Operators in Kotlin : Let’s Practice with Examples
Kotlin, a modern programming language, provides a rich set of operators that enable developers to perform various operations on variables and objects. Operators in Kotlin go beyond the conventional arithmetic and logical operations, empowering programmers to write concise and expressive code.
We will explore the different types of operators available in the language, including arithmetic, assignment, comparison, bitwise, and logical operators.
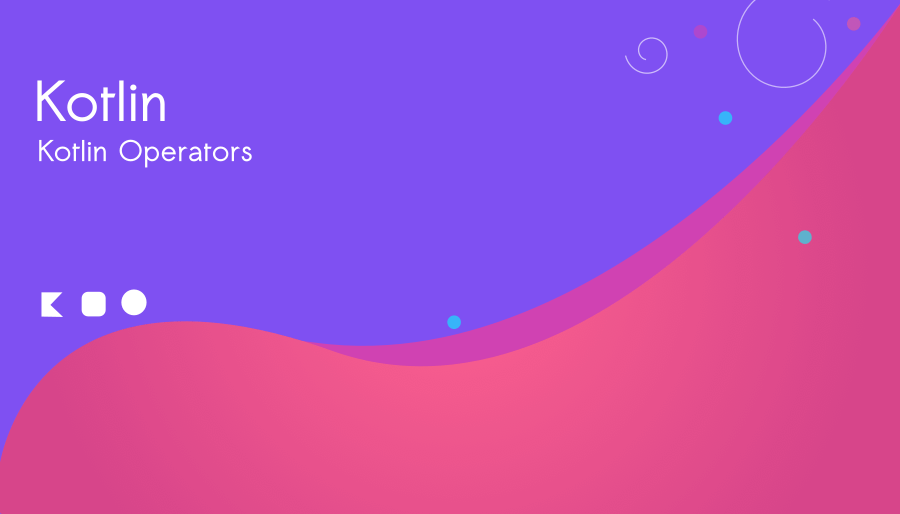
Arithmetic Operators
Arithmetic operators in Kotlin allow you to perform basic mathematical calculations. The standard arithmetic operators include addition (+), subtraction (-), multiplication (), division (/), and modulo (%). Kotlin also introduces the exponentiation operator (*), which raises a number to a specified power.
Program Example for All Arithmetic Operators
Open Kotlin Playground and Write the following program.
import kotlin.math.pow
fun main() {
val a = 20
val b = 10
val addition = a + b
println("Addition: $addition")
val subtraction = a - b
println("Subtraction: $subtraction")
val multiplication = a * b
println("Multiplication: $multiplication")
val division = a / b
println("Division: $division")
val modulo = a % b
println("Modulo: $modulo")
val exponentiation = a.toDouble().pow(b.toDouble())
println("Exponentiation: $exponentiation")
}
Output
Addition: 30
Subtraction: 10
Multiplication: 200
Division: 2
Modulo: 0
Exponentiation: 1.024E13
However, if you wish to use the pow() function from the kotlin.math package, you will need to import it explicitly.
Assignment Operators in Kotlin
Assignment operators in Kotlin provide a shorthand way to assign values to variables while performing an operation. These operators combine an arithmetic operator with the assignment operator (=). For example, += performs addition and assignment in a single step.
Program Example for Assignment operators
fun main() {
var a = 30
var b = 15
println("Initial values: a = $a, b = $b")
a += b
println("After a += b: a = $a")
a -= b
println("After a -= b: a = $a")
a *= b
println("After a *= b: a = $a")
a /= b
println("After a /= b: a = $a")
a %= b
println("After a %= b: a = $a")
}
Output
Initial values: a = 30, b = 15
After a += b: a = 45
After a -= b: a = 30
After a *= b: a = 450
After a /= b: a = 30
After a %= b: a = 0
Comparison Operators in Kotlin
Comparison operators in Kotlin allow you to compare values and determine relationships between them. These operators include equality (==), inequality (!=), greater than (>), less than (<), greater than or equal to (>=), and less than or equal to (<=).
fun main() {
val a = 10
val b = 5
val isEqual = a == b
println("Is a equal to b? $isEqual")
val isNotEqual = a != b
println("Is a not equal to b? $isNotEqual")
val isGreater = a > b
println("Is a greater than b? $isGreater")
val isLess = a < b
println("Is a less than b? $isLess")
val isGreaterOrEqual = a >= b
println("Is a greater than or equal to b? $isGreaterOrEqual")
val isLessOrEqual = a <= b
println("Is a less than or equal to b? $isLessOrEqual")
}
Is a equal to b? false
Is a not equal to b? true
Is a greater than b? true
Is a less than b? false
Is a greater than or equal to b? true
Is a less than or equal to b? false
The boolean result of each comparison is stored in a variable and then printed using string interpolation.
Bitwise Operators in Kotlin
Bitwise operators in Kotlin are used to manipulate individual bits within numeric values. These operators perform operations at the binary level, enabling developers to perform tasks such as bit shifting, bitwise AND (&), bitwise OR (|), bitwise XOR (^), and bitwise complement (unary ~).
Program Example for All Arithmetic Operators
fun main() {
val a = 0b1100
val b = 0b1010
val bitwiseAnd = a and b
println("Bitwise AND: ${bitwiseAnd.toString(2)}")
val bitwiseOr = a or b
println("Bitwise OR: ${bitwiseOr.toString(2)}")
val bitwiseXor = a xor b
println("Bitwise XOR: ${bitwiseXor.toString(2)}")
val bitwiseComplementA = a.inv()
println("Bitwise complement of a: ${bitwiseComplementA.toString(2)}")
val leftShift = a shl 2
println("Left shift of a by 2: ${leftShift.toString(2)}")
val rightShift = a shr 2
println("Right shift of a by 2: ${rightShift.toString(2)}")
val zeroFillRightShift = a ushr 2
println("Zero-fill right shift of a by 2: ${zeroFillRightShift.toString(2)}")
}
Bitwise AND: 1000
Bitwise OR: 1110
Bitwise XOR: 110
Bitwise complement of a: -1101
Left shift of a by 2: 110000
Right shift of a by 2: 11
Zero-fill right shift of a by 2: 11
- and operator: Performs a bitwise AND operation on a and b.
- or operator: Performs a bitwise OR operation on a and b.
- xor operator: Performs a bitwise XOR (exclusive OR) operation on a and b.
- inv() function: Performs a bitwise complement (NOT) operation on a.
- shl operator: Performs a left shift operation on a by the specified number of bits.
- shr operator: Performs a right shift operation on a by the specified number of bits.
- ushr operator: Performs a zero-fill right shift operation on a by the specified number of bits.
This example demonstrates how bitwise operators can be used to perform operations at the binary level, manipulating individual bits within numeric values.
Logical Operators in Kotlin
Logical operators in Kotlin allow you to perform logical operations on Boolean expressions. These operators include logical AND (&&), logical OR (||), and logical NOT (!).
fun main() {
val a = true
val b = false
val logicalAnd = a && b
println("Logical AND: $logicalAnd") // false
val logicalOr = a || b
println("Logical OR: $logicalOr") // true
val logicalNotA = !a
println("Logical NOT of a: $logicalNotA") //false
val logicalNotB = !b
println("Logical NOT of b: $logicalNotB") // true
}
Output
Logical AND: false
Logical OR: true
Logical NOT of a: false
Logical NOT of b: true
In this example, we have two variables a and b assigned boolean values true and false, respectively.
- && (logical AND) operator: Evaluates to true if both a and b are true, otherwise false.
- || (logical OR) operator: Evaluates to true if at least one of a or b is true, otherwise false.
- ! (logical NOT) operator: Negates the boolean value of a or b.
The results of each logical operation are stored in variables and printed using string interpolation.