Kotlin String Interpolation : Format, Performance, Object Property
String manipulation is a fundamental part of programming, and Kotlin offers a variety of powerful tools to make this task more efficient and convenient. One such feature is Kotlin string interpolation, which allows developers to embed expressions directly within string literals.
What is String Interpolation?
String interpolation is a technique that enables developers to include variables, expressions, or statements within a string literal, making it easy to create dynamic strings without the need for concatenation or complex formatting.
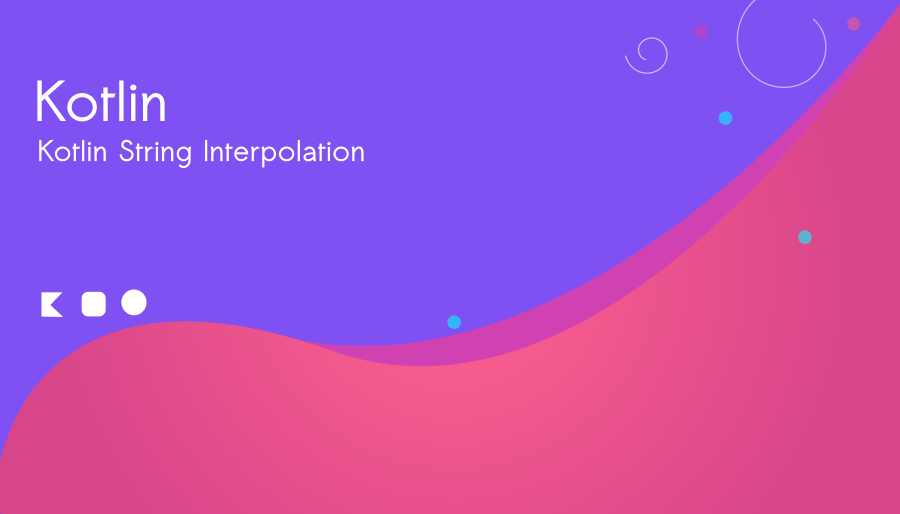
Kotlin provides a concise and intuitive syntax for string interpolation, making it a valuable tool for enhancing the readability and maintainability of your code.
Using String Interpolation in Kotlin
To utilize string interpolation in Kotlin, simply prefix your string literal with the ‘$’ symbol. Any variable or expression enclosed within curly braces ‘{ }’ will be evaluated and interpolated into the resulting string.
Interpolating Variables
fun main() {
val name = "Nikky"
val age = 45
val message = "My name is $name and I'm $age years old."
println(message)
}
Output
My name is Nikky and I’m 45 years old.
In the above example, the variables name and age are directly embedded within the string using string interpolation.
Evaluating Expressions
Open Kotlin Playground and Write the following program:-
fun main() {
val x = 67
val y = 566
val sum = "The sum of $x and $y is ${x + y}."
println(sum)
}
Output
The sum of 67 and 566 is 633.
By enclosing the expression ${x + y} within curly braces, we can evaluate and interpolate the sum of x and y into the resulting string.
Kotlin String Interpolation Formatting Options
Kotlin provides several formatting options to customize the appearance of interpolated values. Let’s explore some of the commonly used formatting techniques
Specifying Precision
When interpolating floating-point numbers, you can specify the desired precision using the format inline function.
fun main() {
val pi = 3.14159265359
val formattedPi = "The value of pi is approximately ${"%.2f".format(pi)}."
println(formattedPi)
}
Output
The value of pi is approximately 3.14.
Formatting Date and Time using String Interpolation
Kotlin allows you to format dates and times using the pattern letters from the java.time.format.DateTimeFormatter class
import java.time.LocalDate
import java.time.format.DateTimeFormatter
fun main() {
val today = LocalDate.now()
val formattedDate = "Today is ${today.format(DateTimeFormatter.ofPattern("yyyy-MM-dd"))}."
println(formattedDate)
}
Output
Today is 2023-07-08.
Escaping Characters
To include special characters like ‘$’ or ‘{‘ within the resulting string without triggering interpolation, you can escape them by using a backslash.
fun main() {
val price = 10.99
val formattedPrice = "The price is \$${price}."
println(formattedPrice)
}
Output
The price is $10.99.
The backslash ‘$’ prevents the ‘$’ symbol from being interpreted as the start of an interpolation expression.
How to call a function within string interpolation?
If you want to call a function within string interpolation in Kotlin, you can use the regular function call syntax within the curly braces ${}
fun loveKotlin(): String {
return "Hello, I love kotlin❤️"
}
fun main() {
val msg = "${loveKotlin()}"
println(msg)
}
Hello, I love kotlin❤️
You can apply the same concept to any function call within string interpolation. Just replace loveKotlin() with your desired function call, and the result will be interpolated into the string.
How to handle Null value in Interpolation?
It’s important to handle the null case properly to avoid any potential null pointer exceptions. Here’s an example that demonstrates how to handle null values within string interpolation.
Example
fun main() {
val name: String? = null
val age: Int? = 25
val message = "Name: ${name ?: "Unknown"}, Age: ${age ?: "Unknown"}"
println(message)
}
Name: Unknown, Age: 25
Kotlin : Interpolation vs Concatenation
In string interpolation, we directly embed variables or expressions within the string using the $ symbol and curly braces ${}.
In string concatenation, we use the + operator to combine multiple string literals and variables.
Example
fun main() {
val name = "Niky"
val age = 45
// String Interpolation
val interpolationMessage = "My name is $name and I'm $age years old."
println(interpolationMessage)
// String Concatenation
val concatenationMessage = "My name is " + name + " and I'm " + age + " years old."
println(concatenationMessage)
}
Output
My name is Niky and I’m 45 years old.
My name is Niky and I’m 45 years old.
Kotlin String Interpolation Object Property
In Kotlin, string interpolation allows you to directly access and interpolate object properties within a string.
data class Employee(val name: String, val tag_number: Int)
fun main() {
val emp = Employee("Nikin", 3893)
val message = "My name is ${emp.name} and My tag number is: ${emp.tag_number}"
println(message)
}
To interpolate the object properties within the string, we use the ${} syntax. In the message string, ${emp.name} is used to interpolate the value of the name property, and ${emp.tag_number} is used to interpolate the value of the tag_number property. The resulting message will be:
Output
My name is Nikin and My tag number is: 3893
Good thing about Kotlin Interpolation
One of the good things about Kotlin Interpolation is its ability to easily embed variables or expressions within strings, making string formatting more concise and readable.
Kotlin String Interpolation Performance
String interpolation in Kotlin generally offers good performance. Kotlin’s string interpolation is implemented efficiently and has been optimized to minimize unnecessary overhead.
When you use string interpolation, the compiler generates bytecode that efficiently combines the string literal and interpolated expressions at runtime. This process avoids unnecessary string concatenation operations that can be costly in terms of memory allocation and copying.