Inline Functions in Kotlin : Optimizing the Code Performance
Koltin, known for its concise syntax and powerful features, introduces the concept of inline functions. Let’s dive into the intricacies of inline functions in Kotlin and their influence on code efficiency.
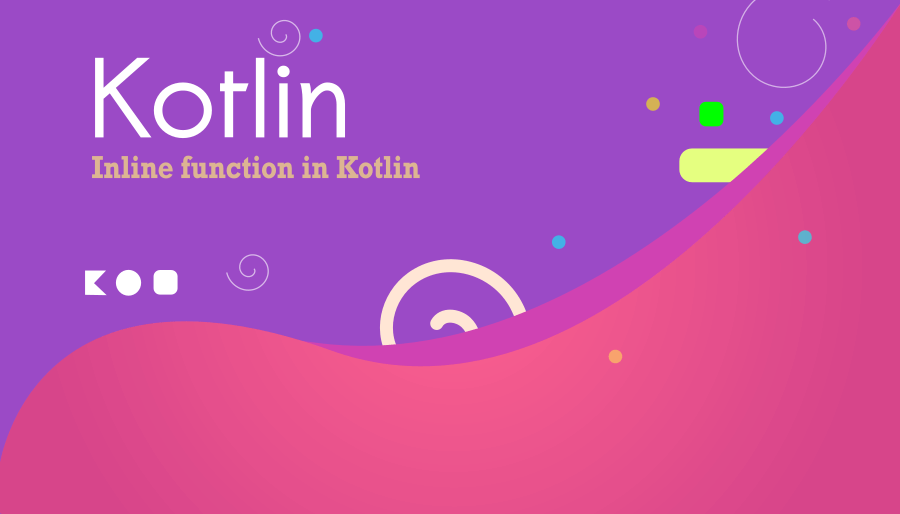
Introduction to Inline Functions in Kotlin
An inline function in Kotlin is a function that, upon invocation, substitutes its code directly into the call site. This substitution eliminates the overhead of function calls, thereby improving performance. By using the inline keyword before function declaration, developers can instruct the compiler to inline the function’s code where it is called.
Syntax and Usage of Inline Functions
Declaring an inline function in Kotlin is straightforward.
Open the Kotlin Playground and Write the following code:
fun main() {
val result = sum(6,7)
println(result)
}
inline fun sum(a: Int, b: Int) : Int {
return a+b
}
You may get a warning message like below:
‘Expected performance impact from inlining is insignificant. Inlining works best for functions with parameters of functional types‘
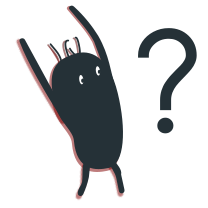
The warning is highlighting that the performance gain from inlining might not be significant in all cases. Inlining tends to be most effective when used with functions that have parameters of functional types like lambdas or function references.
// Regular higher-order function that takes a lambda as a parameter
fun higherOrderFunction(lambda: (Int) -> Int): Int {
return lambda.invoke(5) // Invoking the lambda with a value of 5
}
// Inline function that takes a lambda as a parameter
inline fun inlineHigherOrderFunction(lambda: (Int) -> Int): Int {
return lambda(5) // Directly invoking the lambda with a value of 5 (no function call overhead)
}
fun main() {
val regularResult = higherOrderFunction { value -> value * 2 }
println("Result of regular higher-order function: $regularResult")
val inlineResult = inlineHigherOrderFunction { value -> value * 2 }
println("Result of inline higher-order function: $inlineResult")
}
Result of regular higher-order function: 10
Result of inline higher-order function: 10
In this example, both higherOrderFunction and inlineHigherOrderFunction perform the same task: they take a lambda that doubles an integer and apply it to the value 5. However, the inlineHigherOrderFunction is marked as inline, allowing the lambda to be directly substituted at the call site. This avoids the function call overhead, potentially improving performance in scenarios where such optimizations matter.
Advantages of Using Inline Functions
The utility of inline functions extends beyond mere performance enhancement. Furthermore, when used in conjunction with higher-order functions, inline functions prevent unnecessary object creation and memory allocation, improving overall efficiency.
Moreover, the utilization of inline functions with lambdas contributes to improved code readability without compromising performance. Inlining lambda bodies at the call site reduces function call overhead and enhances execution efficiency.
Understanding Control Over Inlining Behavior
While inline functions offer significant performance benefits, not all functions should be marked as inline. Additionally, indiscriminate inlining may result in code bloat, adversely affecting application performance. Developers must judiciously use the inline keyword to balance performance optimization and code maintainability.
The Role of ‘noinline’ Modifier
In scenarios where selective inlining is necessary, Kotlin provides the noinline modifier. This modifier prevents specific lambdas within higher-order functions from being inlined. It allows developers to optimize only certain parts of the code while maintaining the overall structure and clarity.
Inline Functions and Reified Type Parameters
Another compelling aspect of inline functions in Kotlin is their interaction with reified type parameters. Marking a function as inline enables it to access type information at runtime through reified type parameters. This feature ensures compile-time safety while allowing runtime type checks and casts within the function body.
Conclusion
However, it’s essential to strike a balance between code optimization and maintainability. Overusing the inline keyword may lead to code duplication and larger executable sizes, potentially negating the performance benefits.
In conclusion, inline functions in Kotlin are a powerful tool for optimizing code performance, especially in scenarios involving higher-order functions and lambdas. By judiciously incorporating inline functions, developers can strike a balance between efficient execution and code readability.