AdMob Splash Banner: How to Show an Ad on Android Splash Screen
In the world of making mobile apps, making money is really important to keep your app going. One way to make money is by showing ads in your app. AdMob is a famous choice for putting ads in Android and iPhone apps. This guide will help you put an AdMob banner ad on your app’s splash screen. The AdMob SDK doesn’t offer any native AdMob splash banner ad, so we will place an adaptive banner ad on your app’s splash screen. Let’s create an Android app for this implementation.
Prerequisites for AdMob Splash Ad
Before we dive into the integration process, make sure you have the following prerequisites in place:
1. Android Studio : You’ll need the appropriate development environment.
2. AdMob Account (Optional) : You can use test banner unit ID and App ID for development.
3. Developed App (Optional): You should have an existing app with a splash screen where you want to display the banner ad.
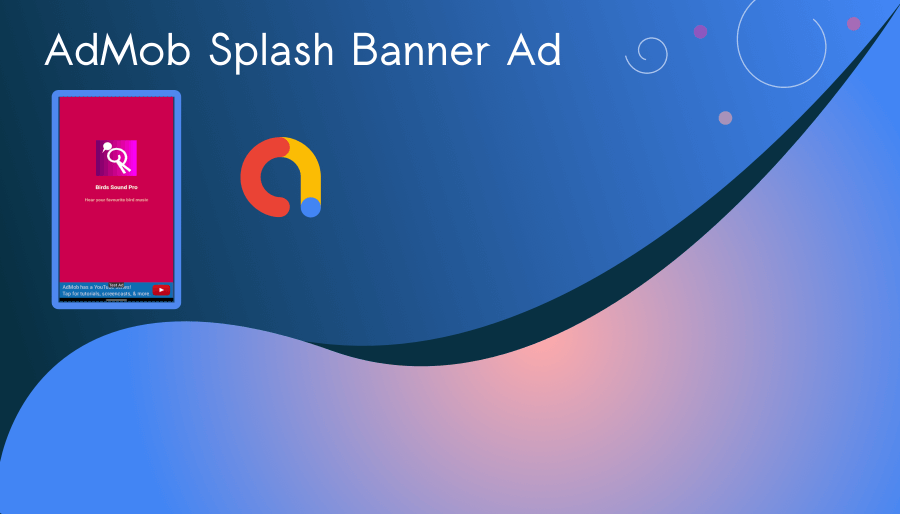
How to implement AdMob Splash Banner ?
1. At present, Splash Banner ads can only be requested through both AdMob and AdManager from a Banner Ad Unit.
2. Publishers should aim to keep users on the Splash Loading screen of their app for no longer than 5 seconds (with a recommended duration of 3-5 seconds). This provides ample time for users to view the Banner ad and take action if desired.
3. Splash banner can be anchored to either top or bottom of the screen.
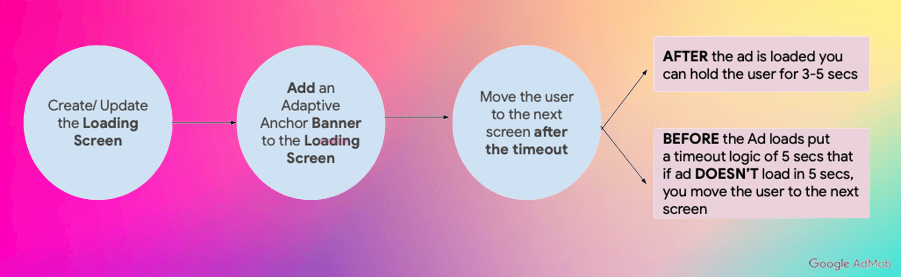
Let’s build an Android app and Add the following:
Add the following dependency to build.gradle(:app)
implementation 'com.google.android.gms:play-services-ads:22.1.0'
Include the App Id inside the application tag of the Android Manifest File.
<meta-data
android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="ca-app-pub-xxxxx" />
Please replace the ca-app-pub-xxxxx with your own App Id or Test Id for debugging.
Test IDs Table
App Id (Testing) – Test ID | ca-app-pub-3940256099942544~3347511713 |
Adaptive Banner Id (Testing) | ca-app-pub-3940256099942544/9214589741 |
Banner Id (Testing) | ca-app-pub-3940256099942544/6300978111 |
You can find many other ids on official Google AdMob doc.
If you are using a real device for testing use the following code inside your Splash Activity.
// Test your Ad
MobileAds.setRequestConfiguration(new RequestConfiguration.Builder().setTestDeviceIds(Arrays.asList("ABCDEF012345"))
.build());
// Testing ends
Note: Replace ‘ABCDEF012345’ this with your own Device ID.
Create a new Full Screen Activity for your Splash Screen
Create a new Android Activity ‘SplashScreen‘.
Create a full screen theme for our Splash Screen.
Add the following to the theme.xml file :
<style name="Theme.Slideimage.FullScreen">
<item name="windowActionBar">false</item>
<item name="windowNoTitle">true</item>
</style>
Theme.Slideimage will be your Base Theme (Change it according to your project)
Open the Android Manifest file add this theme to SplashScreen.
<activity
android:theme="@style/Theme.Slideimage.FullScreen"
android:name="com.bigknol.SplashScreen"
android:exported="true" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
Create another Activity called ‘RainWords’. We will invoke the RainWords activity from the Splash screen.
Let’s define a timeout for SplashScreen.
Decorate the Splash Screen
Add the ad container (fragment) to activity_splash_screen.xml
<FrameLayout
android:id="@+id/adViewContainer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
android:layout_alignParentBottom="true"
/>
activity_splash_screen (Full code)
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:ads="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/splashx"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#D81B60"
tools:context="com.bigknol.SplashScreen">
<LinearLayout
android:id="@+id/mainContent"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginTop="172dp"
android:orientation="vertical"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<ImageView
android:id="@+id/logo"
android:layout_width="140dp"
android:layout_height="140dp"
android:src="@drawable/forest_bird"
android:layout_gravity="center_horizontal"
/>
<Space
android:layout_width="match_parent"
android:layout_height="20dp"/>
<TextView
android:id="@+id/app_title"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:padding="10dp"
android:textStyle="bold"
android:textAlignment="center"
android:textSize="20sp"
android:textColor="#FFFFFF"
android:text="Birds Sound Pro" />
<Space
android:layout_width="match_parent"
android:layout_height="5dp"/>
<TextView
android:id="@+id/app_des"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:padding="10dp"
android:textStyle="bold"
android:textAlignment="center"
android:textSize="16sp"
android:textColor="#F4B1B1"
android:text="Hear your favourite bird music" />
</LinearLayout>
<FrameLayout
android:id="@+id/adViewContainer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
android:layout_alignParentBottom="true"
/>
</RelativeLayout>
Image used in this project is given below :
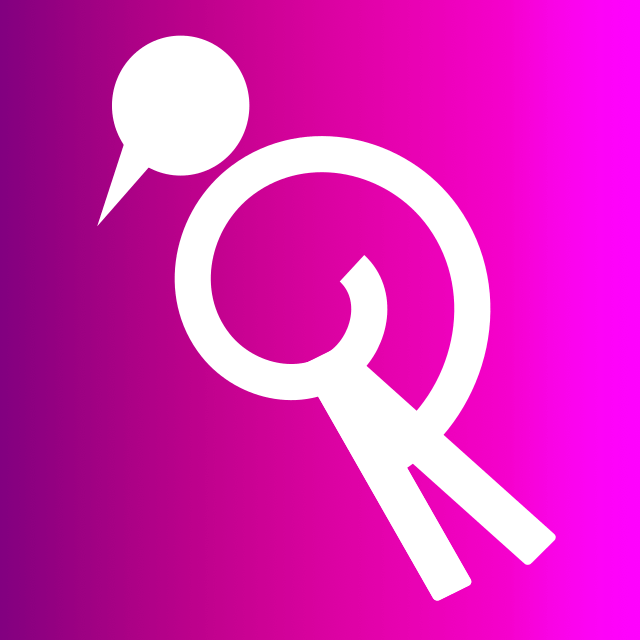
SplashScreen.java
private static final int SPLASH_SCREEN_TIME_OUT = 5000;
Let’s invoke the second activity from our splash screen.
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
Intent i = new Intent(SplashScreen.this, Rainwords.class);
startActivity(i); // invoke the Second Activity.
finish(); // the current activity will get finished.
}
}, SPLASH_SCREEN_TIME_OUT);
Get the device size
// get device size
private AdSize getAdSize() {
// Determine the screen width (less decorations) to use for the ad width.
Display display = getWindowManager().getDefaultDisplay();
DisplayMetrics outMetrics = new DisplayMetrics();
display.getMetrics(outMetrics);
float density = outMetrics.density;
float adWidthPixels = bannerContainerView.getWidth();
// If the ad hasn't been laid out, default to the full screen width.
if (adWidthPixels == 0) {
adWidthPixels = outMetrics.widthPixels;
}
int adWidth = (int) (adWidthPixels / density);
return AdSize.getCurrentOrientationAnchoredAdaptiveBannerAdSize(this, adWidth);
}
//ends
Load Banner
private void loadBanner() {
adView.setAdUnitId(BANNER_ID);
AdSize adSize = getAdSize();
adView.setAdSize(adSize);
AdRequest adRequest = new AdRequest.Builder().build();
// Start loading the ad in the background.
adView.loadAd(adRequest);
}
SplashScreen.java (Full code)
package com.bigknol;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.util.DisplayMetrics;
import android.util.Log;
import android.view.Display;
import android.view.ViewTreeObserver;
import android.view.Window;
import android.view.WindowManager;
import android.widget.FrameLayout;
import com.bigknol.rainwords.Rainwords;
import com.bigknol.slideimage.R;
import com.google.android.gms.ads.AdRequest;
import com.google.android.gms.ads.AdSize;
import com.google.android.gms.ads.AdView;
import com.google.android.gms.ads.MobileAds;
import com.google.android.gms.ads.RequestConfiguration;
import com.google.android.gms.ads.initialization.InitializationStatus;
import com.google.android.gms.ads.initialization.OnInitializationCompleteListener;
import java.util.Arrays;
public class SplashScreen extends AppCompatActivity {
private static final int SPLASH_SCREEN_TIME_OUT = 5000; // AdMob Recommended
private static final String BANNER_ID = "ca-app-pub-3940256099942544/6300978111";
private static final String TAG = "SplashScreen";
private FrameLayout bannerContainerView;
private boolean initialLayoutComplete = false;
private AdView adView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
getWindow().requestFeature(Window.FEATURE_NO_TITLE);
getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
setContentView(R.layout.activity_splash_screen);
// Log the Mobile Ads SDK version.
Log.d(TAG, "Google Mobile Ads SDK Version: " + MobileAds.getVersion());
// Initialize the Mobile Ads SDK.
MobileAds.initialize(this, new OnInitializationCompleteListener() {
@Override
public void onInitializationComplete(InitializationStatus initializationStatus) {}
});
// Test your Ad
MobileAds.setRequestConfiguration(
new RequestConfiguration.Builder().setTestDeviceIds(Arrays.asList("ABCDEF012345")).build());
// Testing ends
bannerContainerView = findViewById(R.id.adViewContainer);
adView = new AdView(this);
bannerContainerView.addView(adView);
bannerContainerView.getViewTreeObserver().addOnGlobalLayoutListener(new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
if(!initialLayoutComplete)
{
initialLayoutComplete = true;
loadBanner();
}
}
});
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
Intent i = new Intent(SplashScreen.this, Rainwords.class);
startActivity(i); // invoke the Second Activity.
finish(); // the current activity will get finished.
}
}, SPLASH_SCREEN_TIME_OUT);
}
// method for loading ad
private void loadBanner() {
adView.setAdUnitId(BANNER_ID);
AdSize adSize = getAdSize();
adView.setAdSize(adSize);
AdRequest adRequest = new AdRequest.Builder().build();
// Start loading the ad in the background.
adView.loadAd(adRequest);
}
// get device size
private AdSize getAdSize() {
// Determine the screen width (less decorations) to use for the ad width.
Display display = getWindowManager().getDefaultDisplay();
DisplayMetrics outMetrics = new DisplayMetrics();
display.getMetrics(outMetrics);
float density = outMetrics.density;
float adWidthPixels = bannerContainerView.getWidth();
// If the ad hasn't been laid out, default to the full screen width.
if (adWidthPixels == 0) {
adWidthPixels = outMetrics.widthPixels;
}
int adWidth = (int) (adWidthPixels / density);
return AdSize.getCurrentOrientationAnchoredAdaptiveBannerAdSize(this, adWidth);
}
//ends
/** Called before the activity is destroyed */
@Override
public void onDestroy() {
if (adView != null) {
adView.destroy();
}
super.onDestroy();
}
}
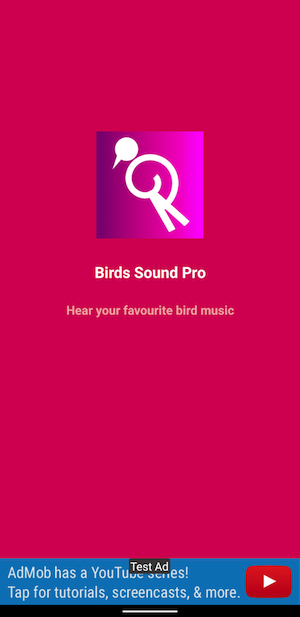
Congratulation! You are successfully implemented Splash Banner Ad using AdMob.
I have some questions!
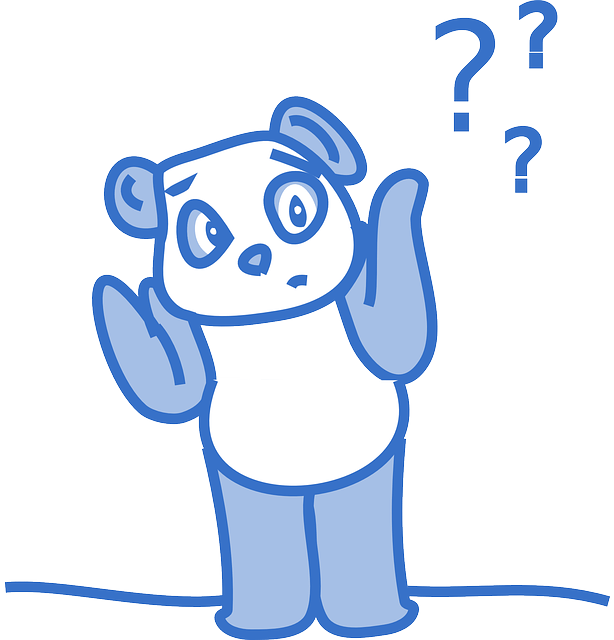
What is the difference between App Open and Splash Banner?
App Open occupies 85% of the screen, while Splash Banner should not exceed 40% of the screen.
Can I use an App Open Ad along with a Splash Banner Ad?
If you have App Open on a cold start, they should not transition to Splash Banner, as this could result in a decrease in revenue.
Does it support mediation?
If the specifications align with those of a normal or adaptive banner ad, third-party platforms should provide support.