Control Flow in Dart If Else Statement
In Dart, an if-else statement is used to execute different code blocks depending on a condition.
Syntax
if (condition) {
// code to execute if the condition is true
} else {
// code to execute if the condition is false
}
The condition can be any expression that evaluates to a boolean value (true or false). If the condition is true, the code block inside the first set of curly braces will be executed. If the condition is false, the code block inside the second set of curly braces will be executed.
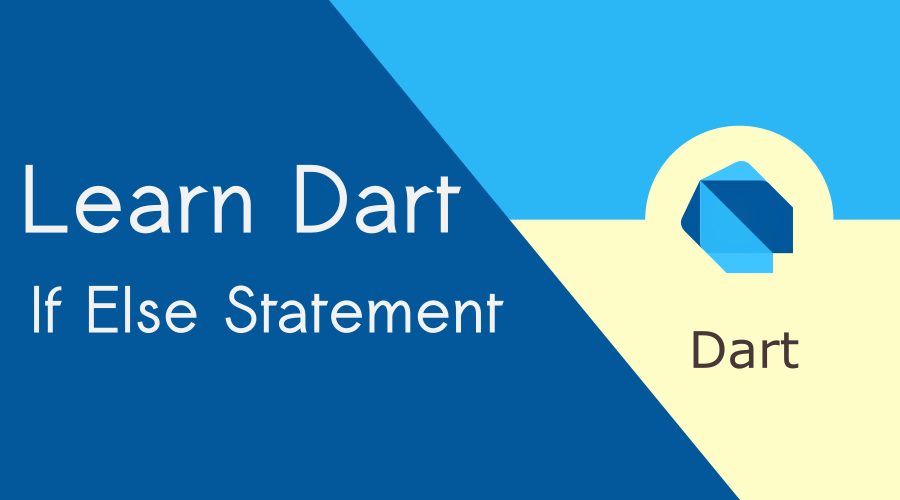
Dart If Else Example
void main()
{
int num = 41;
if(num%2==0)
{
print("Even number");
}
else {
print("Odd number");
}
}
int num = 41; assigns the value 41 to the integer variable num.
if(num%2==0) checks whether num is divisible by 2 (i.e., even) by using the modulo operator % to get the remainder of num divided by 2.
If the remainder is 0, then num is even.
If num is even, the code inside the curly braces {} will execute and print(“Even number”); will output “Even number”.
If num is odd, the code inside the else block will execute and print(“Odd number”); will output “Odd number”.
In this case, num is not divisible by 2 (i.e., num%2 is not equal to 0), so print(“Odd number”); will be executed and “Odd number” will be output.
You can also chain multiple if-else statements together to create more complex logic;
void main()
{
int x = 10;
if (x > 5) {
print("x is greater than 5");
} else if (x == 5) {
print("x is equal to 5");
} else {
print("x is less than 5");
}
}
In this example, the first condition is x > 5, which is true, so the first code block is executed. If the first condition had been false, the next condition (x == 5) would be checked, and so on.
Nano Assignment : Create a calculator using Dart If else statement
import 'dart:io';
void main(){
print("Enter first number: ");
double num1 = double.parse(stdin.readLineSync()!);
print("Enter second number");
double num2 = double.parse(stdin.readLineSync()!);
print("Enter an operation (+,-,*, /):");
String op = stdin.readLineSync()!;
double result;
if(op == '+')
{
result = num1 + num2;
} else if (op == '-'){
result = num1 - num2;
} else if(op == '*')
{
result = num1 * num2;
} else if(op == '/'){
if(num2 == 0)
{
print("Error: Cannot divide by zero");
return;
}
result = num1 / num2;
} else {
print("Error : Invalid operation");
return;
}
print("Result : $result");
}
The program prompts the user to enter two numbers and an operation (+, -, *, /). It then performs the operation on the two numbers and prints the result. If the user enters an invalid operation or tries to divide by zero, an error message is printed instead of the result.
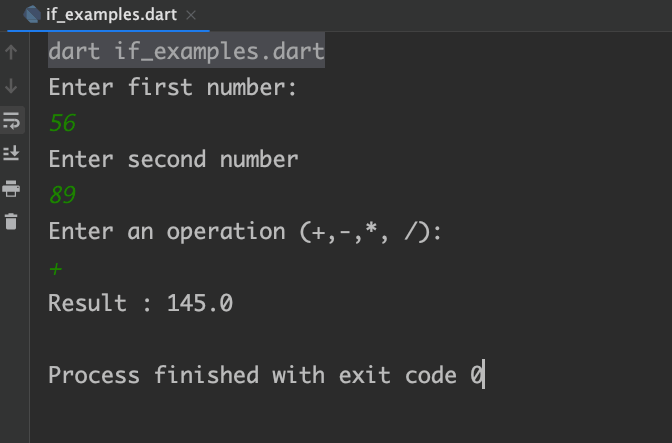