final in Dart : Variables That Can Be Assigned Once
In Dart, the ‘final’ keyword is utilized to declare variables that can only be assigned once. Reassigning a final variable is impossible, making it an ideal choice for declaring constants and variables meant to remain unchanged throughout the program. Let’s explore the use of final in dart language.
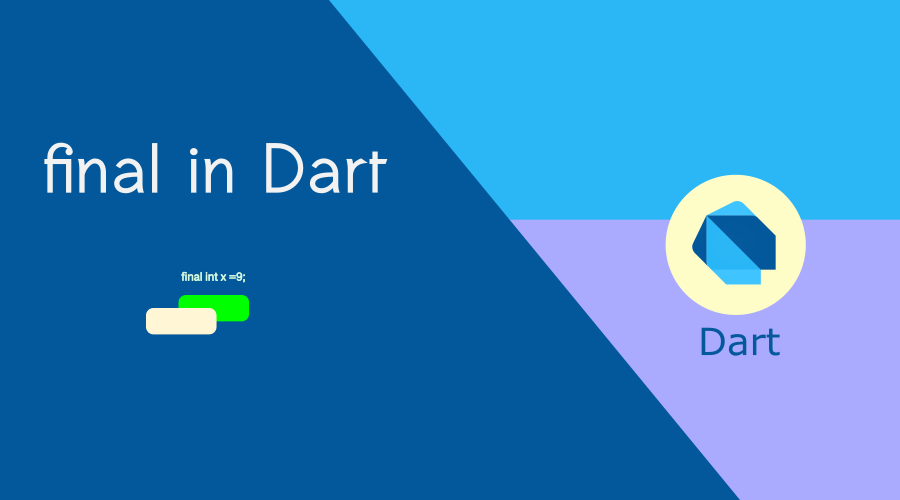
Open Dart pad and write the following program:
void main()
{
final int id = 900;
print(id); // prints 900
id = 90; // error
}
As demonstrated in the example, once a value is initially assigned to the variable ‘id,’ reassigning a new value becomes impossible.
If attempted, it will generate an error as shown below:
Error: Can’t assign to the final variable ‘id’.
id = 90;
We can add or remove items from a list that has already been declared as final, but reassigning is not possible. Let’s examine the following example.
final List<String> phones = ['pixel', 'iphone', 'galaxy'];
phones.add('moto'); // possible
phones.remove('iphone'); // possible
print(phones);
List<String> old_phones = ['nokia', 'htc', 'ibm'];
phones = old_phones; //error
Error: Can’t assign to the final variable ‘phones’.
phones = old_phones;
Final vs Const in Dart
The value of a final variable can be computed at runtime, whereas the ‘const‘ keyword is used when the variable must be known at compile time.
When to use final in Dart :
Use ‘final’ when the value needs to be computed at runtime or when the variable’s value might change during the execution of the program.
When to use const:
Utilize ‘const’ when the value is known at compile time and represents a constant expression. ‘const’ is commonly employed for literals and expressions involving only literals.
//Using final (Runtime)
final int time = DateTime.now().hour;
print(time);
//Using const
const int taskNumber = 34;
The use of ‘final‘ is particularly beneficial when you aim to guarantee that a variable or collection remains constant throughout the execution of your program.
Handling final in Class
In Dart, when ‘final’ is employed within the context of a class, it can be applied to fields, signifying that the value of the field can only be assigned once.
class Demo {
final int id;
Demo(int value): id = value{
}
void showValue() => 'Constant value $id';
}
Verdict
In essence, opt for ‘final’ when the value can be computed at runtime, and choose ‘const’ when the value is known at compile time and constitutes a constant expression. While both facilitate the declaration of constants, ‘const’ is more restrictive and frequently results in enhanced performance optimizations by the Dart compiler.