Say Hello to Flutter Row Widget
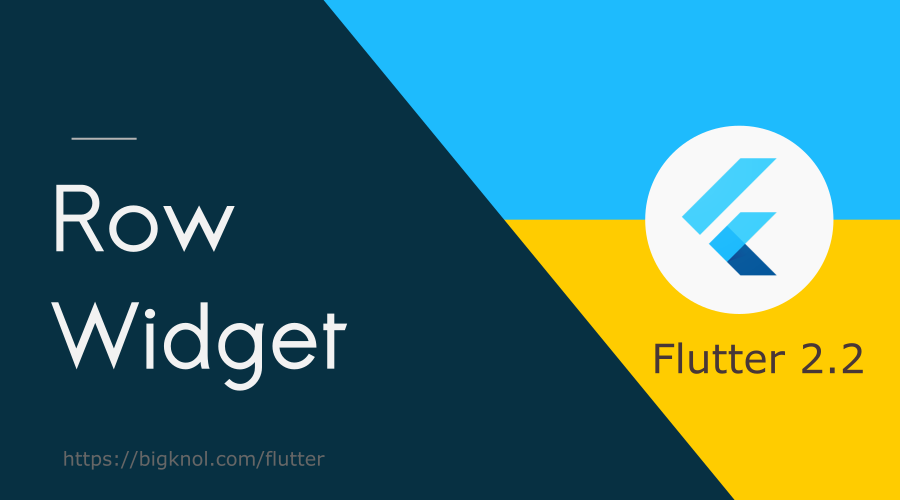
The Row widget is one of the fruitful widgets in flutter app development.
It can accommodate other widgets in a horizontal array fashion. The flutter row widget shows its children in a horizontal array.
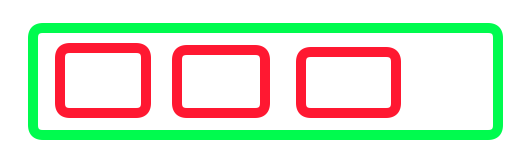
The Column and Row widgets are indispensable for making an app using Flutter.

The Row widgets do not scroll. Consider ListView instead of a Row widget.
Let’s start with a Hello, World starter code.
Let’s add some Text() as children in the Row widget.
Row(
children: [
Text("I like Java."),
Text("I like Python."),
Text("I like Lua."),
Text("I like Cobol."),
Text("I like Kotlin."),
],
),
main.dart
import 'package:flutter/material.dart';
void main()
{
runApp(MyRowApp());
}
class MyRowApp extends StatelessWidget
{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Row App"),),
body: Row(
children: [
Text("I like Java."),
Text("I like Python."),
Text("I like Lua."),
Text("I like Cobol."),
Text("I like Kotlin."),
],
),
),
);
}
}
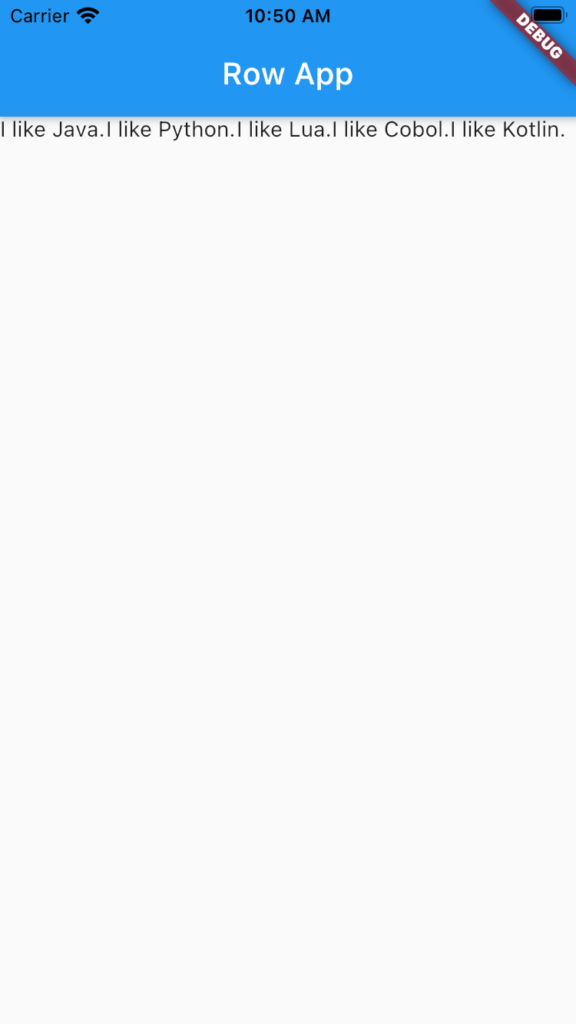
I like to add another Text() to the Row widget. which will be Text(“I like Go”)
import 'package:flutter/material.dart';
void main()
{
runApp(MyRowApp());
}
class MyRowApp extends StatelessWidget
{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Row App"),),
body: Row(
children: [
Text("I like Java."),
Text("I like Python."),
Text("I like Lua."),
Text("I like Cobol."),
Text("I like Kotlin."),
Text("I like Go")
],
),
),
);
}
}
Yes, We have a problem with the Flutter Row widget
In Row philosophy, it is considered an error to have more children in a Row if there no room for a new item.
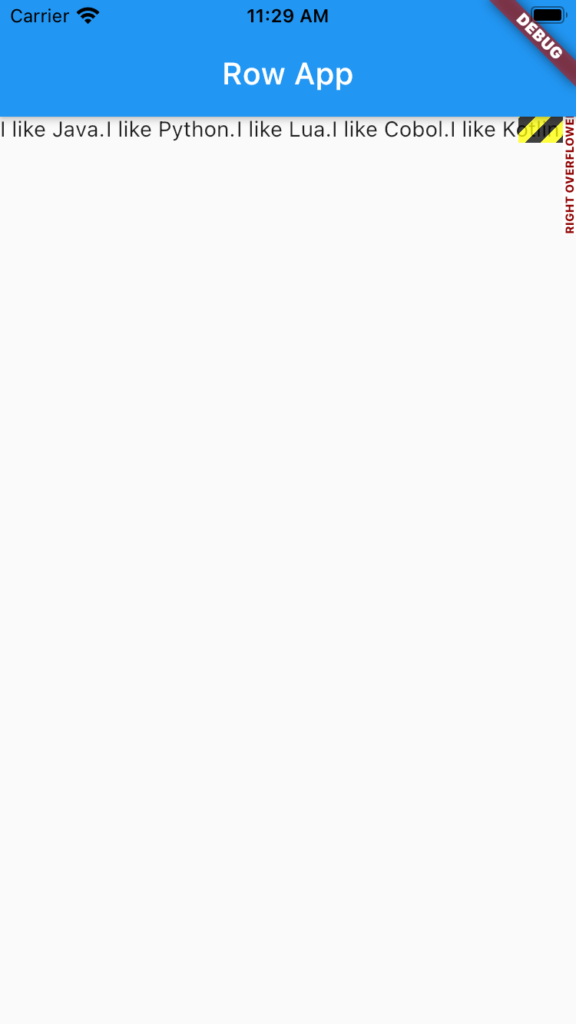
If you observe the error
Consider applying a flex factor (e.g. using an Expanded widget) to force the children of the RenderFlex to fit within the available space instead of being sized to their natural size.
This is considered an error condition because it indicates that there is content that cannot be seen. If the content is legitimately bigger than the available space, consider clipping it with a ClipRect widget before putting it in the flex, or using a scrollable container rather than a Flex, like a ListView.
Let’s use Expanded widget
import 'package:flutter/material.dart';
void main()
{
runApp(MyRowApp());
}
class MyRowApp extends StatelessWidget
{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Row App"),),
body: Row(
children: [
Expanded(child: Text("I like Java.")),
Expanded(child: Text("I like Python.")),
Expanded(child: Text("I like Lua.")),
Expanded(child: Text("I like Cobol.")),
Expanded(child: Text("I like Kotlin.")),
Expanded(child: Text("I like Go")),
],
),
),
);
}
}
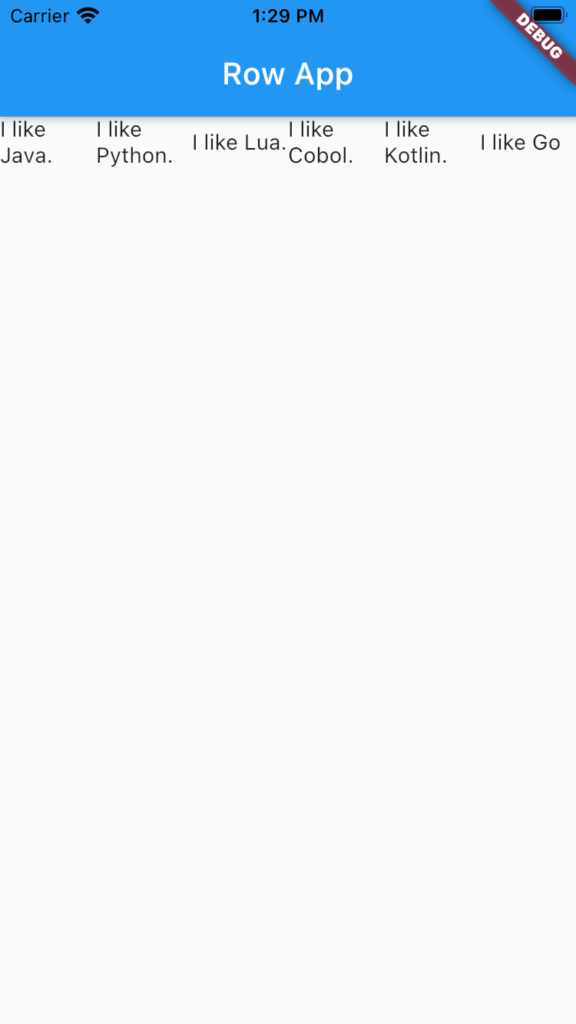
crossAxisAlignment and mainAxisAlignment
import 'package:flutter/material.dart';
void main()
{
runApp(MyRowApp());
}
class MyRowApp extends StatelessWidget
{
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Row App"),),
body: Row(
crossAxisAlignment: CrossAxisAlignment.end,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Expanded(child: Text("I like Java.")),
Expanded(child: Text("I like Python.")),
Expanded(child: Text("I like Lua.")),
Expanded(child: Text("I like Cobol.")),
Expanded(child: Text("I like Kotlin.")),
Expanded(child: Text("I like Go")),
],
),
),
);
}
}