Kotlin List Tutorial : Create, Iterate and Apply Filters
One of Kotlin’s most powerful features is its support for collections, including the versatile Kotlin List.
What is a Kotlin List?
In Kotlin, a List is an ordered collection that can contain duplicate elements. It is a read-only interface that provides an array-like structure and offers numerous utility functions to manipulate and retrieve data. Lists in Kotlin are mutable (modifiable) or immutable (read-only), depending on the type of List implementation used.
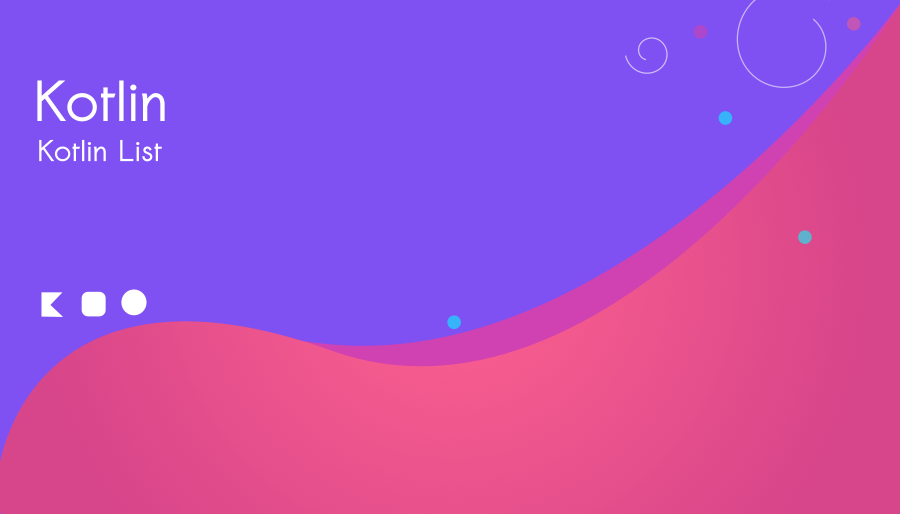
How to create a List ?
Let’s create an immutable list using listOf()
val vegetable = listOf("Cabbage","Tomato","Bitter Gourd")
listOf():
fun main()
{
val vegetable = listOf("Cabbage","Tomato","Bitter Gourd")
println(vegetable)
}
Output
[Cabbage, Tomato, Bitter Gourd]
Iterate immutable list using for loop:
fun main()
{
val vegetable = listOf("Cabbage","Tomato","Bitter Gourd")
for (veg in vegetable)
{
println(veg)
}
}
Output
Cabbage
Tomato
Bitter Gourd
Iterate immutable list using forEach:
fun main()
{
val vegetable = listOf("Cabbage","Tomato","Bitter Gourd")
vegetable.forEach {
veg -> println(veg)
}
}
Print all elements using joinToString() function:
Open Kotlin Play ground and write the following code:
fun main()
{
val vegetable = listOf("Cabbage","Tomato","Bitter Gourd")
println(vegetable.joinToString())
}
Output
Cabbage, Tomato, Bitter Gourd
How to access the first and last elements of an immutable list?
fun main()
{
val vegetable = listOf("Cabbage","Tomato","Spinach","Bitter Gourd")
//access the last element
val lastItem = vegetable.last()
println("Last item : $lastItem")
// access the element at first
val firstItem = vegetable.first()
println("First item : $firstItem")
}
Output:
Last item : Bitter Gourd
First item : Cabbage
Is there any way to access the middle item?
// access the middle item
val size = vegetable.size / 2
val middleItem = vegetable[size]
println("Middle item: $middleItem")
Output
Middle item: Spinach
How to apply filter on immutable list ?
fun main()
{
val vegetable = listOf("Cabbage","Tomato","Bitter Gourd")
val newVeg = vegetable.filter { it == "Tomato" }
println(newVeg) // prints [Tomato]
}
Let’s create a mutable list using mutableListOf():
var os = mutableListOf("Android", "iOS", "Linux")
Add elements to a mutable list using add, addAll():
fun main()
{
var os = mutableListOf("Android", "iOS", "Linux")
os.add("Solaris") //adds a single element
println(os)
}
Output
[Android, iOS, Linux, Solaris]
Add multiple items to a mutable list using addAll():
os.addAll(listOf("tvOS", "iPadOS","Tizen"))
println(os)
[Android, iOS, Linux, Solaris, tvOS, iPadOS, Tizen]
How to access the last and first elements of a mutable list ?
//access the first element
val first = os.first()
println("First : $first")
//access the last element
val last = os.last()
println("Last : $last")
First : Android
Last : Linux
Remove a single item from a mutable list
fun main()
{
var os = mutableListOf("Android", "iOS", "Linux")
//remove a single item
os.remove("Android")
println(os)
}
[iOS, Linux]
Remove item at a specific position:
//remove item at a specific position
os.removeAt(1)
println(os)
[iOS]
removeAll()
fun main()
{
var os = mutableListOf("Android", "iOS", "Linux")
os.removeAll(listOf("Android", "iOS"))
println(os)
}
[Linux]
Let’s iterate using for and forEach :
for (name in os)
{
println(name)
}
os.forEach{
item ->
println(item)
}
If you don’t need to modify the list after creation, use immutable lists (e.g., listOf) as they offer better performance and immutability guarantees.
Kotlin Lists are an indispensable tool for every Kotlin developer. Their versatility, combined with Kotlin’s expressive syntax, empowers developers to handle collections of data effectively. By understanding the common operations and adopting best practices, you can leverage the full potential of Kotlin Lists in your projects, resulting in more maintainable and efficient code.
Kotlin Lists maintain the order of elements as they are inserted, providing a predictable sequence. This ensures that elements are retrieved in the same order as they were added, which is crucial for scenarios where element order matters.