Flutter SnackBar Material3 Example
Flutter SnackBar is a widget in the Flutter framework that displays a small message at the bottom of the screen, which appears temporarily and then disappears. It is used to give feedback to the user after they perform an action, such as after they submit a form, delete an item, or successfully complete a task.
The SnackBar widget typically contains a short message and an optional action button that allows the user to undo or perform another action related to the previous task. The SnackBar widget is highly customizable, and it allows developers to specify the duration of the message, the color of the background, the style of the text, and many other properties.
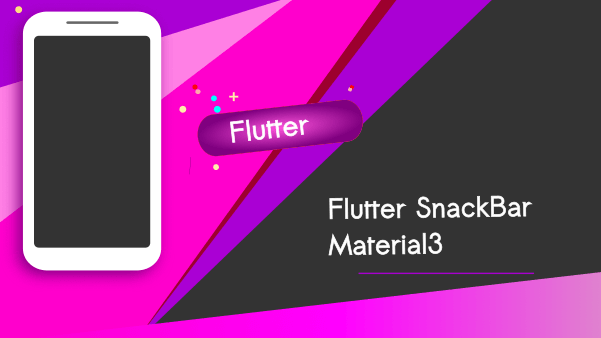
SnackBar is a part of the Material Design language, which is a design language developed by Google for Android, and it follows the guidelines of Material Design. However, it can also be used in non-Material apps.
main.dart
import 'package:flutter/material.dart';
import 'package:flutter/foundation.dart';
void main() {
runApp( const MyApp());
}
class MyApp extends StatelessWidget{
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return(
MaterialApp(
theme: ThemeData(
useMaterial3: true,
colorSchemeSeed: Colors.blue,
),
home: const MyAppScreen(),
)
);
}
}
class MyAppScreen extends StatelessWidget{
const MyAppScreen({super.key});
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
appBar: AppBar(
title: const Text("Simple SnackBar Demo"),
),
body: Center(
child: ElevatedButton(
onPressed: () {
final snackBar = SnackBar(
content: const Text('You did it.'),
action: SnackBarAction(
label: 'Cancel',
onPressed: () {
debugPrint('cancelled');
},
),
);
ScaffoldMessenger.of(context).showSnackBar(snackBar);
},
child: const Text('Tap to see SnackBar'),
),
)
);
}
}
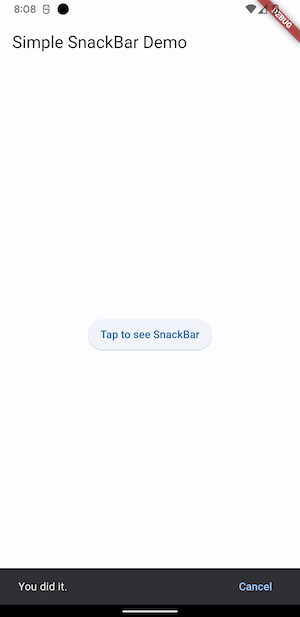
You can also find the official documentation of SnackBar from flutter.
Material 3
However, assuming that Material3 has been released since then, enabling it in a Flutter app would likely involve updating the app’s dependencies in the pubspec.yaml file to include the Material3 package, which may have a different name than the regular Material Design package.
After updating the dependencies, the app’s widgets would need to be updated to use the new Material3 widgets and components, which would likely have different names and properties than their Material Design counterparts. The specific changes needed would depend on the differences between Material Design and Material3.
It’s worth noting that Material3 is still under development and subject to change, so developers should keep an eye on updates and be prepared to make further changes as needed.