Dart Enum : Enumerated Dart types Examples
Enum is a powerful feature in Dart that allows developers to define a set of named constants. Dart Enum provides a way to define a set of predefined values.
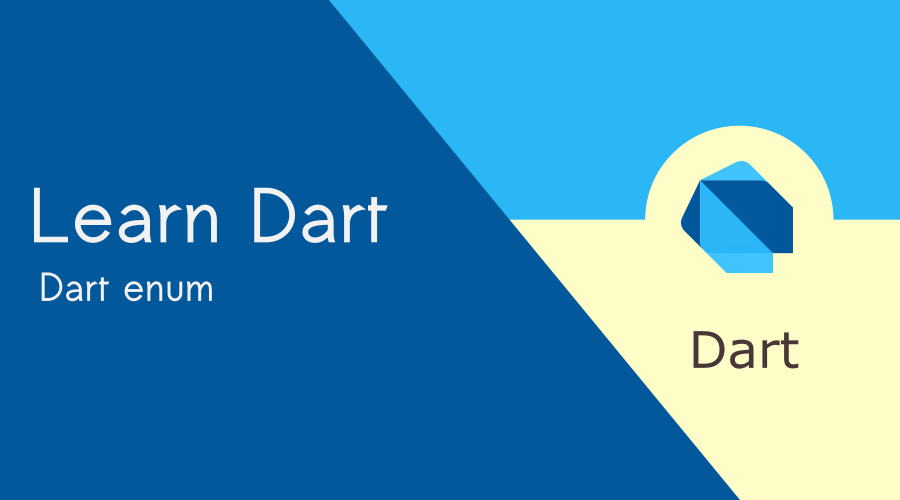
We will explore the concept of enum in Dart, its benefits, and how it simplifies code by providing a structured way to represent a fixed set of values.
An enum in Dart is a special data type that represents a collection of related named constants, also known as enumerations.
Enums provide a way to define a set of predefined values that can be used throughout your code. Each value in an enum is called an enum constant.
To define an enum in Dart, you use the enum keyword followed by the name of the enum and a list of constant values enclosed in curly braces.
enum Color {
red,
blue,
green,
yellow,
}
In this example, we have defined an enum called Color with four constants: red, blue, green, and yellow.
Defining simple enums
Dart Enum Switch Example :
enum Day {
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday,
Sunday,
}
void main() {
Day today = Day.Tuesday;
if (today == Day.Saturday || today == Day.Sunday) {
print("It's the weekend!");
} else {
print("It's a weekday.");
}
switch (today) {
case Day.Monday:
print("Today is Monday");
break;
case Day.Tuesday:
print("Today is Tuesday");
break;
case Day.Wednesday:
print("Today is Wednesday");
break;
case Day.Thursday:
print("Today is Thursday");
break;
case Day.Friday:
print("Today is Friday");
break;
case Day.Saturday:
print("Today is Saturday");
break;
case Day.Sunday:
print("Today is Sunday");
break;
}
}
It's a weekday.
Today is Tuesday
n this example, we have defined an enum called Day representing the days of the week. We have defined seven constants within the enum: Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, and Sunday.
In the main function, we assign the value Day.Tuesday to the variable today. Then, we use an if statement to check if today is a weekend day (Saturday or Sunday) and print the corresponding message.
Next, we use a switch statement to print the name of the day based on the value of today. Depending on the value of today, the corresponding case will be executed.
Dart enum with string values
In Dart, enums can also have string values associated with each constant. Here’s an example of how to define an enum with string values:
Open the DartPad and Write the following code:
enum Fruit {
apple,
banana,
orange,
}
void main() {
Fruit favoriteFruit = Fruit.banana;
switch (favoriteFruit) {
case Fruit.apple:
print("I love apples!");
break;
case Fruit.banana:
print("I enjoy bananas!");
break;
case Fruit.orange:
print("Oranges are delicious!");
break;
}
}
I enjoy bananas!
How to Convert Dart enum to String
You can convert an enum value to a string using the toString() method. The toString() method is automatically provided by Dart for all objects, including enum values.
enum Fruit {
apple,
banana,
orange,
}
void main() {
Fruit myFruit = Fruit.banana;
String fruitString = myFruit.toString();
print(fruitString); // Prints "Fruit.banana"
}
In the above example, we have an enum called Fruit with three constants: apple, banana, and orange. We create an instance of the Fruit enum and assign Fruit.banana to the variable myFruit.
To convert the myFruit enum value to a string, we call the toString() method on the myFruit object. The toString() method returns a string representation of the enum value.
Fruit.banana
Dart enum Extension Example
enum Fruit {
apple,
banana,
orange,
}
extension FruitExtension on Fruit {
String get stringValue {
return this.toString().split('.').last;
}
}
void main() {
Fruit myFruit = Fruit.banana;
String fruitString = myFruit.stringValue;
print(fruitString); // Prints "banana"
}
In this example, we define an enum called Fruit with three constants: apple, banana, and orange.
Next, we create an extension called FruitExtension on the Fruit enum. Inside the extension, we define a getter called stringValue that returns the string representation of the enum value.
The getter uses the toString() method and then splits the resulting string by the . character to extract just the last part, which represents the enum constant name.
In the main function, we create an instance of the Fruit enum and assign Fruit.banana to the myFruit variable.
Finally, we call the stringValue getter on the myFruit object to get the string representation of the enum value, and print it to the console.
banana
By creating an extension for the enum, we can easily add additional functionality to it. In this case, the extension allows us to conveniently retrieve the string representation of the enum value using the stringValue getter.
enum index property
You can get the index value (as an integer) of an enum constant by using the index property of the enum.
enum Fruit {
apple,
banana,
orange,
}
void main() {
Fruit myFruit = Fruit.banana;
int fruitIndex = myFruit.index;
print(fruitIndex); // Prints 1
}
In this case, since Fruit.banana is the second constant in the Fruit enum, its index value is 1.
1
By using the index property, you can easily obtain the integer value corresponding to an enum constant in Dart.
Dart enum inheritance support
In Dart, enums do not support inheritance directly. Enumerations are a special kind of data type that represents a set of related named constants, and they cannot be extended or inherited from.
However, if you want to define related sets of constants with similar behavior or properties, you can use classes and inheritance instead. Here’s an example that demonstrates how to achieve similar functionality using classes
abstract class Fruit {
void eat();
}
class Apple implements Fruit {
@override
void eat() {
print('Eating an apple.');
}
}
class Banana implements Fruit {
@override
void eat() {
print('Eating a banana.');
}
}
void main() {
Fruit apple = Apple();
apple.eat(); // Prints 'Eating an apple.'
Fruit banana = Banana();
banana.eat(); // Prints 'Eating a banana.'
}
By using classes and interfaces, we can achieve similar functionality to enum inheritance, where each class represents a specific type of fruit with its own behaviour.
Dart enum naming convention
In Dart, the naming convention for enums follows the same guidelines as other identifiers in the language.
PascalCase
Enum type names should be written in PascalCase, which means the first letter of each word should be capitalized, including the first word.
For example: Color, DayOfWeek, LogLevel.
Singular nouns
Enum type names should typically use singular nouns to represent a single value from the enumeration. For
example: Color, Day, LogLevel.
enum Color {
RED,
GREEN,
BLUE,
}