Kotlin Constants : const and val keywords in Kotlin Code
In this tutorial, we will explore the fundamentals of Kotlin constants. Having already learned about Kotlin variables, let’s now dive into how Kotlin, a modern and concise programming language, provides a straightforward way to declare and utilize constants in your code.
First of all, let’s understand the importance of constants. Constants are values that remain unchanged throughout the program’s execution. Declaring them enhances code readability and prevents accidental modifications, promoting code stability.
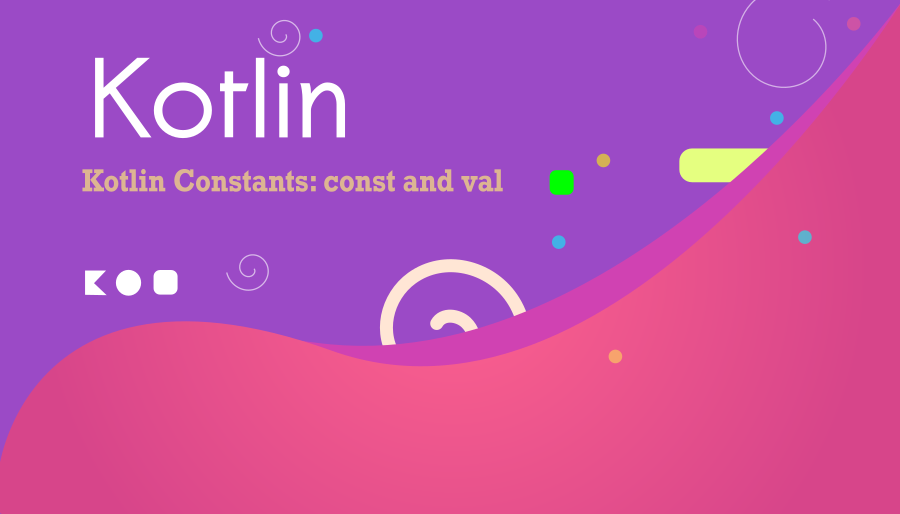
Declaring Kotlin Constants
In Kotlin, constants are declared using the `val` keyword. This keyword is following by the constant’s name, a colon, the data type, and finally the assigned value. Here’s a simple example:
val PI: Double = 3.14
All read-only variables are declared using the val keyword. JetBrains, the developer of Kotlin, recommends declaring all variables as read-only (val) by default.
Immutability and Kotlin Constants
Kotlin constants, being immutable, do not allow modifications to their values once assigned. This immutability ensures that the declared constant remains constant throughout the program’s execution.
Similarly, when working with complex data types, such as objects or collections, Kotlin constants maintain their immutability.
Type Inference in Kotlin
Kotlin supports type inference, allowing you to omit the explicit type declaration when the compiler can infer it. This makes the code more concise without sacrificing clarity.
val appName = "KotlinApp"
// The compiler infers the type as String based on the assigned value.
However, you can still use explicit type declarations when clarity is crucial or when the compiler may not unambiguously infer the type.
const keyword in Kotlin
The const keyword in Kotlin primarily serves for compile-time constants, and it comes with specific requirements and limitations:
Top-level or Member of an Object
You can use the const keyword for properties that are top-level or members of objects (including companion objects). However, you cannot use it for properties inside functions, constructors, or any other places.
const val MIN_VALUE = 100
fun main() {
val kotlin = "🙂"
println(kotlin)
println(MIN_VALUE)
}
🙂
100
Types and String
The value being assigned using const must be a compile-time constant. This means it should be a data type (like Int, Long, Double, etc.) or a String.
const val ERROR = "Page not found"
fun main() {
println(ERROR)
}
Page not found
No Custom Getters
The property with the const keyword cannot have a custom getter. Implicitly functioning as a constant, its value is determined at compile time.
const val AREA = calculateArea(radius) // Invalid
Keep in mind that the const keyword is more restrictive than the regular val keyword. It is evaluated at compile time, and its usage is limited.
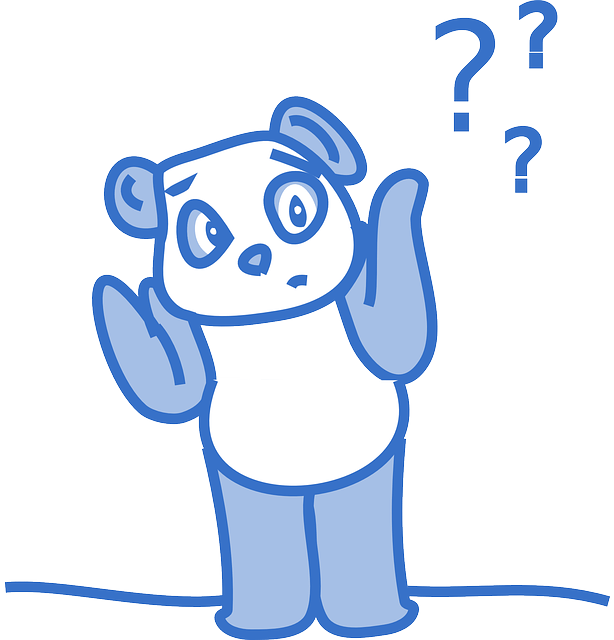
final keyword alternative in Kotlin?
While Kotlin does not have a direct equivalent to the final keyword, the language’s emphasis on immutability through val and other features encourages writing robust and predictable code. Using val appropriately helps achieve similar benefits to what final provides in Java.
What about the Global scope?
In Kotlin, you can use the val keyword at the top level of your file to declare constants that are effectively global in scope within that file.
// Constants.kt
val PI = 3.14
val APP_NAME = "MD pro"
fun main() {
println("PI: $PI")
println("App name: $APP_NAME")
}
In this example, PI and APP_NAME are constants declared at the top level of the file, making them accessible throughout the entire file.
You can then use these constants in functions, classes or any other code within the same file.
Keep in mind that the scope of these constants is limited to the file in which they are declared. If you want to share constants across multiple files, you can create a separate Kotlin file dedicated to constants and import them where needed. For instance, you might have a Constants.kt file with all your global constants, and then import them in other files:
Constants.kt
// Constants.kt
val MAX = 100
val PI = 3.14
val APP_NAME = "MD pro"
User.kt
// User.kt
import Constants.MAX
import Constants.PI
import Constants.APP_NAME
fun main() {
println("Max: $MAX")
println("PI: $PI")
println("App name: $APP_NAME")
}
Let’s summarize the key points covered in this tutorial:
- Kotlin constants, declared with val, provide a way to represent unchanging values.
- Immutability ensures that the assigned values of constants remain constant throughout the program.
- Kotlin constants are not confined to the global scope; they can be used within functions and other code blocks.
- The const keyword in Kotlin is primarily used for compile-time constants.