50 Kotlin Interview Questions Crafted for Android Developers
Kotlin, a powerful and versatile programming language, has gained immense popularity in the Android development community and beyond. As more companies adopt Kotlin for their projects, the demand for skilled Kotlin developers continues to rise. Whether you’re preparing for a job interview or aiming to deepen your Kotlin expertise, the guide gives you 100 Kotlin interview questions and answers to help you crack any Kotlin related job interview.
1. What is Kotlin?
Kotlin is a statically-typed programming language developed by JetBrains. It runs on the Java Virtual Machine (JVM) and is fully interoperable with Java, making it an excellent choice for Android app development and backend services.
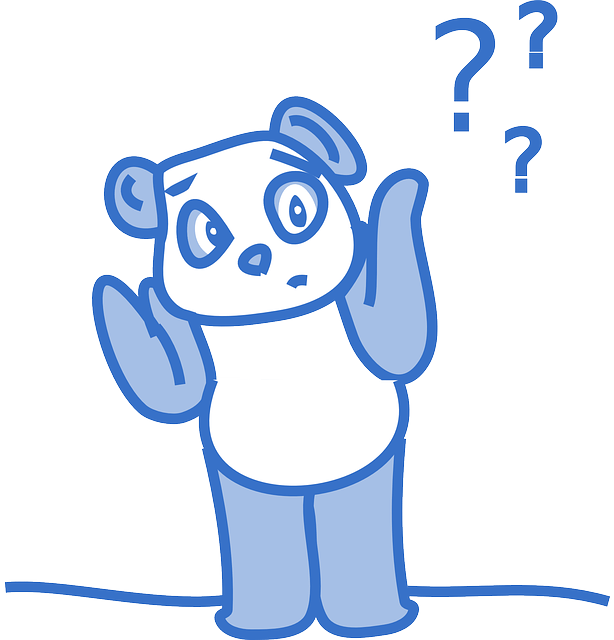
Does Kotlin need JVM every time?
Kotlin is intentionally designed to be versatile, supporting a wide array of platforms like Android, JVM, JS, and Native. Kotlin/JVM applications necessitate the use of JVM. However, with Kotlin/JS and Kotlin/Native, there is no reliance on JDK and JVM.
2. What are the main advantages of using Kotlin for Android development?
Kotlin offers concise and expressive syntax, null safety, extension functions, coroutines for asynchronous programming, seamless Java interop, and improved type inference, which makes it a more productive and safe language for Android development.
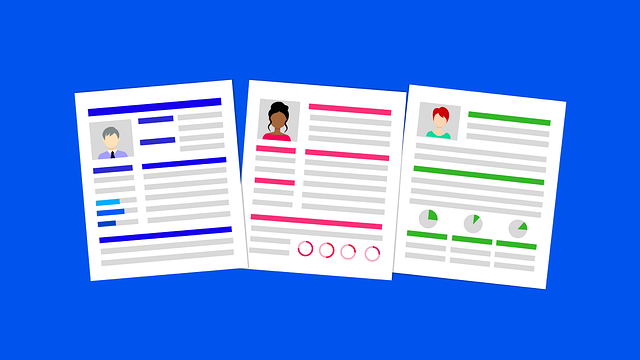
3. How do you declare a variable in Kotlin?
In Kotlin, you declare a variable using the “val” keyword for read-only (immutable) variables and “var” for mutable variables. For example:
val pi = 3.14
var count = 0
Learn more about Kotlin variables
4. Explain the difference between “val” and “var”
“val” creates read-only variables, meaning their values cannot be changed after initialization. “var” creates mutable variables that can be modified throughout their lifecycle.
5. What is the Elvis operator (?:) in Kotlin used for?
The Elvis operator (?:) is used to handle null values. It allows you to provide a default value if a nullable variable is null. For example:
fun main() {
val firstName: String? = null
val showFirstName = firstName ?: "Unknown Name"
println(showFirstName)
}
Output
Unknown Name
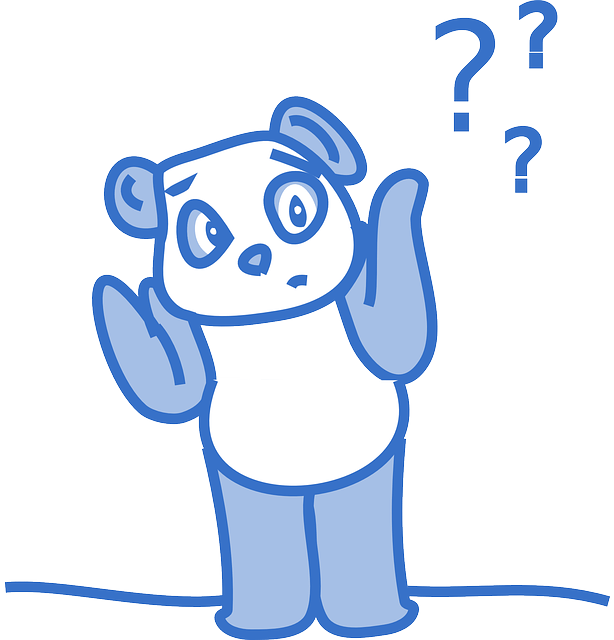
Tricky Question : It appears that you are referring to the conditional operator. Is it possible to utilize the conditional operator in Kotlin?
Learn more about Conditional Operator alternative in Kotlin
6. What is a data class in Kotlin?
A data class is a special Kotlin class used for holding data. It automatically generates equals(), hashCode(), toString(), and copy() methods based on its properties. For example:
data class foo(val name:String)
7. How do you create an extension function in Kotlin?
To create an extension function in Kotlin, define a function outside any class and prefix the receiver type before the function name.
fun main() {
val str = "Hello BigKnol!".Demo()
println(str)
}
fun String.Demo(): String {
return this.uppercase();
}
Output : HELLO BIGKNOL!
8. Explain the “when” expression in Kotlin
The “when” expression in Kotlin is similar to a switch-case statement in other languages. It allows you to perform different actions based on the value of an expression.
val dayOfWeek = 3
val dayString = when (dayOfWeek) {
1 -> "Sunday"
2 -> "Monday"
// Add cases for other days
else -> "Invalid day"
}
9. How do you handle null safety in Kotlin?
Kotlin provides null safety by distinguishing between nullable and non-nullable types. To declare a nullable variable, use the question mark “?” after the type
var name: String? = "Nikky"
10. Explain the concept of smart casts in Kotlin ?
Kotlin’s smart casts automatically cast a variable to a non-nullable type after null-checking. It eliminates the need for explicit type casting.
fun printLength(str: String?) {
if (str != null) {
println(str.length) // No need for explicit cast, smart cast occurs
}
}
11. Can you explain about Kotlin Scope functions?
let: Used for scoping non-null objects and performing operations on them.
run: Similar to let, but can be used on both nullable and non-null objects. It returns the result of the last expression within the block.
with: Used for scoping an object without the need for a lambda parameter.
apply: Used for configuring an object during its initialization and returns the object itself.
also: Similar to apply, but it returns the object itself rather than the last expression.
12. What are coroutines in Kotlin?
Coroutines are Kotlin’s lightweight threads used for asynchronous programming. They provide an efficient way to perform long-running tasks without blocking the main thread, leading to smoother user experiences.
13. Can you show/write a simple coroutine example?
To define a coroutine, use the launch or async function from the CoroutineScope
import kotlinx.coroutines.*
fun main() {
GlobalScope.launch {
// Coroutine code here
}
}
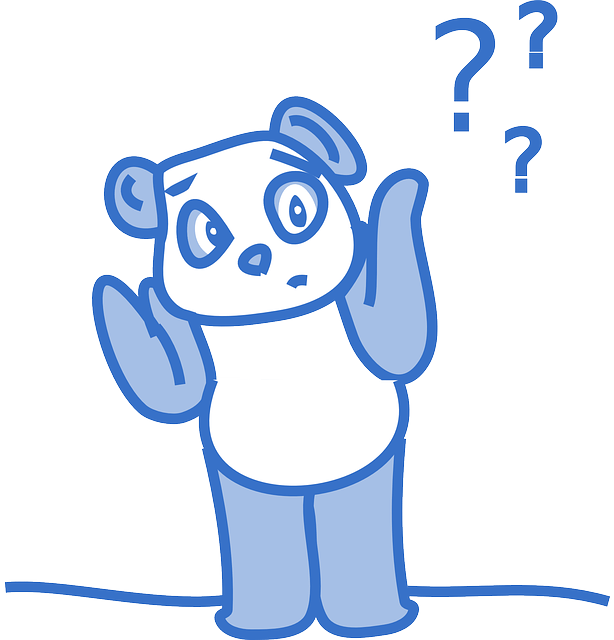
What are launch and async?
launch: Starts a new coroutine and returns a Job, which is used for handling the lifecycle of the coroutine. It does not return a result.
async: Starts a new coroutine and returns a Deferred, which is a lightweight non-blocking future that eventually produces a result.
14. How do you define a suspend function in Kotlin?
To define a suspend function, use the suspend modifier before the function declaration. Suspend functions can be called from other coroutines and are used for asynchronous operations.
suspend fun main(){
println(showMessage())
}
suspend fun showMessage(): String {
// Asynchronous operations
return "I did it"
}
Output : I did it
15. What is the purpose of the async function in Kotlin coroutines?
The async function is used to perform concurrent asynchronous operations. It returns a Deferred object representing a future result, which can be awaited using the await()
16. How do you perform background work with Kotlin coroutines?
You can use withContext(Dispatchers.IO) to perform background work inside a coroutine.
import kotlinx.coroutines.*
suspend fun fetchDataFromNetwork(): String {
return withContext(Dispatchers.IO) {
// Perform network request here
"Data from network"
}
}
suspend fun main() {
println(fetchDataFromNetwork())
}
Output (for demo only) : Data from network
17. What is the role of the CoroutineDispatcher in Kotlin coroutines?
A CoroutineDispatcher is responsible for scheduling and running coroutines on specific threads. It defines the context in which coroutines execute their code. The Dispatchers object provides several pre-defined dispatchers, such as Main, IO, and Default.
18. Explain the purpose of GlobalScope in Kotlin coroutines?
GlobalScope is a predefined coroutine scope that can be used for launching global coroutines that live throughout the lifetime of the entire application. It is recommended to use other coroutine scopes like viewModelScope, lifecycleScope, or custom scopes to better manage the lifecycle of coroutines.
19. How do you cancel a coroutine in Kotlin?
To cancel a coroutine, you can call the cancel() function on its corresponding Job.
val job = GlobalScope.launch {
// Coroutine code here
}
// Cancel the coroutine
job.cancel()
20. What is the difference between cancel() and cancelAndJoin() in Kotlin coroutines?
cancel(): Cancels the coroutine and returns immediately.
cancelAndJoin(): Cancels the coroutine and suspends the current thread until the coroutine is completed or canceled.
21. What are the main threading options available in Android?
In Android, the main threading options are:
Main Thread (UI Thread): Used for handling UI interactions.
Background Threads: Used for performing time-consuming tasks without blocking the UI thread.
AsyncTask: Deprecated since Android 11, used to perform background tasks with a simplified API.
Handlers: Used to send and process messages between threads.
Kotlin Coroutines: Modern and preferred approach for asynchronous programming, allowing easy concurrency without callbacks.
22. What is a higher-order function in Kotlin?
A higher-order function is a function that takes one or more functions as arguments or returns a function as its result. Kotlin supports higher-order functions, enabling functional programming paradigms.
23. What is the purpose of LiveData in Android?
LiveData is an observable data holder class provided by Android Architecture Components. It is used to hold and observe data changes, ensuring that UI components are updated automatically when the data changes.
24. Explain the difference between LiveData and MutableLiveData
LiveData: Represents read-only data that can be observed. It is returned as a LiveData object from the ViewModel.
MutableLiveData: A subclass of LiveData that allows changing the value it holds. It is typically used to update the UI with new data.
25. What is a ViewModel in Android?
A ViewModel is a class provided by Android Architecture Components used to store and manage UI-related data that survives configuration changes, such as screen rotations. It is part of the MVVM (Model-View-ViewModel) pattern.
26. How do you create a custom RecyclerView.Adapter in Android?
class CustomAdapter(private val data: List<String>) :
RecyclerView.Adapter<CustomAdapter.ViewHolder>() {
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
// ViewHolder code here
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
// Inflate and return the view holder
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
// Bind data to the view holder
}
override fun getItemCount(): Int {
return data.size
}
}
27. How do you create a Room database in Android?
To create a Room database, you define an abstract class that extends RoomDatabase and annotate it with @Database. This class represents the database and includes a list of @Entity classes representing the database tables.
@Database(entities = [User::class], version = 1)
abstract class AppDatabase : RoomDatabase() {
abstract fun userDao(): UserDao
}
28. What is the “it” keyword used for in Kotlin?
The “it” keyword is a shorthand for a single parameter in lambda expressions.
29. How do you create an anonymous function in Kotlin?
To create an anonymous function in Kotlin, you use the “fun” keyword without providing a name.
30. How do you define an Entity in Room?
To define an Entity in Room, you create a data class and annotate it with @Entity. You can also define a primary key and other database-related annotations.
@Entity(tableName = "users")
data class User(
@PrimaryKey val id: Long,
val name: String,
val age: Int
)
31. What is the purpose of the Dao class in Room?
The Dao (Data Access Object) class in Room is an interface or abstract class that defines the database operations, such as insert, update, delete, and queries. It allows you to interact with the database using high-level methods.
@Dao
interface UserDao {
@Query("SELECT * FROM users")
fun getAllUsers(): List<User>
@Insert
fun insertUser(user: User)
// Other database operations
}
32. What is the purpose of the @Query annotation in Room?
The @Query annotation in Room is used to define custom SQL queries for database operations. It allows you to perform complex queries and retrieve specific data from the database.
@Dao
interface UserDao {
@Query("SELECT * FROM users WHERE age >= :minAge")
fun getUsersWithMinimumAge(minAge: Int): List<User>
}
33. What is the Navigation component in Android?
The Navigation component is a part of Android Jetpack that simplifies navigation between destinations in an Android app. It provides a visual editor and a set of APIs for handling navigation and passing data between destinations.
34. How do you create a custom ViewModelFactory in Android?
To create a custom ViewModelFactory, subclass ViewModelProvider.Factory and override the create() method to return the appropriate ViewModel instance.
class MyViewModelFactory(private val param: String) : ViewModelProvider.Factory {
override fun <T : ViewModel?> create(modelClass: Class<T>): T {
return MyViewModel(param) as T
}
}
35. Explain the concept of Dependency Injection in Android?
Dependency Injection (DI) is a design pattern used to manage the dependencies of an object and provide them from external sources. It helps to decouple classes and makes them more maintainable, testable, and flexible.
36. What are the main benefits of using Dependency Injection in Android?
The main benefits of using Dependency Injection in Android are:
- Easier testing by providing mock dependencies.
- Increased code modularity and reusability.
- Simplified object creation and reduced boilerplate code.
- Improved separation of concerns.
37. How do you use ProGuard in an Android project?
ProGuard is a tool used to optimize and obfuscate code in an Android project, making it smaller and more difficult to reverse-engineer. To use ProGuard, add the following to the build.gradle file.
38. Explain the purpose of the proguard-rules.pro file in Android.
The proguard-rules.pro file contains the configuration rules for ProGuard. You can customize the rules to specify which classes, methods, or attributes should be kept, obfuscated, or optimized.
39. What is the purpose of the @Keep annotation in Android?
The @Keep annotation is used to instruct ProGuard not to remove or obfuscate the annotated class, method, or field, even if it seems unused or redundant. It is useful for preserving certain classes, especially those used with reflection or from external libraries.
40. How do you enable multidex support in an Android project?
Multidex support allows an Android app to have multiple DEX files, accommodating apps that exceed the 65k method limit. To enable multidex, add the following to the build.gradle file:
android {
defaultConfig {
multiDexEnabled true
}
}
dependencies {
implementation 'androidx.multidex:multidex:2.0.1'
}
41. How do you handle runtime permissions in Android?
To handle runtime permissions in Android, you need to request the necessary permissions from the user at runtime. Use the ActivityCompat.requestPermissions() method to request permissions and handle the user’s response in onRequestPermissionsResult().
42. Explain the concept of Fragments in Android?
Fragments are modular UI components in Android that represent a portion of a user interface. They are used to create flexible and reusable UI layouts that can be shared between activities and adapt to different screen sizes and orientations.
43. Explain the concept of content providers in Android?
Content providers are components in Android that provide a standard interface for sharing data between different apps. They allow apps to securely access and modify data from other apps or expose their data to other apps.
44. What is the purpose of the Uri in Android content providers?
The Uri (Uniform Resource Identifier) is used to uniquely identify a piece of data exposed by a content provider. It consists of a content scheme (content://), authority (package name of the app providing the data), and the data path.
45. What is the purpose of “lateinit” in Kotlin?
“lateinit” is used for properties that will be initialized later, usually in the constructor or a setup method.
46. How does “lazy” initialization work in Kotlin?
“lazy” is a function that delegates the initialization of a property to the first access. It’s only computed once and then cached for subsequent calls.
47. How do you create a singleton in Kotlin?
To create a singleton in Kotlin, you use the “object” keyword followed by the class name.
48. What is a sealed class in Kotlin?
A sealed class is a class that can be subclassed only within the same file where it is defined. It is often used for representing restricted class hierarchies.
49. How do you perform string interpolation in Kotlin?
In Kotlin, string interpolation is done by using the “$” symbol before the variable name in a string.
50. Explain the “init” block in Kotlin.
The “init” block in Kotlin is used to initialize properties or execute code during object creation, just like a constructor.