Android Jetpack Compose Hello World
Android Jetpack Compose is a modern toolkit for building amazing native apps for Android devices. It does come with intuitive Kotlin APIs. A few lines of code can make a beautiful UI without old school boilerplate codes. Android Jetpack Compose offers built-in support for Material Design, Animations, Themes, and more.
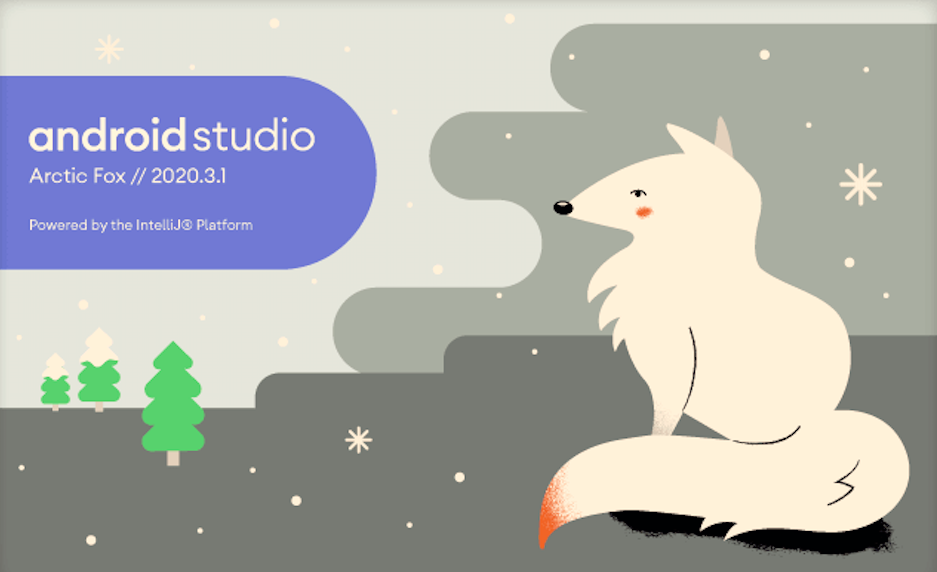
To create a project in an Android studio is super easy. Open the IDE and create a new project. Choose Empty Compose Activity.

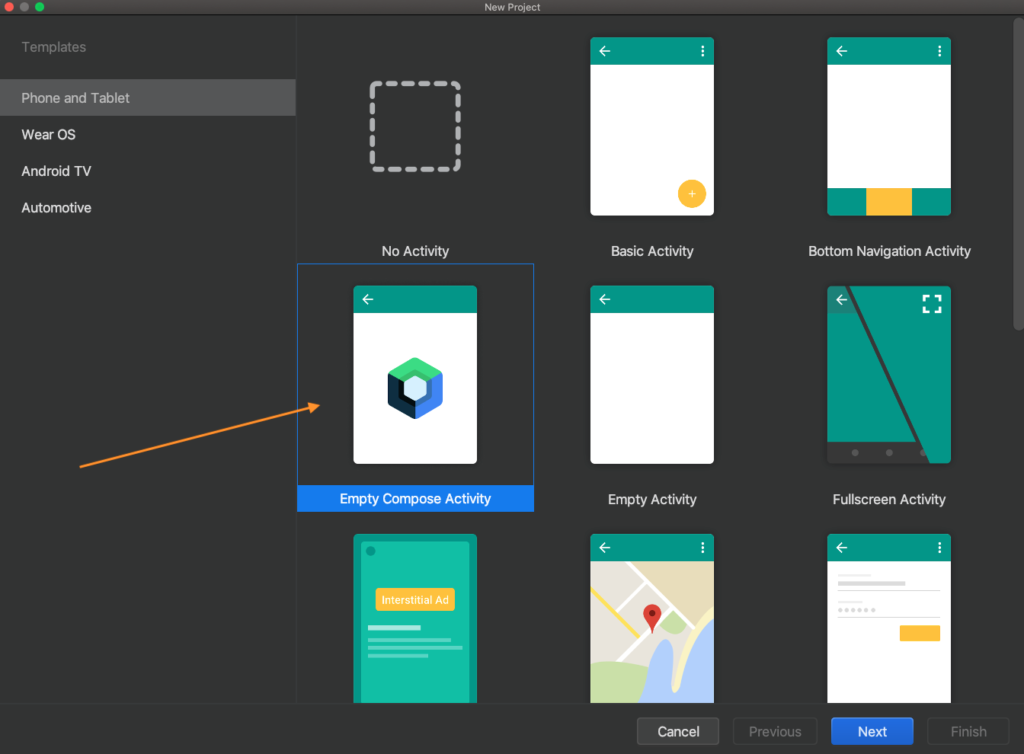
Make sure that you have given the name of the project and location. Unfortunately, the Tool Kit does not support Java Programming language. So you should choose Kotlin as your primary language. Finally hit the Finish button.
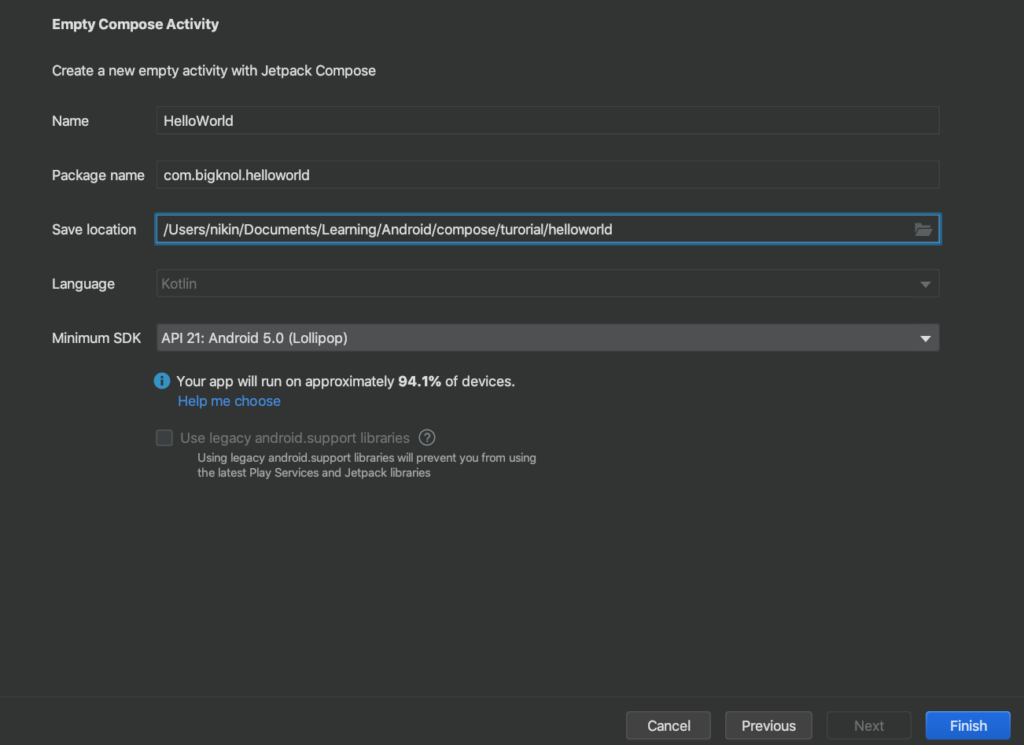
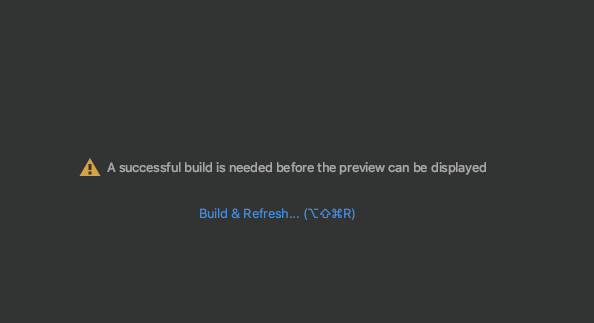
You might have encountered this kind of error message at the beginning. Don’t panic! Just click Build & Refresh link
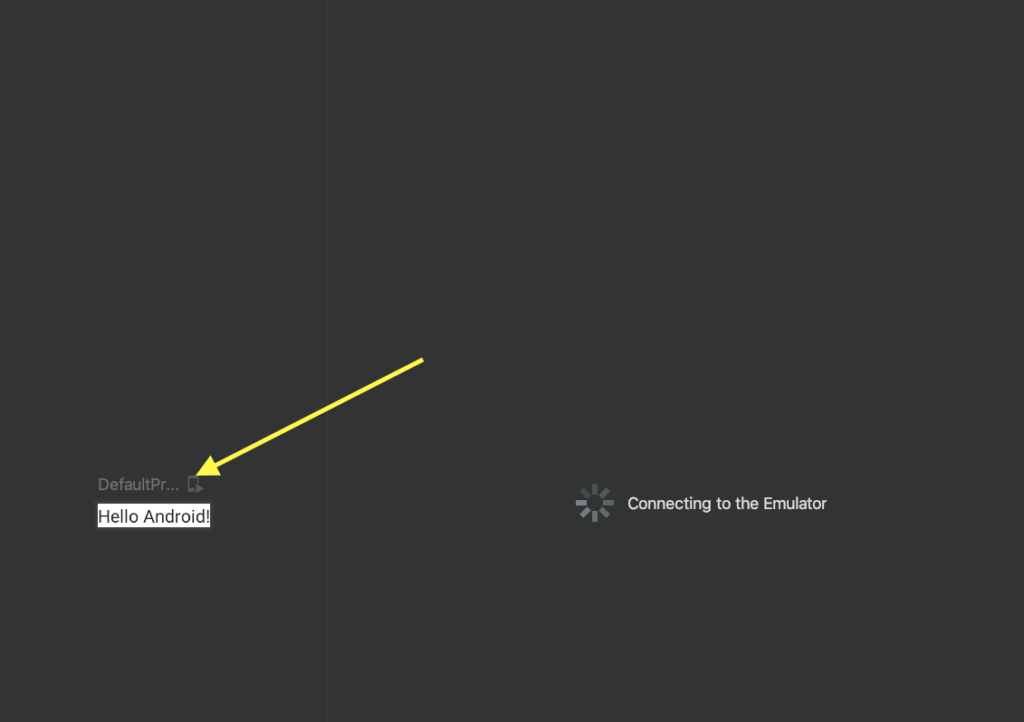
Next step: Click on the Run button. It will connect to the Emulator. If everything goes well, you can see a label saying “Hello World”.
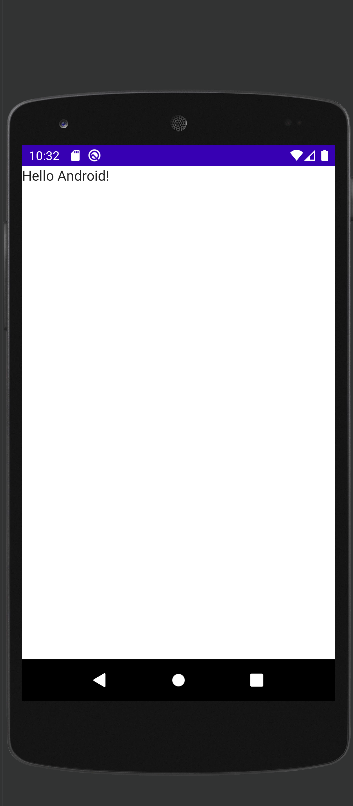
If you observe the MainActivity.kt file, you can notice @Composable annotations. Jetpack Compose is built roughly composable functions. These functions are efficient and let you define your app’s user interface programmatically by describing how it should look.
MainActivity.kt
package com.bigknol.helloworld
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.material.MaterialTheme
import androidx.compose.material.Surface
import androidx.compose.material.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.tooling.preview.Preview
import com.bigknol.helloworld.ui.theme.HelloWorldTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
HelloWorldTheme {
// A surface container using the 'background' color from the theme
Surface(color = MaterialTheme.colors.background) {
Greeting("World")
}
}
}
}
}
@Composable
fun Greeting(name: String) {
Text(text = "Hello $name!")
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
HelloWorldTheme {
Greeting("World")
}
}
Let’s add our own composable function
@Composable
fun SayHello()
{
Text("I like Compose ")
}
Make changes in DefaultPreview()
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
HelloWorldTheme {
SayHello()
}
}
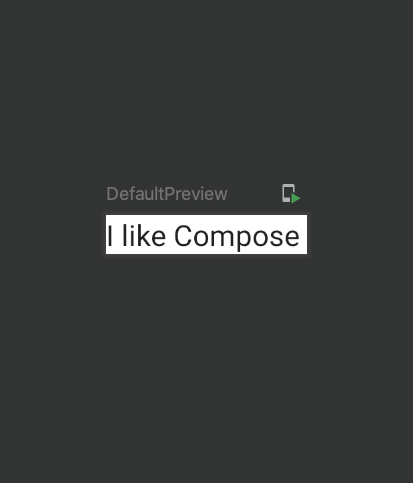
If you run the app you will not see the actual text. We need to make changes in setContent
setContent {
HelloWorldTheme {
// A surface container using the 'background' color from the theme
Surface(color = MaterialTheme.colors.background) {
SayHello()
}
}
}
Calculation
Create a composable function for the addition of two numbers.
@Composable
fun addNumbers(a: Int, b: Int)
{
Text("Sum of $a and $b : ${a+b}")
}
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
HelloWorldTheme {
// A surface container using the 'background' color from the theme
Surface(color = MaterialTheme.colors.background) {
addNumbers(a = 10, b = 20 )
}
}
}
}
}
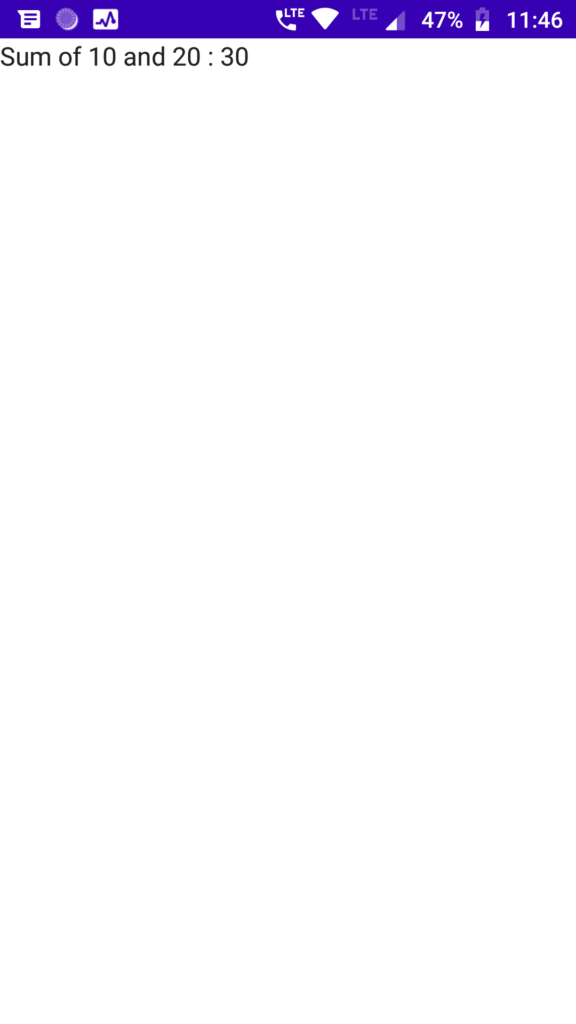