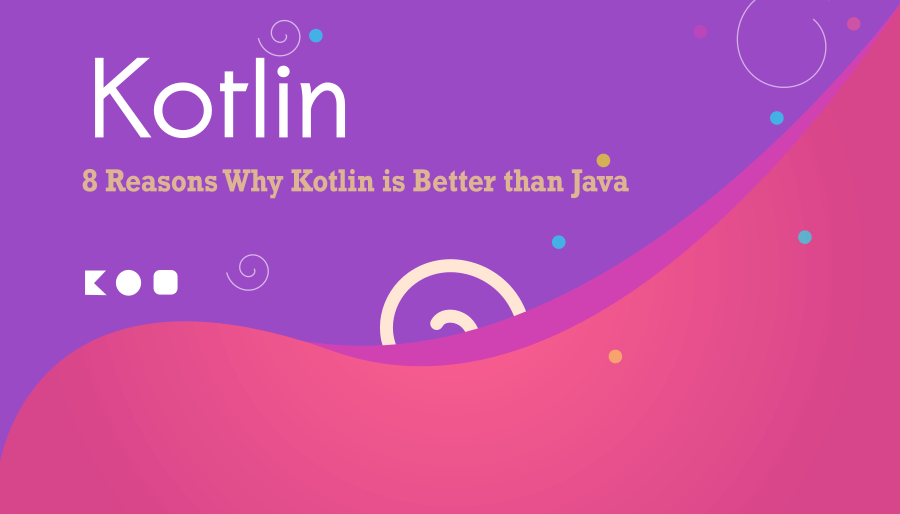
1. Conciseness and Readability
One of the key advantages of Kotlin over Java is its conciseness. Kotlin code tends to be more concise and readable, reducing boilerplate code significantly. This means you can accomplish the same tasks with fewer lines of code, making your codebase cleaner and more maintainable.2.2
2. Null Safety
Kotlin’s null safety features make it a safer choice when it comes to avoiding dreaded NullPointerExceptions. With Kotlin, you explicitly specify whether a variable can be null or not, reducing the chances of runtime crashes caused by null values.
3. Interoperability with Java
Kotlin has excellent interoperability with Java, allowing you to seamlessly integrate Kotlin into existing Java projects. This means you can migrate gradually, taking advantage of Kotlin’s features without having to rewrite your entire codebase.
4. Modern Language Features
Kotlin is a modern language designed with the latest programming concepts in mind. It includes features like lambdas, extension functions, and smart casts, which enhance your productivity and make your code more expressive.
5. Extension Functions
Kotlin introduces extension functions, enabling you to add new functionality to existing classes without modifying their source code. This is a powerful feature that promotes cleaner and more modular code.
6. Functional Programming
Kotlin embraces functional programming concepts, providing first-class support for functions as objects. Java introduced functional programming features in later versions, but Kotlin’s implementation is more elegant and intuitive.
7. Asynchronous Programming with Coroutines
Kotlin Coroutines simplify asynchronous programming. With coroutines, you can write non-blocking code in a more sequential and readable manner. It allows you to fetch user data from the network without blocking the main thread, resulting in a more responsive application.
8. Community
Kotlin has a strong global community with plenty of support and contributors. You can benefit from a wide range of community libraries and get help easily, either from the community or the Kotlin team.
Kotlin vs. Java: A Direct Comparison with Examples
Now, let’s compare Kotlin and Java directly in various aspects to highlight why Kotlin is better than Java. Let’s explore coding examples where Kotlin shines.
Syntax Clarity and Verbosity
Kotlin’s concise syntax reduces the verbosity seen in Java. For example, consider the code to create a simple class:
Java:
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Kotlin:
class Person(val name: String)
The Kotlin version is much more concise, making it easier to read and write.
Conciseness and Null Safety
Java:
public double calculateAverage(List<Integer> scores) {
List<Integer> validScores = new ArrayList<>();
for (Integer score : scores) {
if (score != null) {
validScores.add(score);
}
}
double sum = 0;
for (Integer score : validScores) {
sum += score;
}
return sum / validScores.size();
}
Kotlin:
fun calculateAverage(scores: List<Int?>): Double {
val validScores = scores.filterNotNull()
return validScores.sum().toDouble() / validScores.size
}
In this example, Kotlin’s concise syntax and null safety features allow you to write cleaner and more readable code. The Kotlin version removes the need for explicit null checks and reduces the boilerplate code.
Kotlin Shines: Data Classes
Java:
public class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
return age == person.age && Objects.equals(name, person.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
Kotlin:
data class Person(val name: String, val age: Int)
In Kotlin, you can define data classes with just a single line, which automatically generates useful methods like equals(), hashCode(), and toString(). This reduces boilerplate code and makes it easier to work with data objects.
Modern Development
In conclusion, Kotlin’s conciseness, null safety, Java interoperability, modern language features, and extension functions make it a compelling choice for modern software development. While Java has a long-standing history and a vast ecosystem, Kotlin offers a more concise and expressive way to write code, reducing the chances of errors and improving developer productivity.
So, when it comes to the age-old debate of Kotlin vs. Java, the answer is clear: Kotlin is better than Java for many of today’s development needs. Its concise and expressive syntax, null safety, and modern features set it apart as a language ready to take on the challenges of modern software development. Make the switch to Kotlin, and you’ll likely find yourself writing cleaner, safer, and more efficient code in no time.