Maps in Kotlin : Let’s Store Items as Key Value Pairs
Maps in Kotlin are useful for storing items as key-value pairs. You can access the value by referencing the key. In a Kotlin map, each key must be unique to enable Kotlin to identify the corresponding value you wish to retrieve. However, duplicate values are permissible within a map.
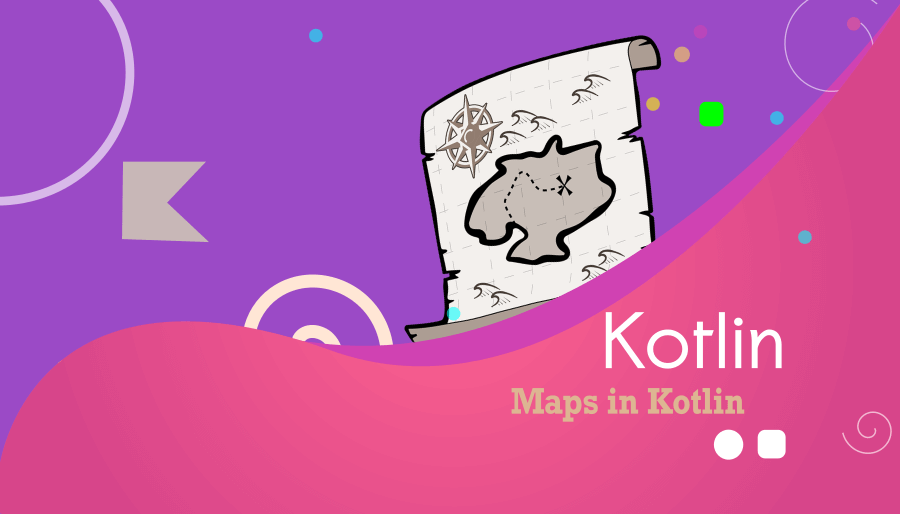
Create a read-only map in Kotlin
val books = mapOf("Deep Work" to 10, "Wonder" to 18, "Atomic Habits" to 15)
How to get the value of Book ‘Wonder’?
println("Price of Wonder : ${books["Wonder"]} $")
To get number of items in a map:
println(books.count())
Explicit type declaration (Map)
val day:Map<Int, String> = mapOf(
1 to "Sunday", 2 to "Monday",
3 to "Tuesday")
Program Example :
fun main() {
val books = mapOf("Deep Work" to 10, "Wonder" to 18, "Atomic Habits" to 15)
books.forEach{
(key, value) ->
println("$key : Price: $${value}")
}
//explicit type declaration
val day:Map<Int, String> = mapOf(
1 to "Sunday", 2 to "Monday",
3 to "Tuesday")
day.forEach{
(keys,values) ->
println("$keys : $values")
}
}
Output
Deep Work : Price: $10
Wonder : Price: $18
Atomic Habits : Price: $15
1 : Sunday
2 : Monday
3 : Tuesday
MutableMap in Kotlin
MutableMap represents a modifiable map; it is an extension of the Map interface and provides additional functions to modify the contents of the map, such as adding, removing, or updating key-value pairs.
val mutableMap: MutableMap<String, Double> = mutableMapOf(
"area" to 67.00,
"place" to 789.07,
)
Adding a new key-value pair
mutableMap["extra"] = 890.878
Updating the value for an existing key
mutableMap["area"] = 89.00
Delete all items
clear() removes all items from the map, and remove(key) deletes the item associated with the specified key.
mutableMap.clear()
Delete a specific item by key (MutableMap)
val keyToDelete = "place"
mutableMap.remove(keyToDelete)
Using replace()
The replace() function in Kotlin is used to replace the value associated with a specified key in a MutableMap
val keyToReplace = "place"
val newValue = 8009.00
mutableMap.replace(keyToReplace, newValue)
Kotlin Data Class Objects to a MutableMap
To convert a Kotlin data class object to a MutableMap, you can use an extension function provided by the Kotlin standard library.
Data class
data class Album(val name:String, val year: Int)
Let’s create an extension function ‘toMutableMap()’ for Album data class.
fun Album.toMutableMap(): MutableMap<String, Any> {
return mutableMapOf("name" to name, "year" to year)
}
Program Example:
Open the Kotlin Playground and write the following code:
data class Album(val name:String, val year: Int)
fun Album.toMutableMap(): MutableMap<String, Any> {
return mutableMapOf("name" to name, "year" to year)
}
fun main() {
// Create an instance of the data class
val album = Album("Midnights", 2022)
// Converting the data class object to a MutableMap
val albumMap:MutableMap<String, Any> = album.toMutableMap()
// print the result
println("Album Name: ${albumMap["name"]} \n Year: ${albumMap["year"]}")
}
Output:
Album Name: Midnights
Year: 2022
Maps in Kotlin : HashMap vs MutableMap
HashMap
HashMap is a specific implementation of the MutableMap interface, offering a mutable, unordered collection of key-value pairs. You can add, remove, and update elements after the map is created. The Java Collection Framework includes HashMap, and Kotlin offers interoperability with Java Collections.
val hashMap: HashMap<String, Int> = hashMapOf("one" to 1, "two" to 2, "three" to 3)
MutableMap
MutableMap is an interface in Kotlin that represents a generic mutable map. It defines common operations for mutable maps, such as adding, removing, and updating key-value pairs.
In conclusion, MutableMap is an interface representing a generic mutable map, while HashMap is a specific implementation of MutableMap that provides unordered key-value pairs.
Happy Coding!