Interface Inheritance in Kotlin (with Example)
Kotlin has many features that boost up your development speed in a tremendous way. One of such features is interface inheritance in Kotlin
In this Kotlin tutorial, we will explore interface inheritance in Kotlin with a fruitful example
An interface can inherit from other interfaces, allowing it to include implementations for their parts and introduce new functions and properties. When a class implements this interface, it only needs to define the parts(implementations) that are missing.
interface Person{
val name:String
}
interface: This keyword is used to define an interface in Kotlin.
‘Person’ :This is the name of the interface. In Kotlin, interfaces follow the same naming conventions as classes, usually using PascalCase.
val name: String: This is a property declaration within the Person interface. The val keyword denotes that this is a read-only property (similar to a constant) that must be implemented by classes that implement the Person interface. The name property is of type String.
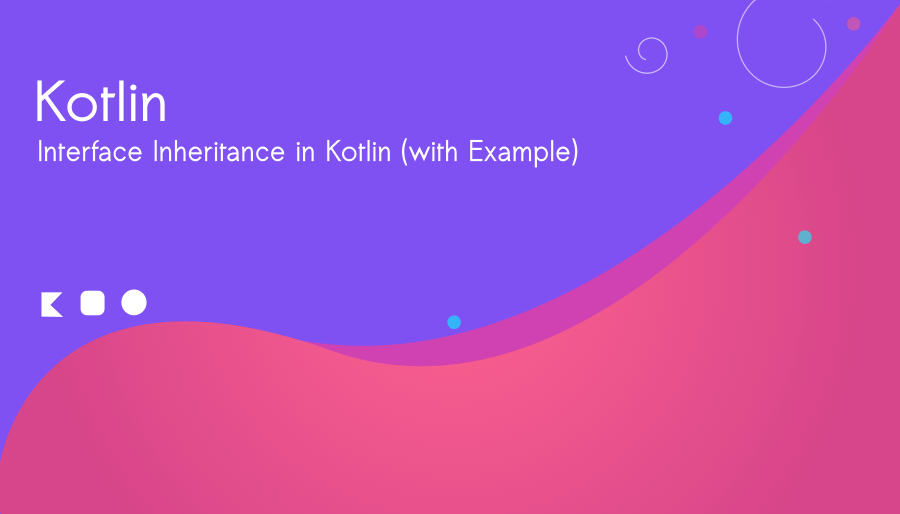
Applying Inheritance
interface User: Person{
val userId: String
val userName: String
override val name: String
get() = "UserName: $userName and ID: $userId"
}
The User interface introduces three properties: userId, userName, and an overriding property name.
The User interface is defined with the interface keyword and extends the Person interface.
This means that any class implementing the User interface must also provide implementations for all properties defined in both User and Person interfaces.
override val name: String: This is an overriding property. The name property is declared in the User interface and is marked with the override keyword, indicating that it overrides the name property from the Person interface.
get() = “UserName: $userName and ID: $userId”: This is the getter implementation for the name property. When a class implementing the User interface accesses the name property, this getter will be called.
The getter returns a formatted string that includes both the userName and userId properties. The value of the name property is dynamically generated based on the values of userName and userId.
In data class, we don’t need to implement the ‘name’ property of interface ‘Person’
data class Info(
// no need to implement the 'name'
override val userId: String,
override val userName: String,
val id:Int = 0
): User
Interface Inheritance Example
Complete code:
Open Kotlin Playground in your browser and write the following code:
interface Person{
val name:String
}
interface User: Person{
val userId: String
val userName: String
override val name: String
get() = "UserName: $userName and ID: $userId"
}
data class Info(
// no need to implement the 'name'
override val userId: String,
override val userName: String,
val id:Int = 0
): User
fun main()
{
val user = Info(userId = "0067", userName = "Nicky")
println(user.name)
}
Output
UserName: Nicky and ID: 0067
By using interfaces and inheritance, this code promotes code reusability and allows you to define common behavior and properties in the Person interface while adding specific properties and behavior in the User interface.