Kotlin lateinit : A guide to late initialization
Kotlin lateinit – a powerful keyword that allows delayed initialization of non-null properties. In this Kotlin tutorial, we will dive into the use of lateinit keyword and explore how it can simplify your code.
What is Kotlin’s lateinit?
lateinit is a Kotlin modifier that lets you mark a property as uninitialized when declared, but you promise the compiler that you’ll initialize it before accessing it. This is particularly useful when dealing with properties that can’t be assigned a value immediately, such as Android views, dependency-injected components, or other objects whose initialization takes place later in the lifecycle.
Instead of using nullable properties and initializing them as null, lateinit allows you to create non-nullable properties without an initial value, effectively deferring the initialization process to a later point in the code.
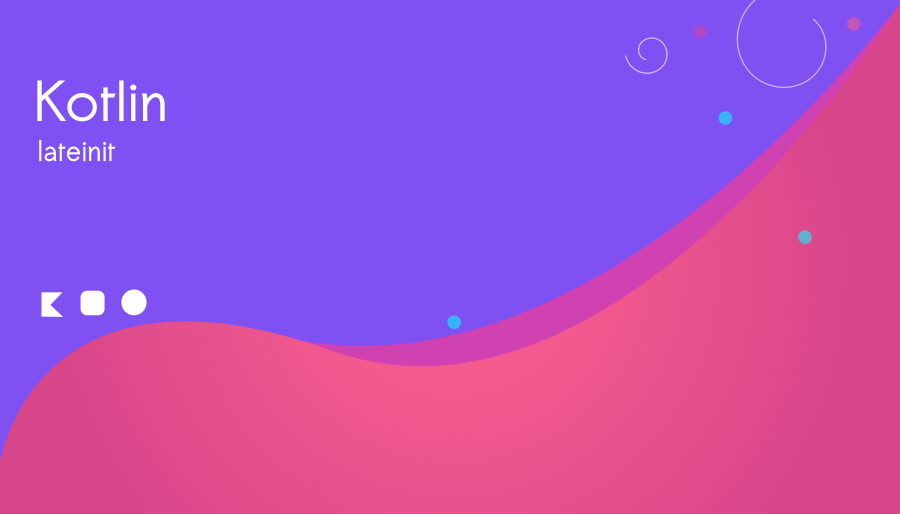
lateinit Syntax and Usage
The syntax for using lateinit is quite straightforward. When defining a property, you simply add the lateinit modifier before the property declaration, like this:
class SampleClass {
lateinit var sample: String
}
When to Use lateinit ?
Dependency Injection: When using dependency injection frameworks like Dagger or Hilt, components are typically injected into the class at runtime. By using lateinit, you can declare these component properties without immediate initialization.
Reducing Null Checks: Using lateinit eliminates the need for excessive null checks when declaring properties that must be initialized later. This helps in writing cleaner, more concise, and safer code.
lateinit example :
class Employee {
lateinit var name:String
fun showName(name: String)
{
this.name = name
}
}
fun main()
{
val employee = Employee()
employee.showName("Nikky SK")
println("Employee Name : ${employee.name}")
}
Output:
Employee Name : Nikky SK
Accessing the ‘name’ property before initialization (add this line after employee creation : println(employee.name)) would result in an error like below: ‘UninitializedPropertyAccessException‘
“Exception in thread “main” kotlin.UninitializedPropertyAccessException: lateinit property name has not been initialized”
In the main function, when we create a Employee instance, we cannot directly access the name property before initialization. Attempting to do so would result in an UninitializedPropertyAccessException.
Remember, it’s crucial to ensure that lateinit properties are initialized before being accessed, as failing to do so will result in runtime exceptions. Always exercise caution and only use lateinit when you are confident that the property will be initialized properly before being used.
In conclusion, lateinit is a powerful feature in Kotlin that simplifies the initialization process for non-null properties. It allows developers to declare properties without an initial value and defer the initialization to a later point in the code. However, with great power comes great responsibility. Always ensure that you initialize lateinit properties before accessing them to avoid runtime exceptions.