Text in Jetpack Compose : Styling, Alignment and Overflow
Creating text in Jetpack Compose is a super easy task. Text() is a composable element that can display text in our app. Text is a fundamental element in app design and serves a critical role in communication and user experience. Textual content conveys information, instructions, and messages to app users.
It serves as the primary means of communication between the app and the user, helping users understand, navigate, and interact with the app’s features. Clarity and usability: Well-crafted text ensures clarity and usability in the app. It provides context, explains functionality, and guides users through the app’s interface.
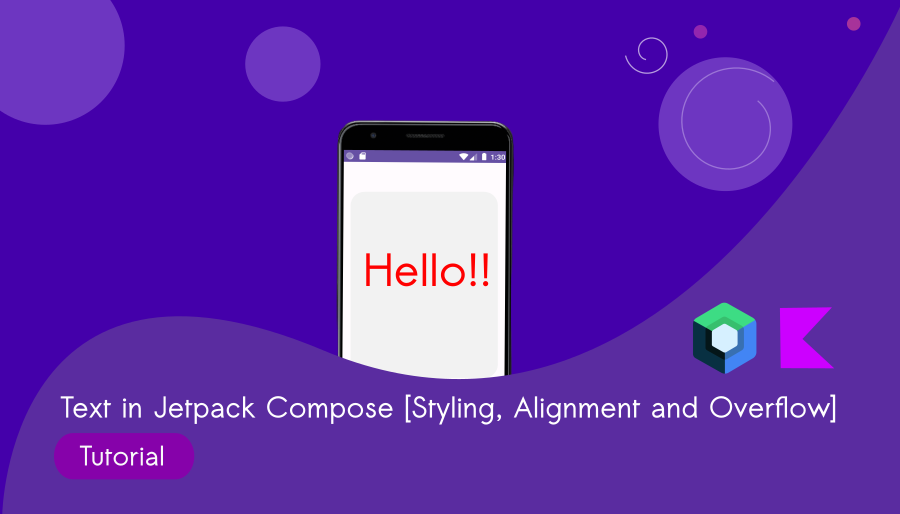
Clear and concise text enhances the user’s ability to achieve their goals within the app. Sounds good? Let’s give it a try.
Text("Simple Text Example : Jetpack Compose!")
Create an Android Studio Jetpack Compose project and write a composable function as below:
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!")
}
MainActivity.kt
package com.bigknol.todolistapp
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.bigknol.todolistapp.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
TextExample()
}
}
}
}
}
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!")
}
@Preview(showBackground = true, showSystemUi = true,)
@Composable
fun GreetingPreview() {
ExampleTheme {
TextExample()
}
}
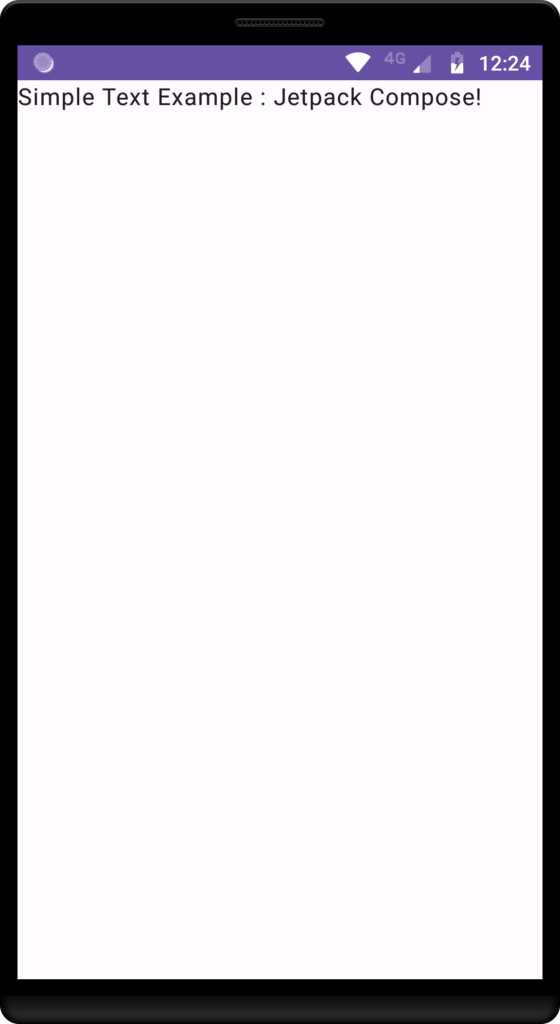
Change Color of Text in Jetpack Compose
Text Styling in Compose
Easily style your text by using the ‘style‘ parameter. You can modify text appearance with properties like fontSize, fontWeight, color, textAlign, and TextDecoration.
Let’s change color of our text to red.
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!", style = TextStyle(color = Color.Red))
}
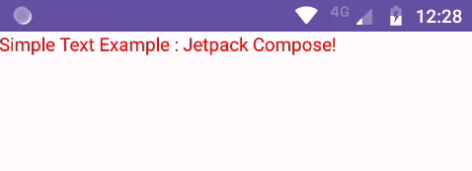
Text Size and Bold Text
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!", style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold, fontSize = 25.sp))
}
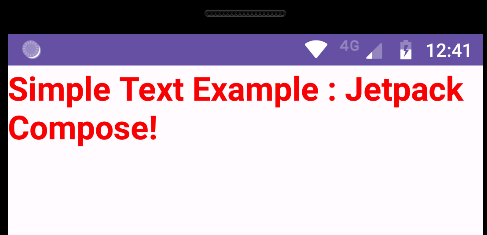
Compose’s Strikethrough Text:
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!", style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 25.sp, textDecoration = TextDecoration.LineThrough ))
}
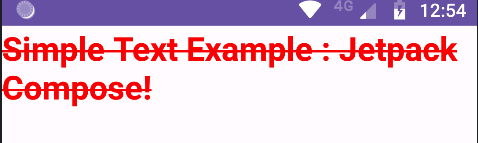
Underline text in jetpack compose
Sometimes, we want to underline our text, so we use the textDecoration parameter and set it to TextDecoration.Underline.
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!", style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 25.sp,
textDecoration = TextDecoration.Underline ))
}
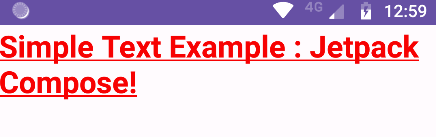
Text Alignment in Compose
We can align text with ‘textAlign‘ property. Options include ‘Start’, ‘End’, ‘Center’ and Justify.
Text("Simple Text Example : Jetpack Compose!",
textAlign = TextAlign.Center)
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!",
textAlign = TextAlign.Center,
style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 15.sp,))
}
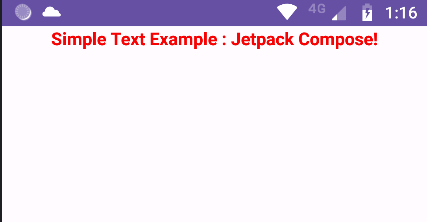
Text Alignment (Start)
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!",
textAlign = TextAlign.Start,
style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 15.sp,))
}
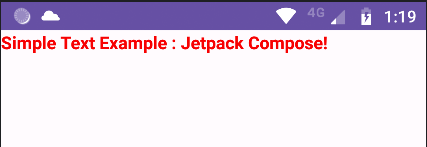
Text Alignment (End)
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!",
textAlign = TextAlign.End,
style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 15.sp,))
}
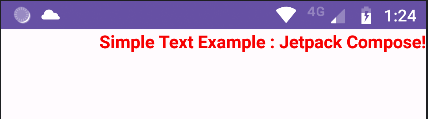
Text Alignment (Justify)
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose!",
textAlign = TextAlign.Justify,
style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 15.sp,))
}
Compose Text Max lines and Overflow
Handle text overflow with the overflow property. Common values are Ellipsis and Clip. For instance:
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose! I am a long text Swagatham!",
maxLines =1, overflow = TextOverflow.Ellipsis,
style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 15.sp,))
}
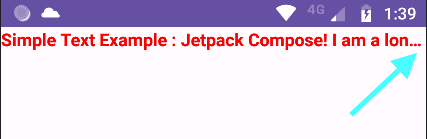
Text Multiline
To display multiline text, set maxLines to control the number of visible lines:
@Composable
fun TextExample()
{
Text("Simple Text Example : Jetpack Compose! I am a long text Swagatham!",
maxLines =3, overflow = TextOverflow.Ellipsis,
style = TextStyle(color = Color.Red,
fontWeight = FontWeight.Bold,
fontSize = 15.sp,))
}
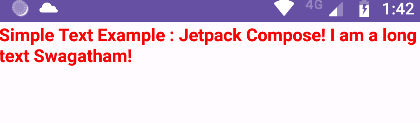
Compose offers versatile tools to work with text in Android app development. By following this tutorial, you can create stylish and interactive text elements to enhance your app’s user experience. Experiment with these features and elevate your app’s text presentation.