Function Overloading in Dart : Code Reusability
Function overloading is a powerful concept in programming that allows developers to define multiple functions with the same name but different parameters. Let’s explore function overloading in Dart through a practical examples.
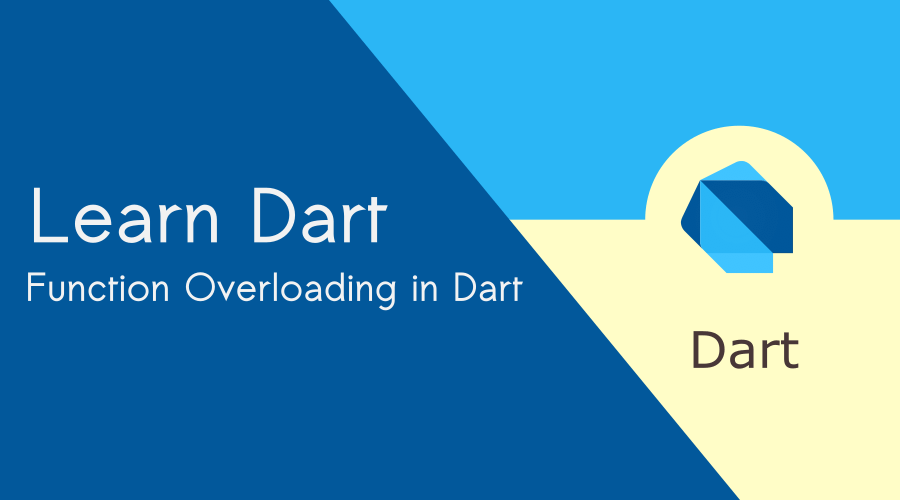
We will explore function overloading in Dart, its benefits, and how it enables code reusability and flexibility in building robust and scalable applications.
What is Function Overloading?
Function overloading refers to the ability to define multiple functions with the same name but different parameter lists. In Dart, however, unlike some other programming languages, true function overloading is not supported. Dart uses named and optional parameters to achieve similar functionality.
Dart allows you to define a single function with optional parameters or named parameters, providing flexibility in how the function can be called. Optional parameters are denoted by enclosing them in square brackets ([]), while named parameters are denoted by enclosing them in curly braces ({}).
Benefits of Function Overloading
Code Reusability
Function overloading, or its equivalent in Dart, allows developers to reuse function names and provide different behaviours based on the parameters passed. This promotes code reusability and reduces code duplication.
Adapting to Different Input Types
Function overloading allows developers to handle different input types and adapt the behaviour of a function accordingly. This is particularly useful when dealing with diverse data types and varying requirements.
Function Overloading in Dart : Example
Open DartPad and write the following code:
void main() {
greet("Nikin");
greet("Nikin", "Good morning");
}
void greet(String name, [String? message]) {
if (message != null) {
print("$message, $name!");
} else {
print("Hello, $name!");
}
}
Output
Hello, Nikin!
Good morning, Nikin!
In the main function, we call the greet function twice, passing different arguments.
The first call to greet(“Nikin”) provides only the required parameter name, which is set to “Nikin”. It does not provide the optional parameter message.
The second call to greet(“Nikin”, “Good morning”) provides both the required parameter name and the optional parameter message. The value of name is set to “Nikin”, and the value of message is set to “Good morning”.
The greet function is defined after the main function. It takes two parameters: name of type String and message of type String? (optional string).
Inside the greet function, there is a conditional check using an if-else statement. It checks if the message parameter is not null.
If the message parameter is not null, it prints the interpolated string $message, $name!, which combines the provided message and name.
If the message parameter is null, it prints the default greeting string “Hello, $name!”.
Since the message parameter is optional, if it is not provided when calling the greet function, it defaults to null.
While Dart does not directly support traditional function overloading, it provides the flexibility of named and optional parameters to achieve similar functionality.