AdMob Anchor Ads : Try Anchored Adaptive Banner Ad in Android
Adaptive banners represent the evolution of responsive ads, aiming to enhance performance through the optimization of ad size tailored to individual devices. They build upon the capabilities of smart banners, which were limited to fixed heights, by introducing the ability for developers to define the ad width. This newfound control allows for the determination of the most suitable ad size. Let’s implement AdMob Anchor Ads in an Android app using Java.
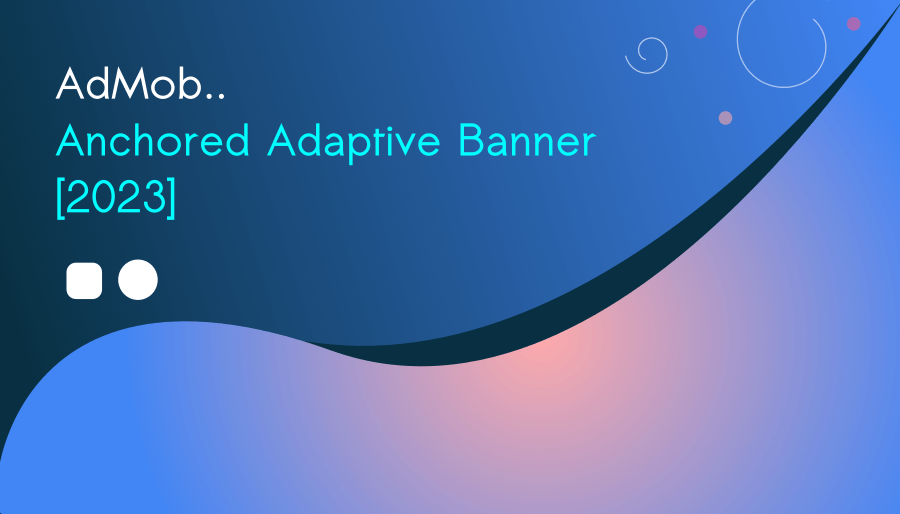
An adaptive banner leverages the available screen size more effectively. Moreover, when compared to a smart banner, choosing an adaptive banner offers several advantages:
- By utilizing a specified width instead of occupying the entire screen width, an adaptive banner provides the flexibility to accommodate display cutouts. This feature allows for seamless integration with devices that have non-standard screen configurations.
- Instead of maintaining a constant height across devices of varying sizes, an adaptive banner dynamically selects an optimal height for each specific device. This approach effectively addresses the challenges posed by device fragmentation, as it ensures the banner’s height is optimized to fit seamlessly within the unique dimensions of each device.
AdMob Anchor Ads : How to show in your Android app?
Let’s create an Android Project using Android Studio (Flamingo)
Open the AndroidManifest.xml file and add the following App ID (It’s a test ID).
<!-- Sample AdMob App ID: ca-app-pub-3940256099942544~3347511713 -->
<meta-data
android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="ca-app-pub-3940256099942544~3347511713"/>
Add a dependency into your build.gradle(:app) file:
implementation 'com.google.android.gms:play-services-ads:22.2.0'
To showcase an anchored adaptive banner within your app, you will typically come across two essential files: “AnchoredBannerAd.java” and “activity_anchored_banner_ad.xml”. However, depending on your project, you might encounter a different main activity file, such as “MainActivity.java”, along with its corresponding XML file.
Let’s create an ad container for displaying the ad in your app.
<FrameLayout
android:id="@+id/bannerAd_container"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:layout_alignParentBottom="true" />
Add the above frame layout to activity_anchored_banner_ad.xml
activity_anchored_banner_ad.xml (Full code) :
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:ads="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/transparent"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
tools:context="com.bigknol.AnchoredBannerAd">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginStart="16dp"
android:text="App Content Area" />
<FrameLayout
android:id="@+id/bannerAd_container"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:layout_alignParentBottom="true" />
</RelativeLayout>
Initialize the AdMob SDK
Log.d(TAG, "Google Mobile Ads SDK Version: " + MobileAds.getVersion());
// Initialize the Mobile Ads SDK.
MobileAds.initialize(this, new OnInitializationCompleteListener() {
@Override
public void onInitializationComplete(InitializationStatus initializationStatus) {}
});
To ensure proper testing of ads on your mobile devices, it is crucial to either utilize the test ID or employ the setRequestConfiguration()
method. This helps in creating a suitable environment for ad testing.
// Test your Ad
MobileAds.setRequestConfiguration(
new RequestConfiguration.Builder().setTestDeviceIds(Arrays.asList("ABCDEF012345")).build());
Please substitute the item in the ArrayList with the appropriate device ID for your own device.
Set up your Ad Unit ID :
private static final String BANNER_ID = "ca-app-pub-3940256099942544/6300978111";
private static final String TAG = "AchoredBannerAd";
private FrameLayout bannerContainerView;
private boolean initialLayoutComplete = false;
private AdView adView;
Create two methods called loadBanner() and getAdSize().
getAdSize() :
private AdSize getAdSize() {
// Determine the screen width (less decorations) to use for the ad width.
Display display = getWindowManager().getDefaultDisplay();
DisplayMetrics outMetrics = new DisplayMetrics();
display.getMetrics(outMetrics);
float density = outMetrics.density;
float adWidthPixels = bannerContainerView.getWidth();
// If the ad hasn't been laid out, default to the full screen width.
if (adWidthPixels == 0) {
adWidthPixels = outMetrics.widthPixels;
}
int adWidth = (int) (adWidthPixels / density);
return AdSize.getCurrentOrientationAnchoredAdaptiveBannerAdSize(this, adWidth);
}
loadBanner():
private void loadBanner() {
// Create an ad request.
adView = new AdView(this);
adView.setAdUnitId(BANNER_ID);
bannerContainerView.removeAllViews();
bannerContainerView.addView(adView);
AdSize adSize = getAdSize();
adView.setAdSize(adSize);
AdRequest adRequest = new AdRequest.Builder().build();
// Start loading the ad in the background.
adView.loadAd(adRequest);
}
Let’s load and display the anchored adaptive banner ad in your Android app.
bannerContainerView = findViewById(R.id.bannerAd_container);
bannerContainerView.getViewTreeObserver().addOnGlobalLayoutListener(new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
if(!initialLayoutComplete)
{
initialLayoutComplete = true;
loadBanner();
}
}
});
The getViewTreeObserver() method returns the ViewTreeObserver object associated with the bannerContainerView, which allows us to listen for changes in the view hierarchy.
The addOnGlobalLayoutListener() method is used to add a listener to the ViewTreeObserver. It takes an instance of the OnGlobalLayoutListener interface as a parameter.
In the code, a new instance of OnGlobalLayoutListener is created as an anonymous inner class. This class overrides the onGlobalLayout() method, which is called when the global layout event occurs.
AnchoredBannerAd.java (Complete code)
package com.bigknol;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.util.DisplayMetrics;
import android.util.Log;
import android.view.Display;
import android.view.ViewTreeObserver;
import android.widget.FrameLayout;
import com.bigknol.slideimage.R;
import com.google.android.gms.ads.AdRequest;
import com.google.android.gms.ads.AdSize;
import com.google.android.gms.ads.AdView;
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.RequestConfiguration;
import com.google.android.gms.ads.initialization.InitializationStatus;
import com.google.android.gms.ads.initialization.OnInitializationCompleteListener;
import java.util.Arrays;
public class AnchoredBannerAd extends AppCompatActivity {
private static final String BANNER_ID = "ca-app-pub-3940256099942544/6300978111";
private static final String TAG = "AchoredBannerAd";
private FrameLayout bannerContainerView;
private boolean initialLayoutComplete = false;
private AdView adView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_anchored_banner_ad);
// Log the Mobile Ads SDK version.
Log.d(TAG, "Google Mobile Ads SDK Version: " + MobileAds.getVersion());
// Initialize the Mobile Ads SDK.
MobileAds.initialize(this, new OnInitializationCompleteListener() {
@Override
public void onInitializationComplete(InitializationStatus initializationStatus) {}
});
// Test your Ad
MobileAds.setRequestConfiguration(
new RequestConfiguration.Builder().setTestDeviceIds(Arrays.asList("ABCDEF012345")).build());
bannerContainerView = findViewById(R.id.bannerAd_container);
bannerContainerView.getViewTreeObserver().addOnGlobalLayoutListener(new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
if(!initialLayoutComplete)
{
initialLayoutComplete = true;
loadBanner();
}
}
});
}
// method for loading ad
private void loadBanner() {
// Create an ad request.
adView = new AdView(this);
adView.setAdUnitId(BANNER_ID);
bannerContainerView.removeAllViews();
bannerContainerView.addView(adView);
AdSize adSize = getAdSize();
adView.setAdSize(adSize);
AdRequest adRequest = new AdRequest.Builder().build();
// Start loading the ad in the background.
adView.loadAd(adRequest);
}
// get device size
private AdSize getAdSize() {
// Determine the screen width (less decorations) to use for the ad width.
Display display = getWindowManager().getDefaultDisplay();
DisplayMetrics outMetrics = new DisplayMetrics();
display.getMetrics(outMetrics);
float density = outMetrics.density;
float adWidthPixels = bannerContainerView.getWidth();
// If the ad hasn't been laid out, default to the full screen width.
if (adWidthPixels == 0) {
adWidthPixels = outMetrics.widthPixels;
}
int adWidth = (int) (adWidthPixels / density);
return AdSize.getCurrentOrientationAnchoredAdaptiveBannerAdSize(this, adWidth);
}
//ends
/** Called when leaving the activity */
@Override
public void onPause() {
if (adView != null) {
adView.pause();
}
super.onPause();
}
/** Called when returning to the activity */
@Override
public void onResume() {
super.onResume();
if (adView != null) {
adView.resume();
}
}
/** Called before the activity is destroyed */
@Override
public void onDestroy() {
if (adView != null) {
adView.destroy();
}
super.onDestroy();
}
}
Output
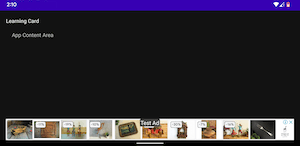
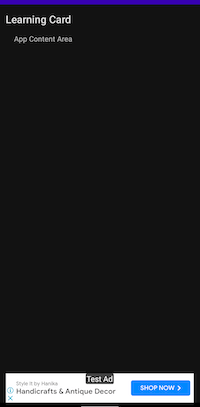
Happy Coding!