Flutter navigation : Push and Pop for routes
It’s good to have proper navigation for your application. Flutter pages or screens tend to be moved around according to user preference. Thanks to Flutter Navigator.
In flutter philosophy, every page or screen is called a route. It is similar to Activity in Android Development.
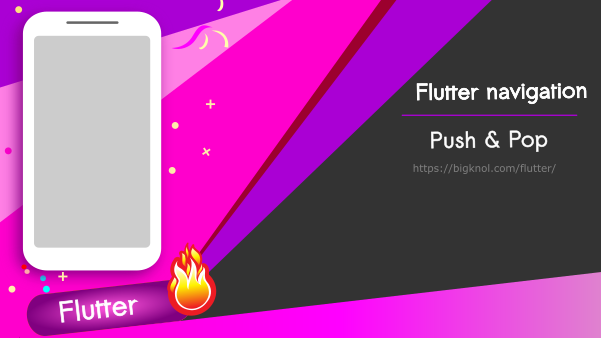
How to navigate to next screen ?
Navigator.push(context, MaterialPageRoute(builder: (context) => const DetailsRoute()));
Here DetailsRoute is another screen.
In flutter, a route is just a widget. If you want to switch to a new ‘route’ you can use Navigator. push() method. The push() method adds Route to the stack of routes operated by the Navigator.
What is ‘Route’?
You can create your own Route or use the default MaterialPageRoute to manage the transition between routes which is helpful for platform-specific animation. (you could see flawless animation on iOS and Android platforms)
How to return to the First Screen (route)?
Navigator.pop(context);
Thanks to the pop(). This method closes the second ‘route’ (screen or page) and returns to the first. The pop() method removes the current Route from a stack of routes.
Let’s create a new project called Flutter Navigation Demo.
Let’s create a new package (or directory) called nav.
Create a file home.dart (nav/home.dart)
import 'package:flutter/material.dart';
import 'package:flutternavigationdemo/nav/details.dart';
void main()
{
runApp(const MaterialApp(
home: HomeRoute(),
title: 'Home Page',
));
}
class HomeRoute extends StatelessWidget
{
const HomeRoute({Key? key}) : super(key :key);
@override
Widget build(BuildContext context) {
return
Scaffold(
appBar: AppBar(title: const Text("Home"),
),
body: Center(
child: Column(
children: [
Image.network("https://cdn.pixabay.com/photo/2021/12/27/14/04/paper-rocket-6897262_960_720.png", height: 150,width: 150, ),
ElevatedButton(onPressed: (){
Navigator.push(context, MaterialPageRoute(builder: (context) => const DetailsRoute()));
}, child: const Text("Show"))
],
),
),
);
}
}
Details.dart
import 'package:flutter/material.dart';
import 'home.dart';
void main()
{
runApp(const MaterialApp(home: DetailsRoute(), title: 'Details',));
}
class DetailsRoute extends StatelessWidget
{
const DetailsRoute({Key? key}) : super(key :key);
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
appBar: AppBar(title: const Text("Details"),
),
body: Center(
child: Column(
children: [
Image.network("https://cdn.pixabay.com/photo/2017/03/22/03/14/emoji-2163841_960_720.png", height: 150,width: 150, ),
ElevatedButton(onPressed: (){
Navigator.pop(context);
}, child: const Text("Back"))
],
),
),
);
}
}
How does this app look like ?
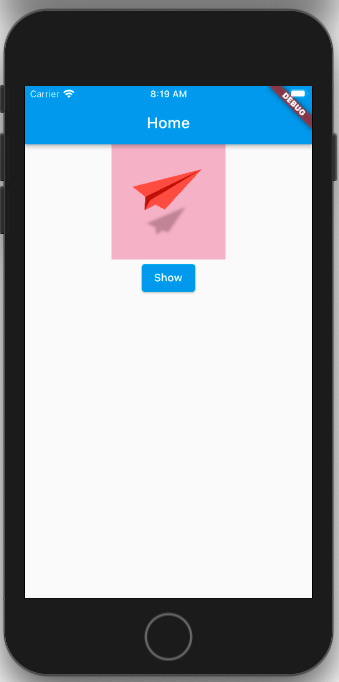
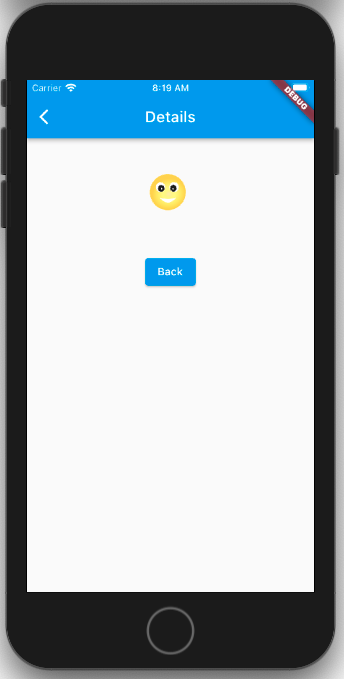