Kotlin Coroutines : Learn from a Simple Example
Kotlin Coroutines are a powerful feature in the Kotlin programming language that enable you to write asynchronous code in a more straightforward and efficient manner. Whether you’re a seasoned developer or an absolute beginner, this tutorial will guide you through the basics of Kotlin Coroutines, helping you understand how they work and how to use them effectively.
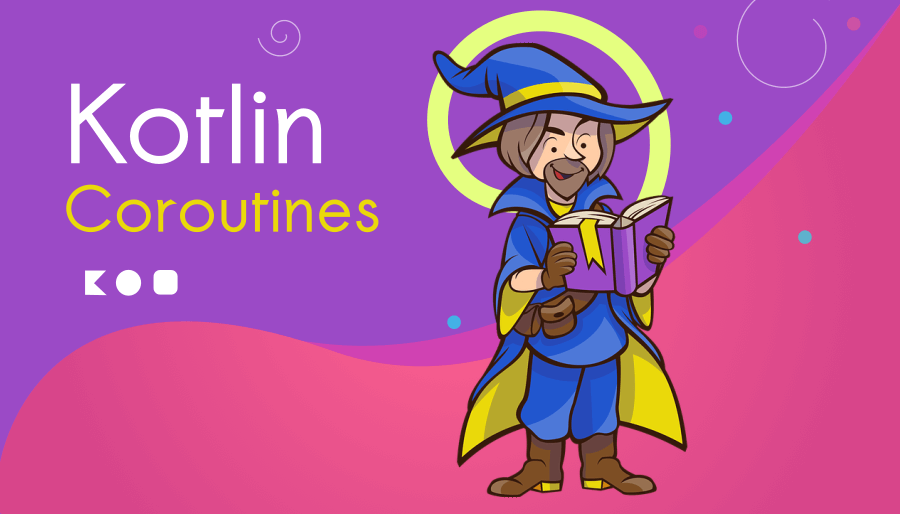
What are Kotlin Coroutines?
In essence, Kotlin Coroutines are a way to write asynchronous code that is both concise and readable. They allow you to perform tasks concurrently without blocking the main thread of your application. This is particularly useful for handling time-consuming operations, such as network requests or database queries, without freezing the user interface.
Do Kotlin Coroutines use Threads?
In concept, a Coroutine resembles a thread. In the sense that it concurrently executes a block of code alongside the rest of the program, a coroutine shares similarities with a thread. However, it’s important to note that a coroutine is not tied to any specific thread.
Coroutines may be likened to lightweight threads, yet several crucial distinctions exist, fundamentally altering their practical application compared to traditional threads.
Let’s write a simple example for a Kotlin Coroutine
It will be your first working coroutine:
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(3000L)
println("to Kotlin World!")
}
println("Welcome")
}
You will see the following result:
Output
Welcome
to Kotlin World!
Let’s break down the behaviour of this code.
import kotlinx.coroutines.*
This line imports the necessary classes and functions from the kotlinx.coroutines package, which is required to work with Kotlin Coroutines.
launch serves as a coroutine builder, initiating a new coroutine that runs concurrently with the remaining code, operating independently.
delay is a special suspending function. It suspends the coroutine for a specific time.
runBlocking another coroutine builder, serves as a bridge between the non-coroutine environment of a standard fun main()
and the code containing coroutines enclosed within runBlocking { ... }
curly braces. In an IDE, you’ll notice this through the CoroutineScope hint right after the opening curly brace of runBlocking.
If you omit or overlook the use of `runBlocking` in this code, an error will occur when calling `launch`, as `launch` is only defined within the `CoroutineScope` context.”
This demonstrates how Kotlin Coroutines can be used to perform asynchronous tasks concurrently without blocking the main thread.
Happy Coding!