Kotlin Extension Functions : Usage and Examples
Kotlin language has many fruitful features for better coding and development, and one of the coolest features is Kotlin extension functions.
Understanding Kotlin Extension Functions
In Kotlin, extension functions let developers add new abilities to existing classes without changing their original code. This means you can enhance the behavior of any class, even ones from external libraries or standard Kotlin classes. By doing this, you can create clearer and more organized code, avoiding the need for large and cluttered utility classes.
Defining an Extension Function
Creating an extension function is straightforward. To do so, define a new function outside the target class, preceded by the target class name with a dot. The first parameter of the extension function is always the target class instance, accessed using the this keyword.
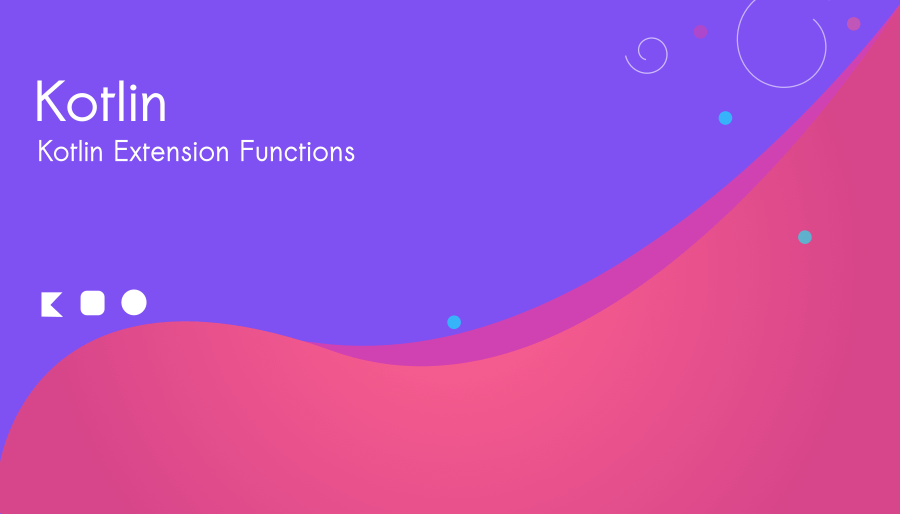
A simple example
Let’s create an extension function as below:
fun String.capConverter(): String{
return this.uppercase()
}
val name = "nikisurf".capConverter()
Complete code:
fun main(){
val name = "nikisurf".capConverter()
println(name)
}
fun String.capConverter(): String{
return this.uppercase()
}
NIKISURF
Usage of Kotlin’s Extension functions
Once you have defined an extension function, using it is as simple as calling any regular method on the target class instance. This not only makes the code more readable but also reduces the number of utility functions scattered across the project.
The primary advantage of extension functions is their ability to enhance code readability and organization. By creating concise, domain-specific extension functions, developers can encapsulate complex logic related to a particular class. As a result, the main codebase remains focused and comprehensible.
Consider the following example, where we create an extension function to calculate the total price of a list of products:
package extensions
fun main(){
val products = listOf(Product("iPhone",67000.00),
Product("iPad", 45000.00)
)
val total = products.calculateTotal()
print(total)
}
fun List<Product>.calculateTotal():Double {
var totalPrice = 0.0
for (product in this){
totalPrice += product.price
}
return totalPrice
}
data class Product(val item:String, val price:Double)
Output
112000.0
Code Reusability of extension functions
Suppose you often find yourself needing to check if a String is a valid email address. Instead of rewriting the same validation logic everywhere, create a simple extension function.
fun main() {
val email = "dsErd#@ds.com"
val check = if(email.isValidEmail()) "Valid!" else "Invalid"
println(check)
}
fun String.isValidEmail(): Boolean
{
val emailPattern = Regex("^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,6}$")
return emailPattern.matches(this)
}
Invalid
Now, you can effortlessly use this extension function to validate email addresses wherever needed.
By using extension functions, you can write more expressive, organized, and reusable code. These concise, domain-specific functions not only enhance the readability of your codebase but also streamline your development process.
Kotlin extension function for formatting date
Open Kotlin Playground and write the following program:
import java.text.SimpleDateFormat
import java.util.Date
import java.util.Locale
fun main() {
val currentDate = Date()
val formattedDate = currentDate.formatDate()
println("Formatted Date: $formattedDate")
}
fun Date.formatDate(): String {
val formatter = SimpleDateFormat("dd MMMM yyyy", Locale.getDefault())
return formatter.format(this)
}
Formatted Date: 01 August 2023